Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial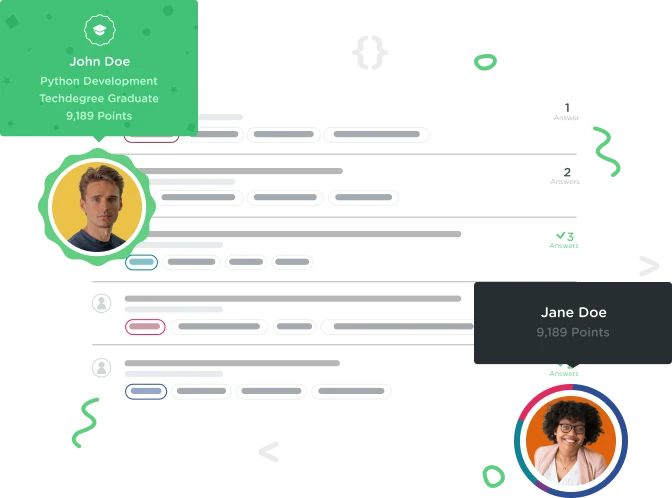

Ante Petrović
Front End Web Development Techdegree Graduate 28,940 PointsHave problem with this challenge:
Challenge Task 4 of 4
You're on fire! Last one and it is, of course, the hardest. Make a function named reverse_evens that accepts a single iterable as an argument. Return every item in the iterable with an even index...in reverse. For example, with [1, 2, 3, 4, 5] as the input, the function would return [5, 3, 1]. You can do it!
this is my code and it doesnt pass, why? def odds(arg): return arg[::-2]
def first_4(itera):
return itera[:4]
def first_and_last_4(n):
return n[:4] + n[-4:]
def odds(index):
return index[1::2]
def reverse_evens(index):
return index[::-2]
24 Answers
William Li
Courses Plus Student 26,868 PointsUpdated May 28th, 2018
Hmmmm, for some reason, this particular post remains pretty active despite being 1 & 1/2 year old, (thanks to Google maybe?), new comments are posted every once in a while, either claiming their solutions to be correct, or calling for Kenneth to fix the buggy grader.
I guess it's necessary to update my post with some clarification for any student who will be reading this forum post in the future.
Make a function named reverse_evens that accepts a single iterable as an argument. Return every item in the iterable with an even index...in reverse. For example, with [1, 2, 3, 4, 5] as the input, the function would return [5, 3, 1]. You can do it!
What this challenge wants you to do is:
- pick out all the elements in the even index
- then sort those elements in reverse order.
I'm aware that at this point of the course, most students probably haven't been exposed to Python code testing yet. But let me say a couple things about testing the code correction anyway.
reverse_evens([1, 2, 3, 4, 5]) # => expected [5, 3, 1]
Many students are under the impression that if their code outputs the matching result as given in the example from the Code challenge description, it'd have enough to pass the grader. Unfortunately, that's not the case. Because everytime the Check Work button is clicked, the grader will check your code against several behind-the-scene test cases for correction, it needs to pass all of 'em to pass the code challenge.
Judging by the comments, my_list[-1::-2] or my_list[::-2]
seems to be the very popular solution here. It works for [1, 2, 3, 4, 5], whose length is odd-number; but this solution would completely fail when passing in even-number length list as argument, as it returns all the wrong elements.
Here. I wrote a unittest to help test your reverse_evens
method so that you'll get little more feedback than simply Bummer: Didn't get the right values from reverse_evens
.
import unittest
def reverse_evens(my_list):
# fill in your implementation below.
return
class TestReverseEvensMethod(unittest.TestCase):
def test_empty_list(self):
self.assertEqual(reverse_evens([]), [])
def test_single_element_list(self):
self.assertEqual(reverse_evens([0]), [0])
def test_odd_length_list(self):
self.assertEqual(reverse_evens([1, 2, 3, 4, 5]), [5, 3, 1])
def test_even_length_list(self):
self.assertEqual(reverse_evens([1, 2, 3, 4, 5, 6]), [5, 3, 1])
if __name__ == '__main__':
unittest.main()
A more extensive testsuit could've been written, but I think 4 test cases should suffice here.
If you haven't had Python setup on your local machine, head over to CodeingGround Python3-Online https://www.tutorialspoint.com/execute_python3_online.php, copy/paste the unittest code over there and click Execute.
Screenshot shows 4 failures, that's because I haven't implemented the reverse_evens
method body yet.
So complete the reverse_evens
method with your own implementation, and run the unittest code to see what output you're getting. If it's NOT showing 0 failures, that means your code needs some more debugging in order to pass the challenge (and the grader is not buggy).
One answer brings up the term "anti-Pythonic"? Please don't get your priority wrong here. It's nice to write beautiful, Pythonic, maintainable, clean code; extra-nice if the code is optimized for efficiency. But before any of that, the first and foremost thing you should think about is making sure the code is well-tested for correctness.
William Li
Courses Plus Student 26,868 PointsThe problem here is that your method implementation doesn't work on all cases.
def reverse_evens(my_list):
return my_list[::2][::-1]
[::2]
is to pick out all the even index elements from the iteralbe; then use [::-1
to reverse the elements.

iworld
4,125 PointsSo, in that case, I think the question should be different. Instead of just asking returning index...in reverse, also include reversing the final result.

Peter Rzepka
4,118 PointsQuoted by iworld: "So, in that case, I think the question should be different. Instead of just asking returning index...in reverse, also include reversing the final result."
There are ALOT of quiz questions that are improperly phrased or missing information about how they want the result.
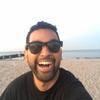
Anthony Albertorio
22,624 PointsIsn't the result the same as using iterable[::-2] as iterable[::2][::-1]
Mehran Moradi
11,140 PointsDear William
I Think [::-2] should work. Why Treehouse cannot interpret that????

Obaa Cobby
9,337 PointsWhy can you simply do this? numbs = [1, 2, 3, 4, 5] numbs[::-2]

Ronald Tse
5,798 PointsYou have to consider the length of your string
list = [0, 1, 2, 3, 4, 5]
If I use your code, I will get [5, 3, 1], but what I want is actually [4, 2, 0] (even index)
Therefore, you have to separate your code into 2 using if.
if len(list)% 2 == 0:
<return what?>
else:
<return what?>
Try it first!! If you have any problem feel free to find me. Good Luck!!

Ben Herro
Full Stack JavaScript Techdegree Student 8,722 PointsThat's for returning even numbers in the list. The even index function on list = [0, 1, 2, 3, 4, 5] should return [5, 3, 1], based on what the question is asking. I'm assuming the question has been asked incorrectly?

Michael Moore
7,121 Pointsi was wondering about that since they didn't give us a predefined list. I was trying this method but I wasn't sure it was taking it as an acceptable answer. Turns out i had my returns in the wrong order.
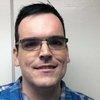
Andrew Calhoun
19,360 PointsThe fact you need to use
return list[::2][::-1]
to pass that challenge is ridiculous. It's anti-Pythonic, and creates additional work that would be obtuse to novitiates or people who are not overly familiar with Python.
My solution, which is vastly more elegant (and is reflected in other comments here) is preferable and considered more ideal syntactically.
return list[::-2]
or
return list[-1::-2]
both return the same result. Why can it not be used?
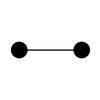
Philip Vaarskov
2,252 PointsI have also used the return list[-1::-2], and that didn't work for me either, that is very strange because it is misleading you in your understanding of python.

Mark Ganhao
18,334 PointsThe issue with your code is that the -1 (or last) index in a list is not always an even number index. For example: In a list of [0, 1, 2, 3], the -1 is 3. Therefore your code returns [3, 1]. When in fact you are looking for [2, 0].
return list[::2][::-1] first splices the list based on even indexes, and the second splice [::-1] reverses the list.
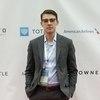
Kirill Kachinsky
15,254 Pointswhy is it ridiculous? [::2] handles the even range and [::-1] handles the reverse countdown for that range.

Joshua Hall
1,204 Pointsdef reverse_evens(given_list):
return given_list[::2][::-1]
This code as some people have used here DOES complete the challenge. It is what I had to use to complete the challenge, but it does not actually fulfill the conditions that the instructions seem to require.
Since there is no list given, the code should theoretically be written such that in any given list, reverse_evens could be used to return, in reverse order, only the even numbers from that list. Using this code completes the challenge but doesn't work as a catch all for any list, only lists that start and maybe end with an even number. If given_list = [1, 2, 3, 4, 5, 6, 7, 8, 9] what happens is this code will break that list down into every other number, and then return the ones left in reverse order. Essentially, the list breaks down into [1, 3, 5, 7, 9], and then reverses into [9, 7, 5, 3, 1].
This challenge either needs to be modified, or more needs to be taught on the use of the modulo '% 2' in order to use only the even numbers in a list like the one above.

tomaszfurmanczyk
Full Stack JavaScript Techdegree Student 18,242 PointsThis is an accurate solution that works fine in the shell and using any interpreter except the built in interpreter from treehouse:
def reverse_evens(items): return items[::-2] #reversing order and providing all the even indexes
result: [15, 13, 11, 9, 7, 5, 3, 1]
I believe there is a bug with python collections > slice functions> Challenge Task 4 of 4

Carlos Rivero
1,403 Pointsme too in the compiler my code functions fine but the challenge task won't allow me to run it : word =[1,2,3,4,5,6,7,8,9,10,11] def first_4(word): return word[ : 4]
def first_and_last_4(word): return(word[ :4] + word[-4: ])
def odds(word): return(word[ : :2])
def reverse_evens(word): return(word[-1 : :-2])

afdaaf sdfsdf
Python Web Development Techdegree Student 143 PointsSame problem

tomaszfurmanczyk
Full Stack JavaScript Techdegree Student 18,242 PointsTHIS WAS MY PREVIOUS COMMENT:
This is an accurate solution that works fine in the shell and using any interpreter except the built in interpreter from treehouse:
def reverse_evens(items): return items[::-2] #reversing order and providing all the even indexes
result: [15, 13, 11, 9, 7, 5, 3, 1]
I believe there is a bug with python collections > slice functions> Challenge Task 4 of 4
However for the built in interpreter on treehouse it is only accepting
TRY THIS AND YOU WILL PASS:
def reverse_evens(items): return items[::2][::-1]

Paul Simon
202 PointsAs Tomasz said, there is some issue with treehourse interpreter. The following code works fine in python interpreter for 4th challenge:
def reverse_evens(items):
return items[::-2]
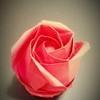
Lynn Zheng
3,569 PointsI tried to write like this, but it is wrong, why? def reverse_evens(ite): ite_new=ite[::-1] ite_new2=ite_new[::2] return ite_new2

Shravya Enugala
9,663 PointsHow is it different from my approach:
def rev_even(e):
return e[::-1][::2]
How does it make a difference from:
def rev_even(e):
return e[::2][::-1]
Aren't they both return same solution?

Mathew V L
2,910 Pointswe never been taught
def reverse_evens(iterable): return iterable[::2][::-1]
aiyaaaaaaaaa

Radovan Valachovic
Python Web Development Techdegree Graduate 20,368 PointsWhy this answer is not correct?
def reverse_evens(item_list):
return item_list[::-2]
list = [1, 2, 3, 4, 5]
print(reverse_evens(list))
My Python interpreter in PyCharm made it work correctly, even in my mac terminal

yuva k
4,410 Pointsdef reverse_evens(item_list): item = item_list[0::2] return item[::-1]
list = [1, 2, 3, 4, 5] print(reverse_evens(list))

yuva k
4,410 Points"" def reverse_evens(item_list): item = item_list[0::2] return item[::-1]
list = [1, 2, 3, 4, 5] print(reverse_evens(list)) ""

Elanas Bartulis
2,422 Pointshow is this:
-> return items[::2][::-1]
different from this? :
-> return items[-2:-9999:-1]

John Napier
14,383 PointsThough long, this one works in Jupyter but not in the treehouse interpretor:
def reverse_evens(iterable):
L=[]
for x in range(0,len(iterable)):
if (x % 2) ==0:
L.append(iterable[x])
L.sort(reverse=True)
return L
The above is not much different than the answer that worked for me for the previous challenge:
def odds(iterable):
L=[]
for x in range(0,len(iterable)):
if (x % 2) ==1:
L.append(iterable[x])
return L

Tiffany Wong
2,704 PointsThe instruction is very confusing. Thank you Andrew Calhoun. Your suggestion works! return list[::2][::-1]

Cathie Dunklee-Donnell
22,083 Pointsreturn my_list[::2][::-1]
I think what makes this task most confusing is that this idea of being able to slice a list twice like this has never been touched on up to this point. The syntax looks weird, almost like we're tying to splice a multi-dimensional list?? weird, right?
but I guess this makes enough sense and should pass:
my_list = my_list[::2]
my_list = my_list[::-1]
return my_list
But I'm really confused as to why this does not pass (anymore?):
my_list = my_list[::2]
my_list.reverse()
return my_list
It seems like the most obvious solution to the instructions and yet the feedback when you fail is not at all helpful.

Chad Goldsworthy
4,209 PointsI used this code below and it worked perfectly in a text editor/terminal, but not accepted by this challenge??
def reverse_even(iterable):
if len(iterable) % 2 == 0:
return iterable[-2::-2]
if len(iterable) % 2 == 1:
return iterable[-1::-2]

Chad Goldsworthy
4,209 PointsUsed this code below, producing the exact same result, and it worked, if anyone is still battling :
def reverse_even(iterable):
evens = iterable[::2]
return evens[-1::-1]

Antonio De Rose
20,885 Pointsactually this is working for me with the python interpreter, not with the question workspace in treehouse.
def reverse_evens(iter):
iter.sort(reverse=True)
return iter[0::2]

periz28
5,359 PointsWow. This one was tough. After realizing the length of the list would affect the outcome I came up with this solution:
def reverse_evens(iterable_list2): if len(iterable_list2) % 2== 0: new_list = iterable_list2[::2] else: new_list = iterable_list2[0:len(iterable_list2):2]
return(new_list[::-1])
print(reverse_evens(['1','2','3','4','5','6','7','8','9','10','11','12']))
Note: it prints the even index, not the even numbers. In my print example the even index numbers are odd numbers in the list
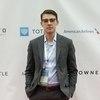
Kirill Kachinsky
15,254 PointsThis is what I have, and the third function worked fine until the 4th challenge, now the 3rd function no longer works for this challenge, so weird.
def first_4(iterable): return iterable[0:4]
def first_and_last_4(iterable): return iterable[:4] + iterable[-4:]
def odds(iterable): return iterable[0::2]
def reverse_evens(iterable): return iterable[4::-2]

Ante Petrović
Front End Web Development Techdegree Graduate 28,940 PointsThis one need to be done with slices. My code is good when i use it in jupyter, but here doesnt work.
n = list(range(6)) def reverse_evens(n): return n[::-2] print(reverse_evens(n))
and result is [5, 3, 1]

Ronald Tse
5,798 PointsYes, You should complete my version using slices in the return part.
Yours version should not be able to work when you have odd number items in your list.

yuva k
4,410 PointsHI Here is my answer
def reverse_evens(item_list):
item = item_list[0::2]
return item[::-1]
list = [1, 2, 3, 4, 5]
print(reverse_evens(list))
Ali AlRabeah
909 PointsAli AlRabeah
909 PointsI appreciate you taking the time to clarify it some more. It makes sense now.
Vishay Bhatia
10,185 PointsVishay Bhatia
10,185 PointsThanks for this William Li, however please could you double check the tests you have written. I believe you wrote one o the tests to give out the reverse_odds as the answers. For example:
if the list is [1,2,3,4,5,6], the correct answer should be [6,4,2], which I am achieving with my code below, however in your test it failed.
def reverse_evens(my_list): return my_list[-1::-2]
Vishay Bhatia
10,185 PointsVishay Bhatia
10,185 PointsSo I think I figured it out! Plus it passed all of William Li tests as well (although why the answer for the list [1,2,3,4,5,6] is [5,3,1] I still don't know)
def reverse_evens(item): even = item[0::2] even.reverse() return even
I am first creating a variable called even which works as normal picking every 2nd number. Then I am using the reverse method on it to flip the numbers around. This passed! Only took me 3 days to figure it out!! (being sarcastic)
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 PointsHi, Vishay Bhatia Good job on figuring out the correct answer for this problem
As to your question on why reverse_evens([1,2,3,4,5,6]) returns [5,3,1]. You can think of it this way.
What reverse_evens method should do is pick out all the elements in even index positions. For this particular list, that would be index 0th, 2nd, & 4th, ends up with the list [1, 3, 5], then reverse this list in place you get [5,3,1].
Hope that makes sense.
Himanshu Verma
5,075 PointsHimanshu Verma
5,075 PointsThanks for the detailed explanation. It makes sense and worked for me :)
Sam Tarnovsky
2,526 PointsSam Tarnovsky
2,526 PointsThis code passes the unittest but fails the grader:
def reverse_evens(my_list):