Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial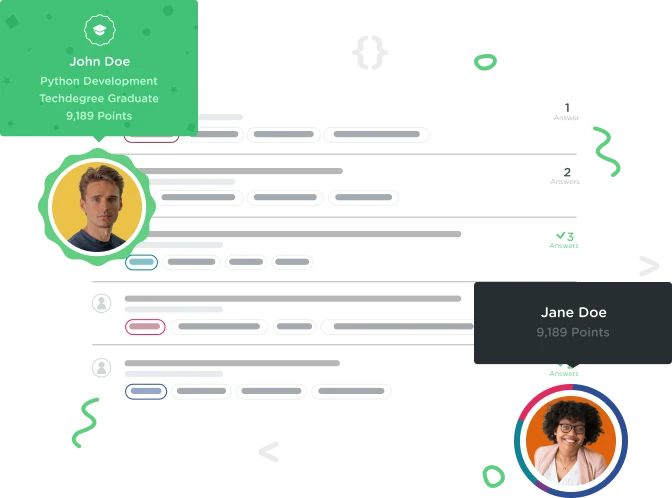

Daniel Hunter
4,622 Pointshow do you use .indexOf in an if statement to see if the last name initial is a letter from 'a' to 'm' or 'n' to 'z'?
please help, I have tried many different ways and am struggling to come up with the answer. I have only got one compiler error left
import java.util.Scanner;
public class ConferenceRegistrationAssistant {
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
char[] charArray = "abcdefghijklm".toCharArray();
char[] charArray2 = "nopqrstuvwxyz".toCharArray();
Scanner scanner = new Scanner(System.in);
System.out.print("Enter Last Name: ");
String nameInput = scanner.nextLine();
char initial = nameInput.charAt(0);
if (initial.indexOf(charArray) != -1){
lineNumber +=1;
} else {
lineNumber +=2;
}
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
3 Answers
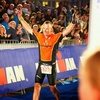
Steve Hunter
57,712 PointsHi Daniel,
You can use charAt()
on the lastName
variable itself to test the char at index zero. Your test will see if that char is less than or equal to M
. If it is, send the person to line 1, else line 2. You don't need your two char arrays for this.
(To use those, pull out the first char with charAt()
and see what methods would work, such as include
or contains
- I'm not sure but the solution is simpler with the hint above.)
Make sense?
Steve.

Daniel Hunter
4,622 Pointsthanks guys, I needed to read the questions better! i thought I had to prompt the user to put in the name. That all makes much more sense now. I wasn't aware that you can use the less than operator on characters to see their place in the alphabet so I couldn't work out how to categorise them without declaring an array, thanks.
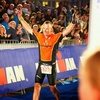
Steve Hunter
57,712 PointsGlad you got it sorted!
Steve.

Yanuar Prakoso
15,196 PointsI agree with Steve Hunter and his solution can be used for your case. But if you insist on using your code since Craig teach you about char array and string prior to the challenge, I have modify your code with some notes:
import java.util.Scanner;
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
String charArray = "abcdefghijklm";// <-- This should be Strings not array of Char (Char[])
//char[] charArray2 = "nopqrstuvwxyz".toCharArray();<---- you do not need this
/*You do not need this: the last name is passed by Example.java not by user input
Scanner scanner = new Scanner(System.in);
System.out.print("Enter Last Name: ");
String nameInput = scanner.nextLine();*/
char initial = lastName.toLowerCase().charAt(0);//<-- make sure you convert the character into lower case first
if (charArray.indexOf(initial) != -1){//<--this is how you use indexOf because indexOf works on String not Char
lineNumber = 1;// you just need to set the line to 1 or 2 no need to increment
} else {
lineNumber = 2;// same case as above
}
return lineNumber;
}
}
I hope you can understand the message in the comment lines. I hope this will help you pass the challenge. Just for comparison sake you can always use Steve's solutions like this:
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
if (lastName.toLowerCase().charAt(0) < 'n'){//<-- NOTE: please remember char uses single quotes
lineNumber = 1;
}else{
lineNumber =2;
}
return lineNumber;
}
}
As you can see it is more practical and shorter. I hope this also helps