Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial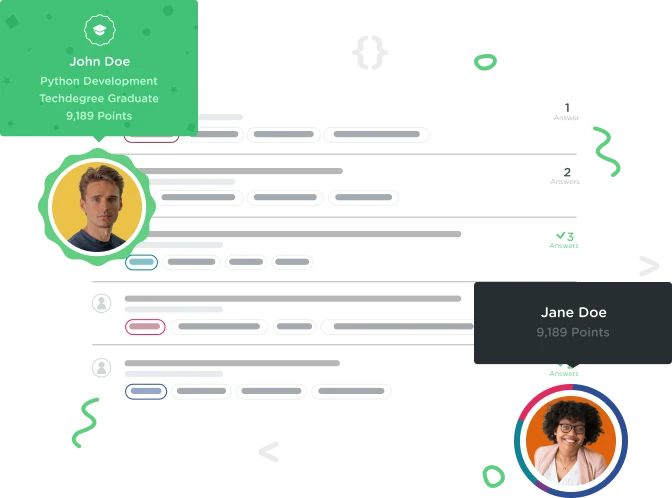

Mamta Chaudhary
527 PointsHow do I use my Identification Class in my Main Class.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
Identification guest = new Identification();
System.out.println("Welcome to the Motel");
System.out.println("Please answer the following Questions to Check In: ");
System.out.println("Enter your Full Name: ");
name = input.nextLine();
System.out.println("Enter your Address: ");
address = input.nextLine();
System.out.println("Date of Birth: ");
dateOfBirth = input.nextInt();
System.out.println("Enter your Identification Card Number or SSC number: ");
iD = input.nextInt();
}
}
public class Identification {
private String guestName;
private String guestAddress;
private int guestDateOfBirth;
private int guestIdentificationNumber;
public String getGuestName () {
return guestName;
}
public void setGuestName (String name){
guestName = name;
}
public String getGuestAddress () {
return guestAddress;
}
public void setGuestAddress (String address){
guestAddress = address;
}
public int getGuestDateOfBirth () {
return guestDateOfBirth;
}
public void setGuestDateOfBirth ( int dateOfBirth){
guestDateOfBirth = dateOfBirth;
}
public int getGuestIdentificationNumber () {
return guestIdentificationNumber;
}
public void setGuestIdentificationNumber ( int iD){
guestIdentificationNumber = iD;
}
}
1 Answer
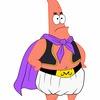
<noob />
17,062 PointsHi, Sorry if i confuse u even more as im still new to java, as far as i know this is the correct way to do this: first, You have to create a constructor for all of the "guess" variable in order to initalize them. and only after that u can use the getters like u did here Why is that?
Answer: right now u just declaring the variables, they do not contain anything, u need to declare the variables in order to store the parameters the users are entering from the constructor. u do it like that:
public Identification (String guestName, String guestAddress, int guestDateOfBirt, int guestIdentificationNumber){
this.guestName = guestName
this.guestAddress= guestAddress;
this.guestDateOfBirth = guestDateOfBirth;
this. guestIdentificationNumber = guestDateOfBirth;
}
after that u just create a new instance of the class as u did, u named it guess. and then u use System.out.readLine method and declare the type of the variable which is string
String name = console.readLine("Enter your name");
System.out.printf( "your name is %s"guest.getGuestName());
if this answer helped u please mark as best answer :D
Mamta Chaudhary
527 PointsMamta Chaudhary
527 PointsBut how would I use the guestName, guestAddress, and others in the other public class Main()? When I enter the name = input.nextLine(); , it states that is cannot resolve symbol "name". I know I could just clarify and put String before name, and do the same for the others but I am trying to incorporate the name from the Identifiction class. Also Thank You for the Help.