Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial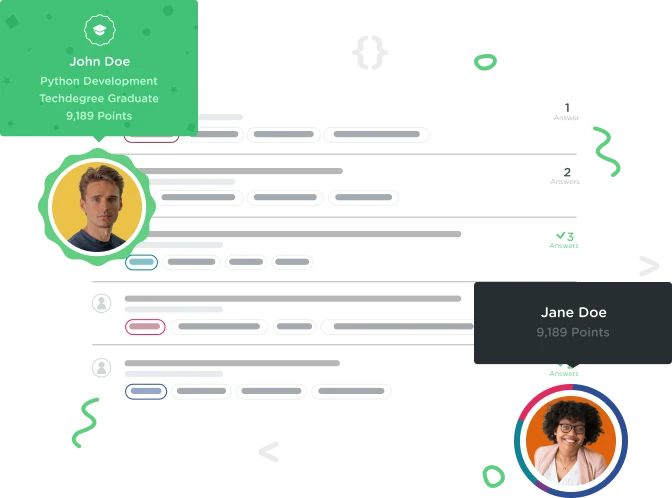

dakota ferrell
2,336 Pointsfunctions and structs
I am not sure what the difference between functions and structs because it seems like there written almost the same way and they have arguments in them like functions?
1 Answer

Sahil Gangele
6,851 PointsBasics
- A struct is something
- A function does something. You give it some parameters, and based on that it returns something, or performs some action
So a struct represents something. So, say you're trying to create a graph. Well, with a graph you have to create points (x,y). How would you do that? Or, really you should ask, "How would I represent this?" Answer: Struct
struct Point {
var x: Int
var y: Int
}
Now, can you do this with functions?
func point(x: Int, y: Int) {
// What to do in here?
// Well the point of a function is to do something, so it doesn't apply here.
// We're not trying to DO anything. We just want to represent something
// See, it's hard to represent even something as simple as a point with functions
}
It makes sense why this doesn't work, and to show this let's do a task: Create a variable and print the x value
(Using our previous definition of struct Point
)
// This calls the structs' implied initializer method. It creates a struct.
// Yes it looks like a function call, but that's with all initializers.
var origin = Point(x: 0, y: 0)
print(origin.x)
Now let's try to do this with a function
(Using our previous definition of func point
var origin = point(x: 0, y:0) // Okay, they look identical so far.....
print(origin.x) // ERROR
// See, there is no .x associated with this because a function doesn't have properties. Structs do!
// This really doesn't make sense. This function doesn't even return anything, so the first line will be an error as well.
So now we see where structs thrive..... What about functions?
Well, let's stick to our point
example. What if we want to calculate the slope between two points?
Now look at that sentence.... I used a verb calculate. This DOES something. So a function will be used here:
func slope(point1: Point, point2: Point) -> Int {
let x1 = point1.x
let y1 = point1.y
let x2 = point2.x
let y2 = point2.y
return (y2 - y1) / (x2 - x1)
}
This makes sense, and is very easy to do. Can we do this with structs!?
Well, you shouldn't! When you're trying to DO something, use functions. Calculating the slope is doing something.