Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial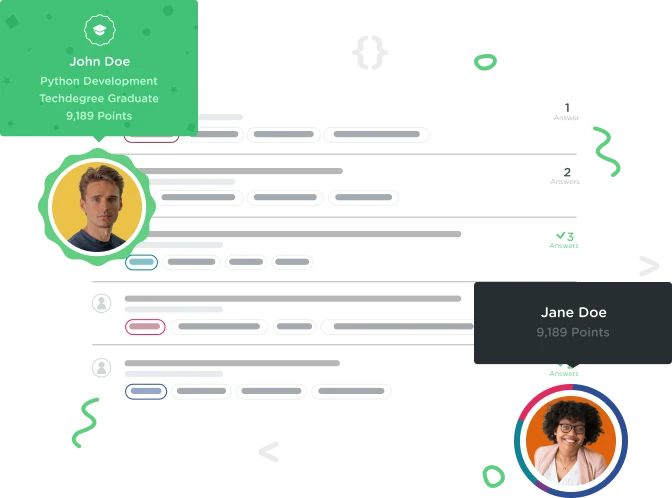

Kittipol Munkatitum
13,074 Pointsgot stuck with challenge
Hi,I can not pass this challenge when I write my code and check my work, treehouse always told that have problem about communication and this is my code
extension String{
func add(number: Int) -> Int {
if let unwrapped:Int = Int(self) ?? 0{
return unwrapped + number
}
}
}
could you help me solve this challenge thanks
3 Answers
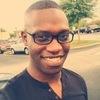
Jason Cornwall
9,645 PointsYou were definitely on the right track. This one took me a while to figure out. Since the string that we are trying to add a number to might or might not have a number inside of it, we aren't actually returning and Int but an Optional of type Int. We also have to remember to return nil if the string doesn't have an integer inside of it. This is how I did it.
extension String {
func add(num: Int) -> Int? {
if let value = Int(self) {
return value + num
} else {
return nil
}
}
}
I hope that helps. Good luck!
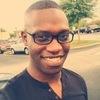
Jason Cornwall
9,645 PointsSure Farhan Hussain . We are being asked to add an extension to the String type but return a value of type Int. It's impossible to do computations with 2 values of type Int and String. So the way I decided to do this was by converting the string that we're adding the method to into an integer. That is, if I have something like this:
"1"
I convert it into an integer by doing this:
Int("1") == 1
Once I have done that, I can now use it for calculations in my method and return an integer. The
Int(self)
part just means that the conversion gets applied to whatever string we happen to be running the method on at the moment. I hope that cleared things up a bit.

Farhan Hussain
13,928 PointsThank you it helped a lot.
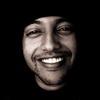
miikis
44,957 PointsHey guys,
Jason's answer works just fine but I thought I'd add that this is the perfect time to use guard because casting a String into an Int β via Int("someString") β returns an optional. So below is a more semantic way to do the deed. Feel free to ask for clarification, if you need it :)
extension String {
func add(val: Int) -> Int? {
guard let this = Int(self) else {
return nil
}
return this + val
}
}
"1".add(val: 2) // returns 3 as an Int
"two".add(val: 3) // returns nil
Kittipol Munkatitum
13,074 PointsKittipol Munkatitum
13,074 Pointsthxx you jason about your answer
Farhan Hussain
13,928 PointsFarhan Hussain
13,928 PointsCan you explain your code? I did not understand the part "Int(self)". I'm also stuck on this problem. Thank you.