Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial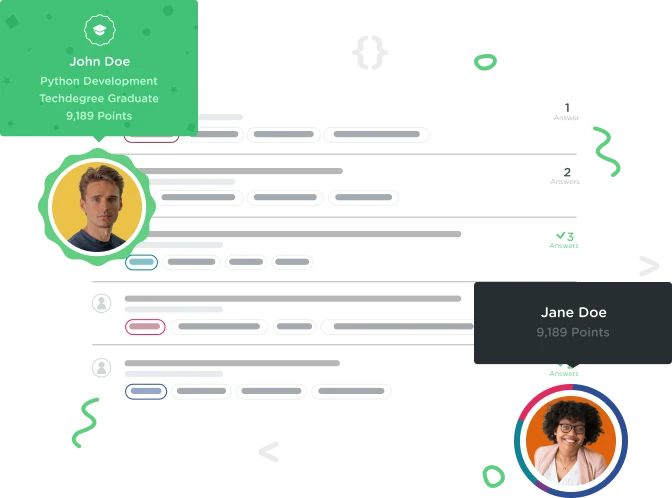

Jonathan Archer
9,786 PointsHow can I add a "No results found" response if the Flickr API returns no results?
At the end of this video, Dave suggests adding a "No results found matching your search" response if the Flickr API returns no items.
I experimented with adding an if/else loop to the callback function, but kept breaking the code. My Google-fu was not able to turn up an answer. Can anyone help?
5 Answers
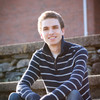
Andrew Lincoln
1,732 Pointsyou could wrap your .each method. If there is no data to be displayed, the if block be false and go to the else block to log out to the client that no results have been found. Let me do a quick example with your code... again taking it out of the context of the lesson since I'm not too familiar.
There is a few ways we can go ahead and implement this feature. This is my take and it may not compile since I'm not in the context of the project and I'm not debugging.
I will also do a little code clean up. :)
$(document).ready(function () {
$('form').submit(function (evt) {
evt.preventDefault();
var $searchField = $('#search'),
$submitButton = $('#submit'),
// the AJAX part
flickerAPI = "http://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=?",
animal = $searchField.val(),
flickrOptions = {
tags: animal,
format: "json"
$searchField.prop("disabled", true);
$submitButton.attr("disabled", true).val("searching...");
};
function displayPhotos(data) {
var photoHTML = '<ul>';
//checking to see if we have items to be displayed.
if (data.items !== 0) {
$.each(data.items, function (i, photo) {
var date = new Date(photo.date_taken);
photoHTML += '<li class="grid-25 tablet-grid-50">';
photoHTML += '<a href="' + photo.link + '" class="image">';
photoHTML += '<img src="' + photo.media.m + '">';
photoHTML += '</a><p>Photo by<br>' + photo.author.slice(19, -1) + '<br>on '
+ photo.date_taken.slice(0, 10) + ' at '
+ photo.date_taken.slice(11, 16) + '</p></li>';
}); // end each
photoHTML += '</ul>';
} else {
//this is the html rendered to the screen incase we have no search results.
photosHTML = "Sorry no results found man!";
} //end if/else block
$('#photos').html(photoHTML);
$searchField.prop("disabled", false);
$submitButton.attr("disabled", false).val("Submit");
} //end displayPhotos function
$.getJSON(flickerAPI, flickrOptions, displayPhotos);
}); // end submit
//check to make sure there is data to be rendered
//if data exists ... render to screen
// else print message to client
}); // end ready

Kan Dong
17,368 PointsI was trying this additional challenge as well. Originally, I looked for status code for AJAX and had trouble with code; after reading this, I realized my mistakes. Thanks for discussing your solutions, Jonathan and Andrew. It really helped!
Jonathan's code didn't quite work for me. But I made some changes and it's working for me now.
function displayPhotos(data) {
var photoHTML = '<ul>';
//checking to see if we have items to be displayed.
if (data.items.length !== 0) {
$('#error').empty();
$.each(data.items, function (i, photo) {
photoHTML += '<li class="grid-25 tablet-grid-50">';
photoHTML += '<a href="' + photo.link + '" class="image">';
photoHTML += '<img src="' + photo.media.m + '">';
}); // end each
} else {
//this is the html rendered to the screen incase we have no search results.
var errorHTML = '<p>No results found for "' + searchTerm + '".</p>';
$('#error').html(errorHTML);
} //end if/else block
photoHTML += '</ul>';
$('#photos').html(photoHTML);
$searchField.prop("disabled", false);
$submitButton.attr("disabled", false).val("Search");
} //end displayPhotos function
Also added a div with id of error in the html for displaying error message.
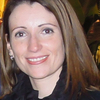
Irina Blumenfeld
9,198 PointsThanks for posting Kan. This helped me get my code to work.
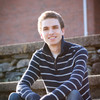
Andrew Lincoln
1,732 PointsI have another challenge for you, see if you can use a standard for loop instead of the .each block. The for loop is more efficient and you will see faster load times. Also Notice how I separated your variables with commas. This is best practice, you only need to use the var keyword once and with all subsequent variables we can exclude it. Another efficiency tip :) hope this helped!

Jonathan Archer
9,786 PointsThanks, mate. I'll try the for loop sometime. (Lesson block was on AJAX hence taking that approach.)
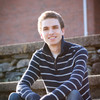
Andrew Lincoln
1,732 Pointsjust do a conditional check.. it should look something along the lines of this... not familiar with the lesson so this will be out of context but bare with me.
var noResults = function(){
if(JSONobject.property === 0){
$(this).html('No results found dude!');
}
}

Jonathan Archer
9,786 PointsMakes sense but I can't find the right place to implement it.
Here is my code as it stands, if you have suggestions.
$(document).ready(function() {
$('form').submit(function(evt) {
evt.preventDefault();
var $searchField = $('#search');
var $submitButton = $('#submit');
$searchField.prop("disabled", true);
$submitButton.attr("disabled", true).val("searching...");
// the AJAX part
var flickerAPI = "http://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=?";
var animal = $searchField.val();
var flickrOptions = {
tags: animal,
format: "json"
};
function displayPhotos(data) {
var photoHTML = '<ul>';
$.each(data.items,function(i,photo) {
var date = new Date(photo.date_taken);
photoHTML += '<li class="grid-25 tablet-grid-50">';
photoHTML += '<a href="' + photo.link + '" class="image">';
photoHTML += '<img src="' + photo.media.m + '">';
photoHTML += '</a><p>Photo by<br>' + photo.author.slice(19, -1) + '<br>on ' + photo.date_taken.slice(0, 10) + ' at ' + photo.date_taken.slice(11, 16) + '</p></li>';
}); // end each
photoHTML += '</ul>';
$('#photos').html(photoHTML);
$searchField.prop("disabled", false);
$submitButton.attr("disabled", false).val("Submit");
}
$.getJSON(flickerAPI, flickrOptions, displayPhotos);
}); // end submit
}); // end ready
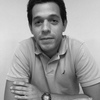
Daniel Mejia
12,481 PointsThanks for posting this guys. I have one question. I tried Andrew's code and didn't work at first with the if statement like so.
if (data.items !== 0)
Then I changed it to how Jonathan has it
if (data.items != 0)
and it worked.
Can anyone tell me why this tiny change made the difference? I appreciate your response.

jamestoynton
10,992 PointsThis works for me , but is there a reason simply using if(data) won't work?
Jonathan Archer
9,786 PointsJonathan Archer
9,786 PointsAwesome! That's what I had tried, but obviously got some of the syntax wrong. (And thanks muchly for the code clean up. )
With a couple tweaks I got it working in context. Posting code snippet in case anyone else on this lesson might find it useful.