Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial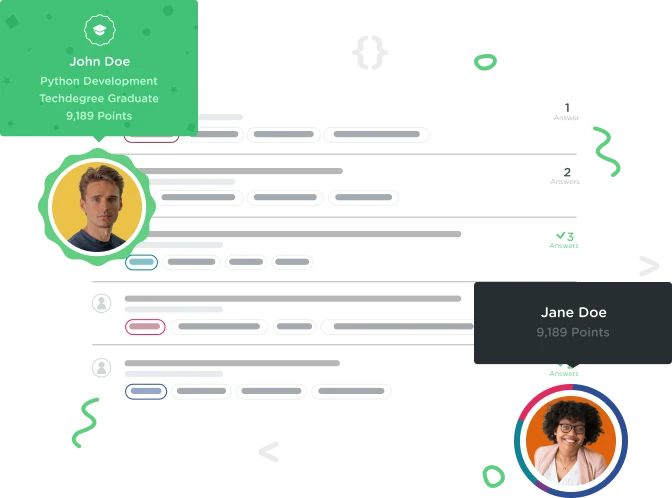

Alfie Miller
2,266 PointsHow can I code for an app to automatically load into the iPhones camera (being the apps default screen)
I was wondering what the code is for an app to access the iPhones camera (main.storyboard of app) with other declarations that would be within the UIImagePickerController, so the app loads into the phone camera automatically from the app icon in home screen ?
and would I put it in the viewDidLoad initialiser of viewController
8 Answers
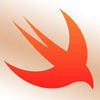
Jeff McDivitt
23,970 PointsYour complete code should look something like this you need to include the proper delegates
class ViewController: UIViewController, UINavigationControllerDelegate, UIImagePickerControllerDelegate {
let imagePicker = UIImagePickerController()
@IBOutlet weak var imageViewer: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func imagePickerController(picker: UIImagePickerController, didFinishPickingImage image: UIImage!, editingInfo: [NSObject : AnyObject]!) {
dismissViewControllerAnimated(true, completion: nil)
imageViewer.image = image
}
func imagePickerController(picker: UIImagePickerController, didFinishPickingImage image: UIImage!, editingInfo: [NSObject : AnyObject]!) {
dismissViewControllerAnimated(true, completion: nil)
imageViewer.image = image
}
}
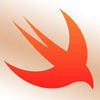
Jeff McDivitt
23,970 PointsHi - Loading it in viewDidLoad will not work, but here is what you will need to do
override func viewDidAppear(animated: Bool) {
if UIImagePickerController.isCameraDeviceAvailable( UIImagePickerControllerCameraDevice.Front) {
imagePicker.delegate = self
imagePicker.sourceType = UIImagePickerControllerSourceType.Camera
presentViewController(imagePicker, animated: true, completion: nil)
}
}

Alfie Miller
2,266 PointsThank you, but also at the moment the imagePicker is an unresolved identifier. How do I resolve this as I've tried stating imagePicker but not working so how do I code for that ? Thanks again
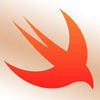
Jeff McDivitt
23,970 PointsDid you add the UIPickerControllerDelegate?

Alfie Miller
2,266 PointsI have now but more errors have occurred, it says "ViewController does not conform to protocol NSObjectProtocol" and imagePicker is still unidentified as well as presentViewController?? Don't know why I'm struggling with this so much but you are being very helpful, thanks
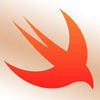
Jeff McDivitt
23,970 PointsCan you paste your code

Alfie Miller
2,266 Pointsimport UIKit
class ViewController: UIImagePickerControllerDelegate {
func viewDidAppear(_animated: Bool) {
if UIImagePickerController.isCameraDeviceAvailable(UIImagePickerControllerCameraDevice.front) {
imagePicker.delegate = self
imagePicker.sourceType = UIImagePickerControllerSourceType.camera
presentViewController(imagePicker, animated: true, completion: nil)
}
func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
}
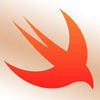
Jeff McDivitt
23,970 PointsThere error is caused because you have a UI Element that is not connected

Alfie Miller
2,266 PointsI think this was caused because it made me change the last bit of code to imagePickerControllerDidCancel instead of just imagePickerController but when I try and keep it the same as your code, it brings up error that I've already previously declared that code from the 1st 'func imagePickerController' code and build fails completely. Is there something you missed out or how does your code work an not mine??
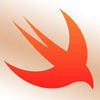
Jeff McDivitt
23,970 PointsMine works. You sure there is not an error somewhere else in your program?

Alfie Miller
2,266 PointsI just tried building it without the last bit of code so there was no redeclaration of imagePickerController, and it came up with the same error as when I had the last bit as imagePickerControllerDidCancel, which you said something isn't connected. Can you see if there is anything not connected, ill show you my code:
import UIKit
class ViewController: UIViewController, UINavigationControllerDelegate, UIImagePickerControllerDelegate { let imagePicker = UIImagePickerController() @IBOutlet weak var imageViewer: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func imagePickerController(picker: UIImagePickerController, didFinishPickingImage image: UIImage!, editingInfo: [NSObject : AnyObject]!) {
dismiss(animated: true, completion: nil)
imageViewer.image = image
}
func imagePickerController(picker: UIImagePickerController, didFinishPickingImage image: UIImage!, editingInfo: [NSObject : AnyObject]!) {
dismiss(animated: true, completion: nil)
imageViewer.image = image
}
}
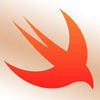
Jeff McDivitt
23,970 PointsI cannot see that by just your code, typically this happens when one of you labels or image view view or something is not connected. Just double check everything is in Xcode

Alfie Miller
2,266 PointsOk thanks, while I figure out this code and correct it, one question: why have you actually stated the exact same piece of code twice?
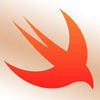
Jeff McDivitt
23,970 PointsThat was my mistake it should only be in there one time

Alfie Miller
2,266 Pointsthe build succeeds now but when attaching the code to run it on iPhone, it came up with "error: attach by pid '53843' failed - unable to attach" ?? don't know what this means
Alfie Miller
2,266 PointsAlfie Miller
2,266 PointsThank you, the code you have put down compiles but when I try and run the app it says 'build successful' but then when running the app it comes up with some sort of error in Thread 1 or something Here's code: class AppDelegate: UIResponder, UIApplicationDelegate { - Error is Thread 1: signal SIGABRT ??
what should I do because app doesn't run completely yet ?