Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial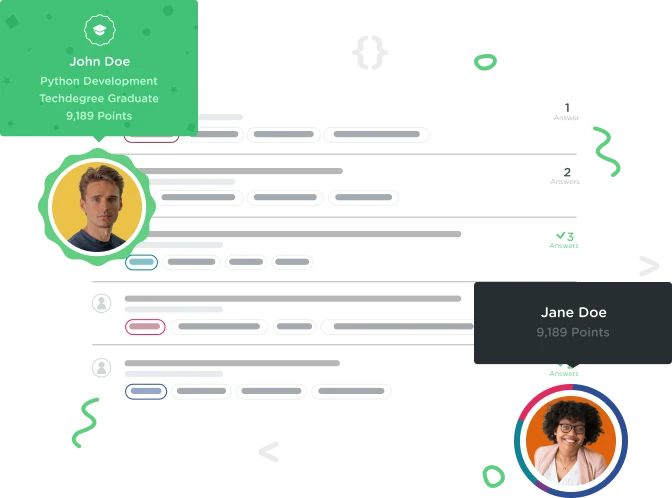
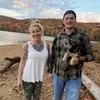
Jennifer Barbee
Front End Web Development Techdegree Student 2,387 PointsHow do I pass in first name and last name? (Step 4/4)
I'm not sure what I'm doing wrong here. It appears that something with the first name and last name are not running correctly.
public class Forum {
private String topic;
private Forum(String topic) {
this.topic = topic;
}
// TODO: add a constructor that accepts a topic and sets the private field topic
public String getTopic() {
return topic;
}
/* Uncomment this when you are prompted to do so
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
*/
}
public class User {
// TODO: add private fields for firstName and lastName
private String firstName;
private String lastName;
public setFirstName() {
firstName = this.firstName;
}
public void setLastName() {
lastName = this.lastName;
}
public User(String firstName, String lastName) {
// TODO: set and add the private fields
this.firstName = "Jen";
this.lastName = "Ell";
}
// TODO: add getters for firstName and lastName
public String getFirstName() {
return this.firstName;
}
public String getLastName() {
return this.lastName;
}
}
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
// TODO: add a constructor that accepts the author, title and description
public ForumPost(User author, String title, String description){
mAuthor = author;
mTitle = title;
mDescription = description;
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User(lastName,firstName);
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost(author, "This is the forumPost title",
"This is the forum post description");
forum.addPost(post);
}
}
2 Answers
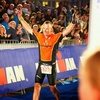
Steve Hunter
57,712 PointsHi Jennifer,
You don't need to set the values of the args - the challenge does that for you.
Leave main
how it is and just use args[0]
and args[1]
when you create the User
object.
Everything else you've done looks spot on!
Steve.
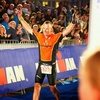
Steve Hunter
57,712 PointsHi Jennifer,
The main
takes an array of Strings as it's argument when the program is started.
The first two elements of this array, which is called args[]
are the first and last names. So, pass in args[0]
and args[1]
to create the User
object in main.java
.
Plus, the comment to your question is correct. You can't hard code the values inside the User constructor; use the parameters that are passed in instead. The two required parameters to create as User
onject are received by the constructor so should be assiigned into the member variables using the .this
keyword.
I hope that helps,
Steve.
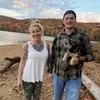
Jennifer Barbee
Front End Web Development Techdegree Student 2,387 PointsHey Steve, I appreciate your help. I adjusted my code so that the constructor parameters in User.java are firstName and lastName rather than "Jen" and "Ell".
I also initialized in Main: args[0] = "Jen"; args[1] = "Ell";
and then called args [0] and args[1]:
User author = new User(args[0],args[1]);
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost(author, "This is the forumPost title",
"This is the forum post description");
However, I'm still getting some errors. Did I misinterpret how to pass in args[0] and args[1]?
Chaltu Oli
4,915 PointsChaltu Oli
4,915 PointsIn the constructor, you're supposed to make the parameters you passed equal to the private field; for example
Instead of setting the name equla inside the counstructor try making it equal the parmeters you passed like this
Then set the first and last name when you call the constructor in the main.java folder.
[MOD: edited code blocks - srh]