Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial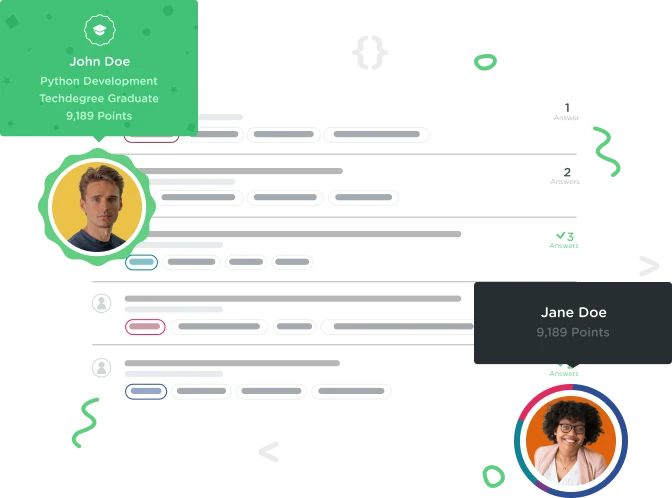

jinhwa yoo
10,042 Pointshelp me ...
as i expect I don't know.. hahah
3 Answers
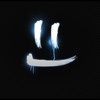
Grigorij Schleifer
10,365 PointsHey jinhwa,
you will make it ... just believe it :)
Here my code proposal:
public static String getTitleFromObject(Object obj) {
//this method takes an Object as argument
// not a String or BlogBost just an object
String result = "";
BlogPost blog = null;
// here we initialize (same as declaring) an empty String and an empty BlogPost
// empty BlogPost is "null" and empty String is " "
// we need them to ibe nitialized, so they can exist inside the if statements
if (obj instanceof String) {
// instanceof method is analizing the Object "obj" we passed in as argument
// if this Object is instance of a String or BlogPost (instance is same as kind or type)
// we need to cast the Object that we passed in
// into a String (String) or BlogPost (BlogPost)
// this is important because the compiler doesn,´t know wich kind of object is the argument
// only we know that this is a String or BlogPost
// don´t forget ... the compiler is stupid :)
result = (String) obj;
// casting obj into String
return result;
}
if (obj instanceof BlogPost) {
blog = (BlogPost) obj;
// casting obj into BlogPost
}
return blog.getTitle();
// after typecasting we return String or BlogPost
// if it is BlogPost we return the title of the blog
}
Does it make sense?
Grigorij

jinhwa yoo
10,042 Pointsif (obj instanceof BlogPost) {
blog = (BlogPost) obj;---->()why use this?
// casting obj into BlogPost
}
return blog.getTitle();---->where is getTitle ();????
blog = (BlogPost) obj;---> BlogPost blog = new BlogPost; is it the same meaning?
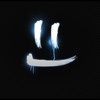
Grigorij Schleifer
10,365 PointsHey,
so let see the first question:
if (obj instanceof BlogPost) { blog = (BlogPost) obj;---->()why use this?
In this case you use the parenthesis () for casting "obj" (your argument) into BlogPost. You need to do so because obj is an Object and you need a BlogPost.
So you write "(BlogPost) obj" and tell the comiler that "blog" is not an object anymore but a BlogPost. This all crazy stuff is made for one purpose ... to use the getTitle() method.
And this is your second question. The getTitle method is inside the BlogPost class. So you need a BlogPost object = same as instance to access the getTitle method.
The Object "obj" that was your argument of the getTitleFromObject() method can´t access methods in BlogPost class. And this is the reason we need to cast it into BlogPost using "blog = (BlogPost) obj"
BlogPost blog = new BlogPost;
// making an instance
// we don´t need to make it in this challenge
Is not the same as
blog = (BlogPost) obj;
// converting Object "obj" into a BlogPost
Don´t hesitate to ask if something is not clear, the topic is not easy to understand
Grigorij

jinhwa yoo
10,042 Pointsinstanceof String ---> what does this mean???
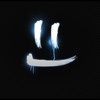
Grigorij Schleifer
10,365 PointsThis line is comparing the type of the argument with a String type. If you give a String as argument to the getTitleFromObject like this:
String argument = "bla bla bla";
public static String getTitleFromObject(//here you give a String "argument" as argument) {
if (string instanceof String) {
// is my argument a String or not?
// code
}
If your argument is a String you need to convert "obj" into String. Because the method takes an Object and not a String explicitly ...
jinhwa yoo
10,042 Pointsjinhwa yoo
10,042 PointsWhat is instance??????
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsInstance is the same as object.
If you create a class somewhere like a BlogPost class, where you define methods like getTitle() and so on. You need to create an object of that BlogPost class to be able to use that methods. We call this "object creation" instantiation. Or making an instance of a class. Making an object is the same but in other words ...
So instantiation look like this:
Then you can call a method from the BlogPost class using the name of the instance "blog" and the dot (.) operator to do this:
Does it make sense?
Grigorij