Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial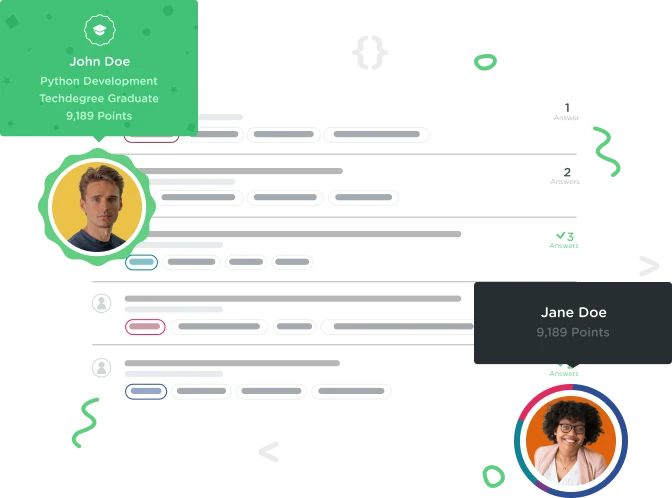

Michael Nanni
7,358 PointsHow can I test out my code in workspaces?
I've been trying to test this out in the interactive shell from workspaces, but I keep getting errors when trying to import these classes. It's not passing the challenge, but I think I can figure it out once I know how to test it.
What command do I use?
>>> from morse.py import S
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ModuleNotFoundError: No module named 'morse.py'; 'morse' is not a package
Thanks!
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
seperator = '-'
super().__init__(pattern)
def __str__(self, pattern):
for i in pattern:
if i = '.':
pattern[i] = 'dot'
elif i = '_':
pattern[i] = 'dash'
print(seperator.join(pattern))
3 Answers

KRIS NIKOLAISEN
54,971 PointsYou see this error message
TypeError: __str__ returned non-string (type NoneType)
because your __str__
method doesn't return anything so print(test)
is attempting to print None
which is the return value when there is no return statement.
I would have thought you'd have another error first since there should be a colon at the end of this line
def __str__(self)
Some additional comments:
You are iterating through self.pattern but then update self.pattern inside the loop with the assignments. It would be better to create a separate list variable before the loop and then append to this list based on your if conditions
You have the join syntax reversed. It should be
separator.join(list)
instead oflist.join(separator)

KRIS NIKOLAISEN
54,971 Pointspattern
is a parameter for __init__
so it doesn't need to be passed in again to __str__
. The only parameter needed there is self
. Inside the __str__
method you can use it as self.pattern
.

Michael Nanni
7,358 PointsHey Kris. I'm getting back to this after a while, but I still seem to be coming across errors in the shell. Maybe I didn't understand your last comment. Hopefully it's something small that I'm just overlooking. Thanks in advance for your help!
In the shell:
>>> from morse import Letter
>>> test = Letter(['.', '.', '.'])
>>> print(test)
-
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: __str__ returned non-string (type NoneType)
>>>
And my code:
class Letter:
def __init__(self, pattern=None, seperator='-'):
self.pattern=pattern
self.seperator=seperator
def __str__(self)
for i in self.pattern:
if i == '.':
self.pattern = 'dot'
elif i == '_':
self.pattern = 'dash'
print(self.pattern.join(self.seperator))
class S(Letter):
def __init__(self):
self.seperator = '-'
self.pattern = ['.', '.', '.']
super().__init__(pattern)

KRIS NIKOLAISEN
54,971 PointsFor the challenge you are to modify class Letter not class S.
I want you to add a
__str__
method to the Letter class
Then to test import without the .py extension, create an instance of the class and print.
>>> from morse import Letter
>>> test = Letter(['.', '.', '.'])
>>> print(test)
The result should be
dot-dot-dot

Michael Nanni
7,358 PointsThanks Kris! At first I was getting tab errors with those commands, so I typed it out by hand and that cleared it up. However, now I'm getting a TypeError.
My code
class Letter:
def __init__(self, pattern=None):
self.pattern=pattern
def __str__(self, pattern):
for i in pattern:
if i == '.':
pattern[i] = 'dot'
elif i == '_':
pattern[i] = 'dash'
print(seperator.join(pattern))
class S(Letter):
def __init__(self):
seperator = '-'
pattern = ['.', '.', '.']
super().__init__(pattern)
In the shell.
>>> from morse import Letter
>>> test = Letter(['.', '.', '.'])
>>> print(test)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: __str__() missing 1 required positional argument: 'pattern'
What is my method missing there?
Michael Nanni
7,358 PointsMichael Nanni
7,358 PointsThat worked! I made some changes based on your comments and managed to pass the challenge. Still the original question remains for me. How can I run this through the shell to get the output I'm returning?