Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial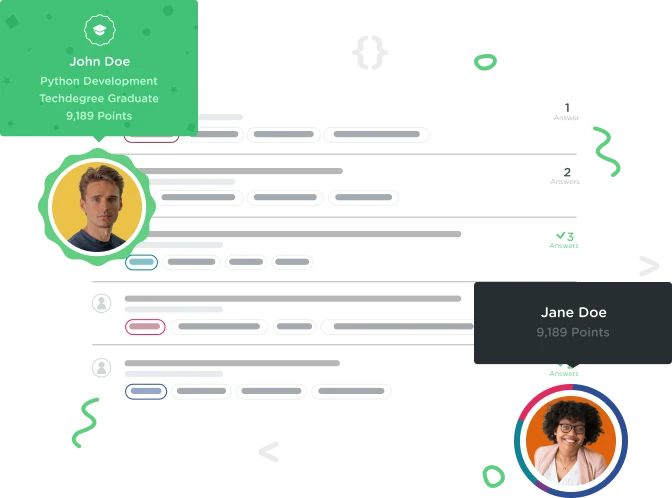
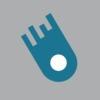
Gabriel E
8,916 PointsHow do I loop through this Map?
Hi there,
I'm on my last challenge of this chapter, and I'm thoroughly confused. I looked over the maps I created in my Treet code, but I can't seem to customize it for the needs of the challenge. Any suggestions? Thanks for the help!
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
}
1 Answer
Christopher Augg
21,223 PointsHello gabriel,
We know from the instructions that we will be editing the Blog.java file. We also know that we will be working with Maps. Therefore, the first thing we want to do is decide on the type of map we want to use and add our required imports. For example, lets say we decide on using a TreeMap. We would import the following:
import java.util.Map;
import java.util.TreeMap;
Now we want to make sure we understand the instructions for the Types the map will use for the Key and Value. The instructions state:
In Blog.java add a new method called getCategoryCounts. It should return a Map of category to count calculated by looping over all the posts.
hmmm. It should return a Map of category to count......
Do we have a category somewhere? That's right! We keep a String named mCategory in our BlogPost. The Key must be just a String. What about count? No, we do not have that anywhere. The instructions say that it will be calculated by looping over all the posts. Well, a count is simply an int. However, we cannot use int with our Map because Maps do not allow primitive data types. We will need to use Integer. See Map Documentation .
With this information we can write a skeleton method:
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> catCounts = new TreeMap<String, Integer>();
return catCounts;
}
Now we have a basic method that will return a map that can hold Key-Value pairs of Strings to Integers that will represent the categories and their respective counts. However, in order for it to work, we will need to loop over all the BlogPosts, get each category as we do, and increment the count for any like categories.
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> catCounts = new TreeMap<String, Integer>();
// loop over all posts
for (BlogPost post: mPosts) {
// get each category as a String
String category = post.getCategory();
// make sure if the Key is in the map because we will get an exception if we called
// the map's get method on something that does not exist.
if (catCounts.containsKey(category)) {
// since we know the key exists, put the category back in the map as the Key. The Value
// will be set calling the Map's get method to get the last count, increment it by 1, and place
// new count into map
catCounts.put(category, catCounts.get(category) + 1);
} else {
// since the key does not exist, just put in category with count of 1
catCounts.put(category, 1);
}
}
return catCounts;
}
I hope this helps. Please let me know if I can help you further.
Regards,
Chris