Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial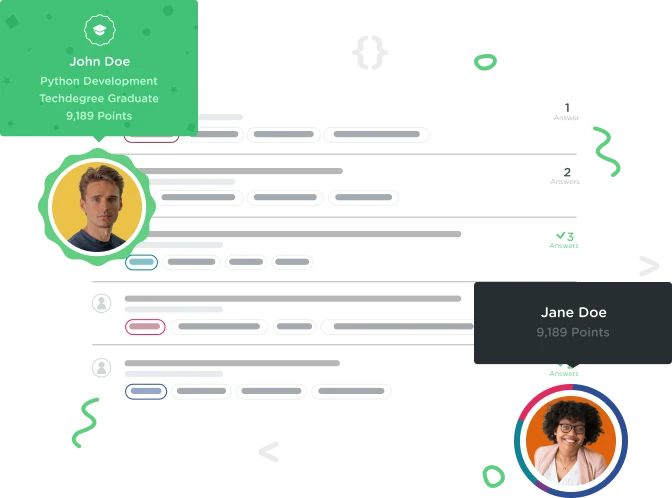

chaya aizenman
Courses Plus Student 405 Pointshelp!! why is my 'mAuther' not set correctly?
any one can help out?
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author,String title,String body, String category,Date creationDate){
String mAuthor = author;
String mTitle = title;
String mBody = body;
String mCategory = category;
Date mCreationDate = creationDate ;
}
}
1 Answer
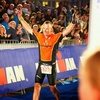
Steve Hunter
57,712 PointsHi there,
I do get the error you mention when I use your code. The reason for this is you have redeclared your member variables inside the constructor.
You don't need to re-declare them as String or Date; you have done that inside the class. So, modify your constructor to assign the parameters to the variable without redeclaring them:
public BlogPost(String author, String title, String body, String category, Date creationDate){
mAuthor = author; // remove String
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate ;
}
Leaving the data type in there restricts the scope of the variables to inside the constructor, so the values passed in as a parameter will not be assigned to your member variables as you intend.
I hope that helps,
Steve.
chaya aizenman
Courses Plus Student 405 Pointschaya aizenman
Courses Plus Student 405 PointsThanx!
Ivan Kazakov
Courses Plus Student 43,317 PointsIvan Kazakov
Courses Plus Student 43,317 PointsHi,
it's not that 'you don't need to re-declare', but you rather shouldn't, or I'd even say you must not. What you're actually doing inside the constructor is assigning values to previously declared private member variables. The suggestion above is correct, and for even more clarity, you can write like this:
'this.' prefix refers to the very class that's being defined, so
this.mAuthor
refers to the 'mAuthor' class member variable, and it's in fact anyway applied implicitly by the compiler, even if you don't write it explicitly, AND IT FINDS NO local variable with the same name declared within the same constructor.
Yes, you actually can (but strongly advised not to) for some reason declare a local variable inside a constructor with the same name as a class member variable (you actually did exactly that in your initial version of the constructor):
in this case, you will have to explicitly write 'this.' prefix before an actual member variable for the compiler to be able to distinguish it from your local variable with the same name:
The code above will work, but strongly advised to be avoided at all costs.