Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial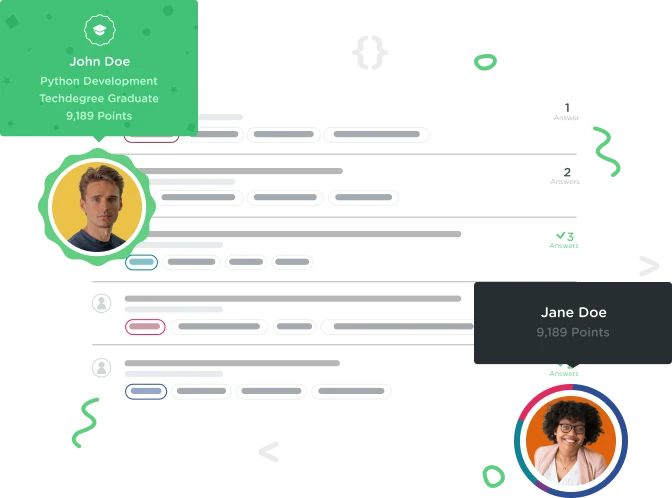
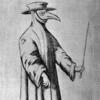
Erik Biebinger
7,299 PointsHow does the code "know" which question to ask? (Improve the Quiz Solution [JavaScript Arrays])
Hey :)
I was wondering how the JavaScript code "knows" which question to ask?
This is the solution code:
// 1. Create a multidimensional array to hold quiz questions and answers const questions = [ ['How many planets are in the Solar System?', '8'], ['How many continents are there?', '7'], ['How many legs does an insect have?', '6'], ['What year was JavaScript created?', '1995'] ];
// 2. Store the number of questions answered correctly const correct = []; const incorrect = []; let correctAnswers = 0;
/*
- Use a loop to cycle through each question
- Present each question to the user
- Compare the user's response to answer in the array
- If the response matches the answer, the number of correctly answered questions increments by 1 */ for ( let i = 0; i < questions.length; i++ ) { let question = questions[i][0]; let answer = questions[i][1]; let response = prompt(question);
if ( response === answer ) { correctAnswers++; correct.push(question); } else { incorrect.push(question); } }
I was wondering why JavaScript isn't asking the first question 4 times since, the loop runs for 4 times due to the length of the first array. How does JavaScript "know" to ask the 2nd and 3rd question as well as the 4th?
I hope you understand my question and can help me get my head around this.
Thanks in advance :) Cheers Erik
1 Answer

Rohald van Merode
Treehouse StaffHi Erik Biebinger,
Great question! JavaScript knows because you're looping over each of the questions and selecting them one at a time. What is happening in your loop is that the initial value of i
is set to 0
. The first time your loop runs the first line in the loop is set to let question = questions[0][0];
and the user is prompted to answer the first question.
After the user has answered that question the loop will go through the next iteration, i
will be increased to 1
and therefor the next line will now be let question = questions[1][0];
. Selecting the next question / answer in the questions
array.
The loop will repeat this process increasing i
by 1 on every iteration until the last question has been asked (until the conditional in the loop i < questions.length;
is no longer true.
Something that helped me tremendously during my learning journey was to log a different values to the console. This will help you to see what your code is doing and gives you a better grasp on the syntax and logic of your code. In this example I would suggest to add some console.log
calls in your code and play around with some different values. For example in the loop you could try to console.log(i)
or console.log(questions[i])
and console.log(questions[i][0]
.
I hope this has been helpful 🙂
Erik Biebinger
7,299 PointsErik Biebinger
7,299 PointsAaaaah, riiight! Now I get it :) This makes more sense now, thanks a lot!