Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial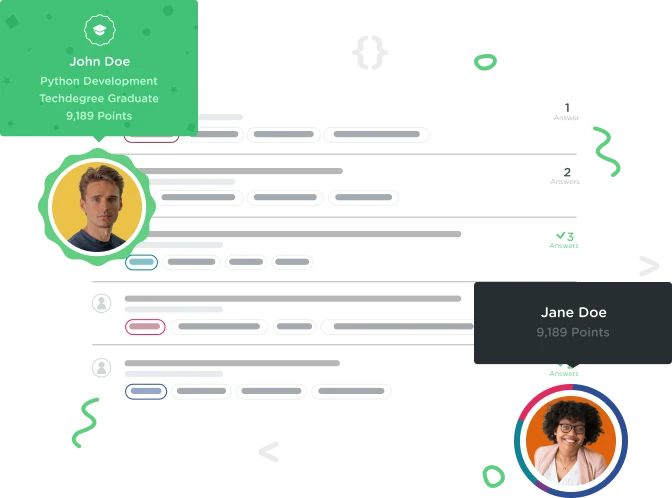

Yassin Sassi
3,571 PointsFrog can't catch new flies, only flies I placed myself in the hierarchy window.
Here is the code:
using UnityEngine;
using System.Collections;
public class FlySpawner : MonoBehaviour {
[SerializeField]
private GameObject flyPrefab;
[SerializeField]
private int totalFlyMinimum = 12;
private float spawnArea = 25f;
public static int totalFlies;
// Use this for initialization
void Start () {
totalFlies = 0;
}
// Update is called once per frame
void Update () {
//While the total number of flies is less than the minimum...
while (totalFlies < totalFlyMinimum)
{
//... then increment the total number of flies
totalFlies++;
// ... create a random position for the fly
float positionX = Random.Range(-spawnArea, spawnArea);
float positionZ = Random.Range(-spawnArea, spawnArea);
Vector3 flyPosition = new Vector3(positionX, 1.5f, positionZ);
Instantiate(flyPrefab, flyPosition,Quaternion.identity);
}
}
}

Yassin Sassi
3,571 PointsHey Justin thanks for helping again,
I did try that already but it didn't work. I added the fly prefab to the GameManager. I even tried to increase the radius of the sphere collider just to be sure. So far I had no luck.
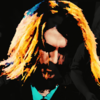
Justin Horner
Treehouse Guest TeacherOkay I'd be happy to look further into it if you could provide the code on GitHub or zipped somewhere I can download it.

Yassin Sassi
3,571 Pointshttps://www.dropbox.com/s/8imly8smpvjihzc/Frogs%20and%20Logs.rar?dl=0 here you go
And thank you very much!
1 Answer
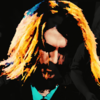
Justin Horner
Treehouse Guest TeacherHello Yassin,
Thanks for providing access to the project. I've had a few minutes to look at it and here's what I found.
The reason the generated flies are not being picked up is because they are located too high on the Y axis. This explains why the collision detection is not firing when it looks like the frog is colliding with the flies. You can visualize this by playing the game in Unity and when the frog is near a fly, press the pause button and move the camera to see how high the fly is compared to the frog.
The source of the problem is some of the prefabs in the view were not at the default position. The environment itself was set to a negative number instead of 0. The player was also set too low on Y. Once I reset the positions, the flies could be reached by the frog as expected. I also fixed an exception that was being caused by an extra FlyMovement component that didn't have the center transform set.
You can download the updated project here. Please let me know when you've had a chance to download it :)
I hope this helps.

Yassin Sassi
3,571 PointsHey Justin,
Thanks so much for helping me out! I fixed all of the positioning errors so far, but I don't quite understand the exception you are talking about. I get the UnassignedReferenceException aswell, but I don't understand where the extra FlyMovement component is.
But everything is working so far thank you again for helping me out :)
Justin Horner
Treehouse Guest TeacherJustin Horner
Treehouse Guest TeacherHello Yassin,
It sounds like maybe the changes you've made to the prefab have not been saved and applied. So when the flies are generated, they don't contain the latest changes you've made to the prefab.
I hope this helps.