Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial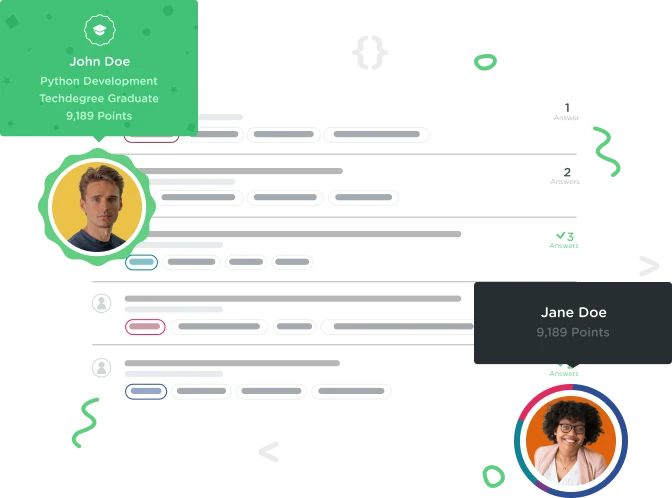

Ketaki Kshetramade
1,661 PointsGetting msg "Hmmm, I entered "Nelson" for the lastName and expected line number 2 to be returned, but instead it was 50"
For the code I've coded for this task I am getting Bummer msg "Hmmm, I entered "Nelson" for the lastName and expected line number 2 to be returned, but instead it was 50".Please help figure out whats wrong in my code.
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
char firstLetter = lastName.charAt(0);
if(firstLetter>='A' && firstLetter <='M'){
lineNumber = '1';
}else{
lineNumber = '2';
}
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
1 Answer
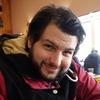
Eric M
11,546 PointsHi Ketaki,
Your solution is very close.
You might already know this, but the reason we can use greater than and less than against characters is that they are encoded as a numerical value, they can be converted between the character and numeric representation. Or, in more specific programming terms, you can cast between a char
and an int
and back.
An ASCII value of 50 is the character 2.
In Java string literals are enclosed in double quotation marks (e.g. String example_string = "hello"
).
Character literals are enclosed in single quotation marks (e.g. char example_char = 'q'
or char example_two = '2'
).
Integers are not enclosed at all (e.g. int my_int = 3
).
If you remove the single quotes around your assignment to lineNumber
in your conditional branches your code passes the challenge.
Happy coding!