Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial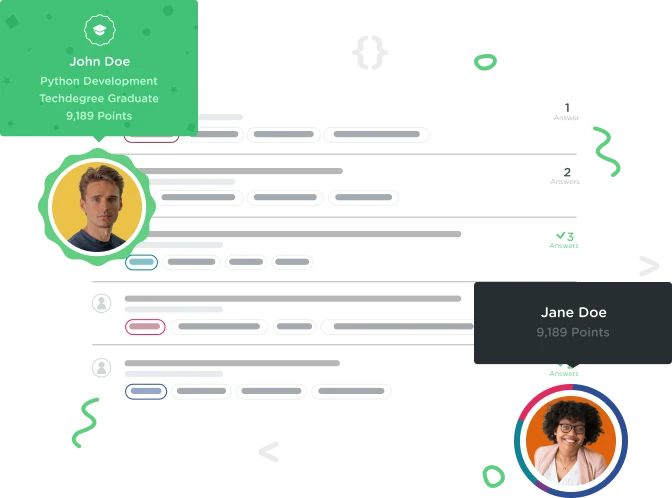
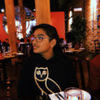
Haardik Gupta
Full Stack JavaScript Techdegree Student 5,459 Pointshelp js
Next, change the color of each child paragraph to blue.
(Remember: paragraphs is a collection of elements, so you'll first need to use a loop to access each element in the collection.)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ is the question my code is getting an error
var section = document.querySelector('section');
var paragraphs = section.children;
for (let i = 0; i < paragraphs.length; i += 1) {
color = "blue"(paragraphs[i]);
}
<!DOCTYPE html>
<html>
<head>
<title>Child Traversal</title>
</head>
<body>
<section>
<p>This is the first paragraph</p>
<p>This is a slightly longer, second paragraph</p>
<p>Shorter, last paragraph</p>
</section>
<footer>
<p>© 2019</p>
</footer>
<script src="app.js"></script>
</body>
</html>
3 Answers
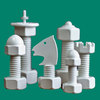
Steven Parker
240,995 PointsYour loop is good, but the assignment syntax needs some work:
- the code to access the paragraph needs to be on the left side of the assignment.
- the properties should be accessed on the paragraph element
- only the color itself should be on the right side
- "color" is a sub-property of the "style" property
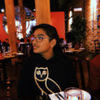
Haardik Gupta
Full Stack JavaScript Techdegree Student 5,459 Pointsi dont understand what you mean by left side and right side
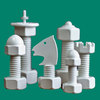
Steven Parker
240,995 PointsIn an assigment, the "=" is the assignment operator, the left side of it is where you put the thing that is being assigned, and the right side is where you put the value being given to it.
ā this is the left side ā = ā
this is the right side
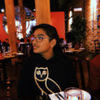
Haardik Gupta
Full Stack JavaScript Techdegree Student 5,459 PointsIs this the index (paragraph[i]); ?
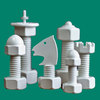
Steven Parker
240,995 PointsThe index is the value in brackets
[i]
Haardik Gupta
Full Stack JavaScript Techdegree Student 5,459 PointsHaardik Gupta
Full Stack JavaScript Techdegree Student 5,459 Pointsi updated the code to
var section = document.querySelector('section'); var paragraphs = section.children; for (let i = 0; i < paragraphs.length; i += 1) { paragraphs.style.color = "blue"(paragraphs[i]); }
and im geting the error
Bummer: Cannot read property '1' of null
Steven Parker
240,995 PointsSteven Parker
240,995 PointsYou're missing the index on the left side of the assignment. And there should be nothing on the right side except for "blue".