Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial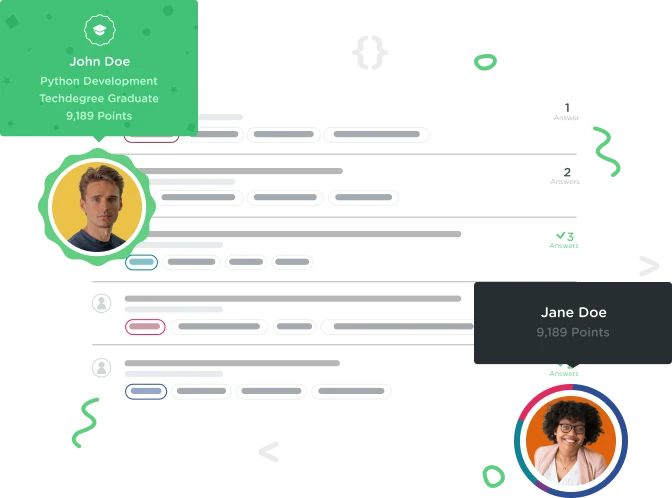

Thomas Grow
3,238 PointsGetting "anonymous function" error
This is my solution:
var message = '';
var student;
var searchFor = '';
var searchForIndex;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
console.log ("In program");
do {
console.log ("In loop");
searchFor = prompt('What student? ');
searchForIndex = students.indexOf('searchFor');
student = students[searchForIndex];
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
print(message);
} while (searchFor.toUpperCase !== 'QUIT');
When I run this, I get the following error: Uncaught TypeError: Cannot read property 'name' of undefinedstudent_report.js:22 (anonymous function)
Can someone help me figure out what is happening please?
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Thomas,
I haven't tested this out but your problem should be in the middle line of code here:
searchFor = prompt('What student? ');
searchForIndex = students.indexOf('searchFor'); // You wrapped searchFor in quotes making it a literal string
student = students[searchForIndex];
So it's looking literally for the name 'searchFor'. This won't be found and will return a -1.
Then you're doing student = students[-1]
That makes student undefined
because it's an invalid array index. Which leads you to the error.
With student.name
you're trying to access the name property of an undefined value.
If you remove the quotes then you'll be passing in whatever string is stored in the variable searchFor
So when you get errors like this you sometimes have to work backwards from the line number that is reported to figure out which piece of code is actually producing the undefined value.

morgan segura
Courses Plus Student 6,934 PointsYou have student defined, however where are you getting the data inside of student? "var student;" the variable student needs data.
Thomas Grow
3,238 PointsThomas Grow
3,238 PointsThanks, but I'm confused... wouldn't the response from my prompt get assigned to searchFor?
Oh wait... I think I see what you're saying. I'm searching for a string of 'searchFor' instead of its value. I'll change it and see if it helps.
Thomas Grow
3,238 PointsThomas Grow
3,238 PointsOk, so I see where I'm getting -1 for my searchFor value when I place it in my indexof line. But I don't understand why... the line before, I do
console.log('searchFor = ' + searchFor);
which gives me a correct string, such as Dave...
But when I follow my indexof line with
console.log('searchForIndex = ' + searchForIndex);
it returns with -1.
What am I missing. What do I need to do going from searchFor to searchForIndex? Is this line wrong?
searchForIndex = students.indexOf(searchFor);
I understand that this line is trying to look for the literal string "searchFor"... but how do I tell Javascript that this is a variable and to read the value of the variable instead of seeing it as a literal string?
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsI'm sorry Thomas, I should have reviewed this video.
When I saw your error with quotes around the variable name I completely missed the fact that the indexOf method isn't the right type of code to use for the type of data you have.
searchForIndex = students.indexOf(searchFor);
That line would be good if
students
was an array of names. Then it would look through each element to find a matching name. However,students
is an array of objects where each one has aname
property.So you have to think about how you can get to that name. Example for Dave's name:
students[0].name
What you can do is replace that line of code with a loop that will go through each object and access the
name
property of that object and see if it matches what's being search for.Something like this inside the loop: