Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial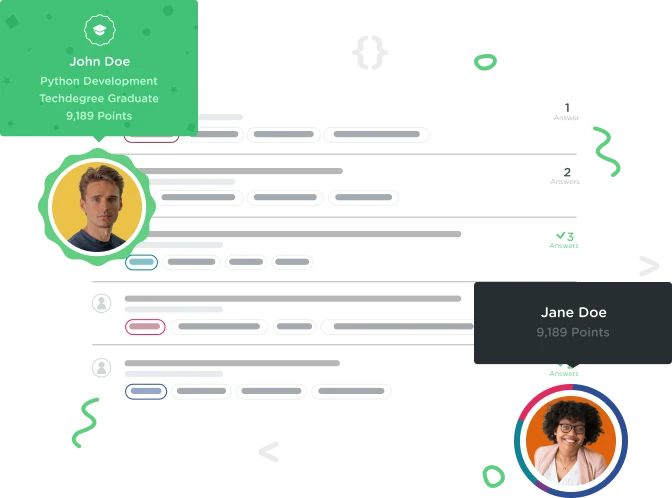

Jonathan Hector
5,225 PointsFunny enough you can always override a constant if you don't assign a value to it.
let exampleBool : Bool
let number1 : Int = 1
let number2 : Int = 2
if(number1 > number2){
exampleBool = false
} else {
exampleBool = true
} //should always be false but you can play around with it.
print(exampleBool)
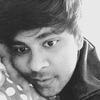
SivaKumar Kataru
2,386 PointsWell its not over riding a CONSTANT value . You created a constant( exampleBool ) without initializing any value to it .When you declare a constant/variable without initializing any value to it , then the variable/constant contains garbage values . And in the IF ELSE control flow , either IF or ELSE block may be executed . So therefore the uninitialized constant may be initialized with the value of true/false based on the evaluation of IF ELSE block only once.
2 Answers
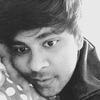
SivaKumar Kataru
2,386 PointsTo, Stephen Emery ........................................ let exampleBool: Bool let firstValue:Int = 1 let secondValue:Int = 2 if(firstValue > secondValue){ // This Line Won't Be executed at all . exampleBool = false } if (firstValue < secondValue){ // This Line will be executed . Therefor Let constant will be assigned with True . And Now it is Locked .so that you cant change further forever exampleBool = true }

Jabes Pauya
Courses Plus Student 2,432 Pointsfor the first place the first constant has no assigned value let exampleBool : Bool = ? has no assigned value yet
until such time you added a condition to check the values of number1 and number2 if the condition satisfied either condition that is the time when your constant exampleBool will have assigned value (true/false)
as we studied in basic iOS, the variable is the only thing you can override the value like :
var String greeting = "Hello Linda"; greeting = "You forgot your glasses" - in this case the value has been override
Stephen Emery
14,384 PointsStephen Emery
14,384 PointsIt's not technically overriding a constant if you leave it unassigned/unitialized. You create an uninitialized constant at the start. Then you assign a value to it with your if statement. Since it's an if/else statement, only one of two possibilities will ever happen. You don't get an compiler error, because only 1 assignment could ever happen. If you write the code like I did below, you will get a compiler error.
let exampleBool : Bool let number1 : Int = 1 let number2 : Int = 2
if(number1 > number2){ exampleBool = false } if(number1 < number2) { exampleBool = true //Immutable value 'exampleBool' may only be initialized once }