Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial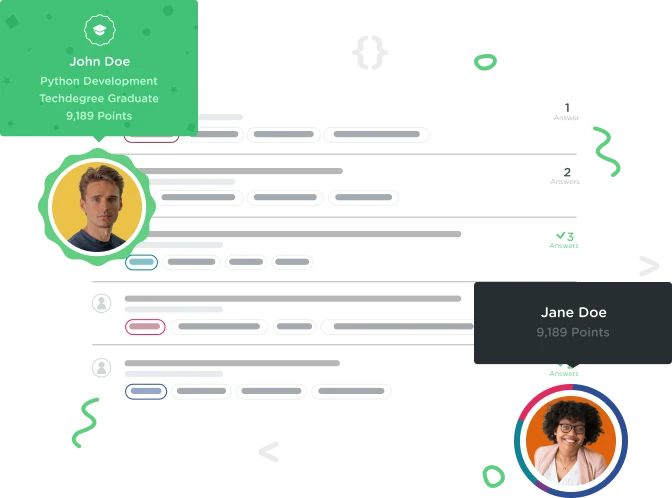

Kavita Bargota
2,741 PointsHow do you call a method that has an "if" statement within it from a class?
class Countries {
let name: String
let size: Int
let disasterRisk: Int
let capital: String
let continent: String
init(name: String, size: Int, disasterRisk: Int, capital: String, continent: String) {
self.name = name
self.size = size
self.disasterRisk = size
self.capital = capital
self.continent = continent
}
func raiseAlarm(if atRisk: Int) {
let scale = 30
if scale > 30 {
print("alert")
}else if scale < 30 {
print("low risk")
}
}
}
let countryStat = Countries(name: "NY", size: 4000, disasterRisk: 30, capital: "NYC", continent: "USA")
countryStat.raiseAlarm(if: 33)
print(countryStat.raiseAlarm(if: 44))
I am stuck!! please help
2 Answers
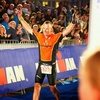
Steve Hunter
57,712 PointsHi there,
You are calling your method correctly with countryStat.raiseAlarm(if: 33)
but the value you pass in is ignored.
Your method receives an integer with an external name of if
and a local name of atRisk
. You then do all your comparisons using a variable that is set internally to 30, scale
. The method prints either "alert" or "low risk" depending whether scale is either above 30, or below 30. It is neither - scale is set to exactly 30 so the method will output nothing. The scenario of scale
being equal to 30 isn't handled by your method. If you change else if
to just else
(with no additional condition) then the method will always output "low risk".
What you want to do is manage the output based on the input integer. Again, we'll handle the condition as being binary; the integer is either greater than 30 or it isn't. No other conditions are required; you may want to tweak the condition depending how you want the scenario of equal to 30 handled; is that an alert or a low risk?
Something like:
func raiseAlarm(if atRisk: Int) {
if atRisk > 30 {
print("alert")
}else {
print("low risk")
}
}
I hope that helps,
Steve.

Kavita Bargota
2,741 PointsHi Steve,
many thanks for your help!
It works! thank your thorough explanation and helping me understand it a bit more
:)
Kavita
Kavita Bargota
2,741 PointsKavita Bargota
2,741 PointsHi Steve,
many thanks for your help!
It works! thank your thorough explanation and helping me understand it a bit more
:)
Kavita
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsAlways happy to help.