Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial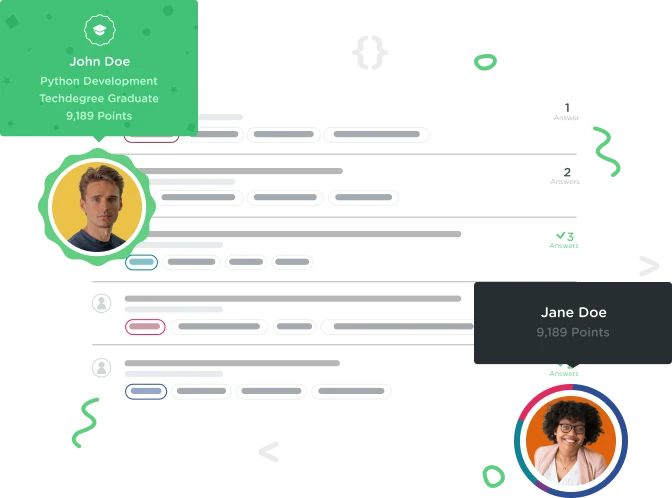

Izzy Davidowitz
681 PointsHelp with extra credit stage 3 - using method on string called contains
Am lost with this extra credit question - use method on string called contains to see if any one of the words included are on the "bad list". Get the concept, just need a little help on how to set up/implement. Googled "java method contains" did not seems to get anything helpful back.
10 Answers

Ken Alger
Treehouse TeacherIzzy;
1.
Odd Chrome "Feature" it sounds like.
2.
I was a bit confused by the instructions as well. One of the general ways in which Treehouse operates their courses is that they try not to have you do things that they have not taught yet. For example, in the Java course there isn't a challenge question asking us to access a database to get the information for the list of questions. The Java Basics course didn't get into actual lists or arrays, I don't believe. As such, it would be unusual for the challenge, or even the extra credit, to want us to implement an actual list of words to check against. That would require, generally speaking, the importing of an additional Java class (such as java.util.ArrayList
) and knowing how to do some interaction with arrays or lists.
All that to say, the best I could come up with is to go back to the or
operator and check for a bunch of different words using the contains
method. Perhaps I am missing something simple and am stuck thinking in terms of Arrays, List<String>, String Lists, etc. for the solution. Maybe Craig Dennis would show a hint of the direction he was thinking of given the teachings thus far.
3.
In terms of why noun
is inside the do...while block and word
is defined outside, I'll see if my over simplified explanation makes sense. We define our variables and assign their type (String, Boolean, Int, etc.) outside of repetitive code (do...while, for, etc.) because the don't need to be redefined or re-typed during each time through the code. In the case of the variable noun
we aren't re-typing it, we are reassigning it's value each time we are in the do...while block, but we don't need to tell Java each time it is a String. We certainly could have declared String who;
outside of the do...while, and assigned it a value inside the block without an issue, but that's kind of repetitive when it comes to memory allocation.
Think of when you attend a social gathering and you meet new people. Once they have introduced (declared) themselves, "String who" or "String noun" you really don't want them telling you for the rest of the conversation, Hey, I'm "String noun". That only needs to be done once. Putting it inside a do...while block makes Java angry. Having
noun
ask the same question over and over would be like a guest roaming around asking if you've seen his car keys. He's on a mission and until someone tells him "yes", or in our case doesn't call him a "nerd" or variation thereof, he asks the same question over and over again.
Hopefully that makes some sense and hope that I have helped more than hindered the learning process.
Ken

Craig Dennis
Treehouse TeacherSorry for the lack of clarity!
I was thinking of something like this to avoid the logical OR repetition. You could have a master String
of things you don't want to see. Like this:
String badWords = "nerd dork jerk lame";
badWords.contains(noun.toLowerCase());
And yes Ken Alger I only intended what we learned in the course, along with these two methods! Nice instinct!
btw Ken, you are an amazing moderator! Thanks for all your help!

Ken Alger
Treehouse TeacherI tried that earlier and I think I disregarded it because I was not getting the same functionality in the list as in a single word using the contains
method. So if you do:
String badWord = "nerd";
isInvalidWord = noun.contains(badWord);
that will catch things like nerdly
, big nerd
, nerdmeister
, etc. Whereas badWord.contains(noun)
only catches nerd
.
I worked with badwords = "nerd dork jerk"
and I must have reveresed the variable names in the badWords.contains(noun)
statement to noun.contains(badWords)
. This leads to nerd
being considered a "bad word", but the rest of them in the string not being an invalid word.
The toLowerCase()
method is rather straight forward.
Thanks,
Ken

Nicholas Demetriou
639 PointsHi Greg
where do we add this?
String badWords = "nerd dork jerk lame"; badWords.contains(noun.toLowerCase());

Cristofer Hernandez
440 PointsWhen I use:
String badWords = "nerd dork jerk lame";
as Craig Dennis offered, The code just continues on rather than prompting for another word not on the bad list. I believe the list does not properly separate the words and instead implies that "nerd dork jerk lame" is one long bad word. How do we list multiple words within the String badWords so that any one of them entered prompts for a new non-bad word?

May Loh
5,213 PointsI'm puzzled about the master list of bad words. Should it be an array or list instead? I tried adding in a list of words but it reads them as a whole word on its own.
Eg. "jackass dork idiot" as one word, not "jackass", "dork", "idiot".

Ken Alger
Treehouse TeacherIzzy;
Extra Credit
Task 1
There is a method on string called contains
. It checks to see if one string is contained within the other. This seems like a good way to build a master list of words that are not allowed. Why don't see if what they typed is in a long string of bad words.
In looking at this we are told about a method called contains
. If we look at the Java documentation on Strings, the contains
method returns a boolean value and returns true if and only if this string contains the specified sequence of char values
.
We should, therefore be able to do something like:
isInvalidWord = (noun.contains(word));
If we then define our String word
outside the do...while block thusly,
String word = "nerd";
The checker would look for words like "nerd", "nerdly", "nerdmeister", etc.
The Extra Credit wants to check for a long string of bad words
. I'll let you interpret that as you see fit.
Task 2
After you get that working, attempt to take case into account. If your list of bad words that you maintain is all lower cased, you can check the lower case version of the input in the bad string. How do you make a string lowercase?
There are a couple of options here, but this shouldn't give you too much trouble if you are comfortable with Java methods. There are actually a few different approaches.
Happy coding,
Ken

miguel narvaez
440 PointsCraig, no worries i got it to work...thanks

Izzy Davidowitz
681 PointsHi Ken -
Didn't use anything yet - I'm stuck at were I'd use contains and the proper syntax for it. Here's what we have so far - the goal is to have it look through the strings in Contains and give the "That language is not allowed" message if there are any matches.
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here!
*/
String ageAsString = console.readLine("How old are you? ");
int age = Integer.parseInt(ageAsString);
if (age < 13) {
console.printf("Sorry you must be at least 13 to use this program.\n");
System.exit(0);
}
String name = console.readLine("Enter your name: ");
String adjective = console.readLine("Enter an adjective: ");
String noun;
Boolean isInvalidWord;
do {
noun = console.readLine("Enter a noun: ");
isInvalidWord = (noun.equalsIgnoreCase("dork") ||
noun.equalsIgnoreCase("jerk"));
if (isInvalidWord) {
console.printf("That language is not allowed. Try again. \n\n");
}
} while(isInvalidWord);
String adverb = console.readLine("Enter an adjective ");
String verb = console.readLine("Enter a verb ending in -ing: ");
console.printf("Your TreeStory:\n-----------------\n");
console.printf("%s is a %s %s. ", name, adjective, noun);
console.printf("They are always %s %s . \n", adverb, verb);
}
}

Izzy Davidowitz
681 PointsThanks so much Ken. Will go through it tomorrow. One issue is that the link to java/oracle documentation was giving me an error. (https://docs.oracle.com/javase/7/docs/api/java/lang/String.html) saw you linked to that too... hopefuly it's back up soon.
Izzy

Ken Alger
Treehouse TeacherI just checked the Oracle link and it is there and working. Do a search on the page for the contains
method.
Happy coding,
Ken

Izzy Davidowitz
681 PointsKen
For some reason that link to java (in chrome) is https which returns an error. Changing to http solved that
Was able to create method that will not allow any variation of the string to pass. Am not able to figure out how to add more words to that string though. What I have so far:
String word = "nerd";
- Would you be able to explain why for noun we had to define it within the do, however word is defined outside the do and that does not create a scoping issue.
Thanks again!

Izzy Davidowitz
681 PointsThanks Ken and Craig. Still slightly confused but much more ahead than before these answers. Starting java objects class now, hope that clarifies things further.

miguel narvaez
440 PointsWhat is the scope of this code? Eliminate the ||(Or) statements all together? An example here would be nice?
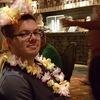
Ryan Lee
13,806 PointsI struggled with this one for hours, but I refused to quit until I got it.
I kept trying to put what I have below outside of the do syntax when it was obviously supposed to go inside.
isInvalidWord = (noun.contains(badWord));
Anyway, here is my working code for the first problem if anyone is still having trouble.
import java.io.Console;
public class Treestory {
public static void main(String[] args) {
Console console = System.console();
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here:
*/
String ageAsString = console.readLine("How old are you? ");
int age = Integer.parseInt(ageAsString);
if (age < 13) {
//Insert exit code
console.printf("Sorry you must be at least 13 to use this program.\n");
System.exit(0);
}
String name = console.readLine("Tell me your name: ");
String adjective = console.readLine("Do you even know what a adjective is? Prove it: ");
String noun;
String badWord = "nerd";
boolean isInvalidWord;
do {
noun = console.readLine("Do one of 'dem 'dere nouns: ");
isInvalidWord = (noun.contains(badWord));
if (isInvalidWord) {
console.printf("That language is not allowed. Try again. \n\n");
}
} while(isInvalidWord);
String adverb = console.readLine("How 'bout a good 'ol adverb?: ");
String verb = console.readLine("Verb! Do it now!: ");
console.printf("%s is a %s %s. ", name, adjective, noun);
console.printf("They are always %s %s.", adverb, verb);
}
}
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherIzzy;
Welcome to Treehouse!
Would you mind posting the code you are using and let's see if we can get this sorted out.
Ken