Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial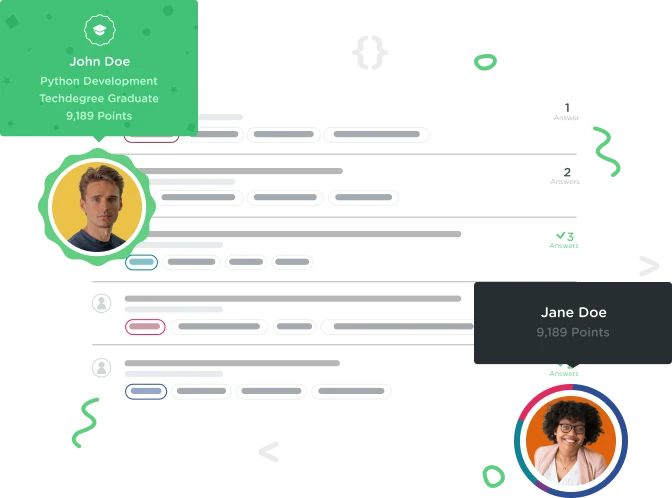

Rory Letteney
1,342 PointsFP Player Object flying out of control on start
I created a first-person view player object with a capsule, added a basic player controller and movement controls and it was working fine. Then I added a standard rigid body component to the capsule and added a basic jump feature. Now my character flies upwards as soon as I start the game. I'm sure I'm not the first person to which this has happened, but I'm not sure how to search for it. I have added my player movement code below, and the camera movement code below that. I apologize if I haven't posted correctly, this is my first post.
/////////////////////////Player Movement Code
using System.Collections; using System.Collections.Generic; using UnityEngine;
public class PlayerMove : MonoBehaviour {
private CharacterController charControl;
[SerializeField]
private float moveSpeed;
private bool isGrounded;
private Rigidbody playerRigidBody;
[SerializeField]
private float jumpHeight;
// Use this for initialization
void Start () {
charControl = GetComponent<CharacterController>();
isGrounded = true;
playerRigidBody = GetComponent<Rigidbody>();
}
// Update is called once per frame
void Update () {
MovePlayer();
if (isGrounded)
{
if (Input.GetKeyDown("Jump"))
{
playerRigidBody.velocity = new Vector3(0f, jumpHeight, 0f);
isGrounded = false;
}
}
}
void MovePlayer()
{
float horizontal = Input.GetAxisRaw("Horizontal");
float vertical = Input.GetAxisRaw("Vertical");
Vector3 moveDirectionSide = transform.right * horizontal * moveSpeed;
Vector3 moveDirectionForward = transform.forward * vertical * moveSpeed;
charControl.SimpleMove(moveDirectionSide);
charControl.SimpleMove(moveDirectionForward);
}
private void OnCollisionEnter(Collision collision)
{
collision.gameObject.CompareTag("Ground");
isGrounded = true;
}
}
/////////////////////////Camera Movement Code
using System.Collections; using System.Collections.Generic; using UnityEngine;
public class PlayerLook : MonoBehaviour {
[SerializeField]
private float mouseSensititvity;
[SerializeField]
private Transform playerBody;
float xAxisClamp = 0;
void Start()
{
//Lock the cursor in the center of the screen
//To allow the game to be minimized while also keeping the mouse...
//...locked after maximizing, this needs to be in the update method
Cursor.lockState = CursorLockMode.Locked;
}
private void Update()
{
RotateCamera();
}
void RotateCamera()
{
//Get the mouse location
float mouseX = Input.GetAxis("Mouse X");
float mouseY = Input.GetAxis("Mouse Y");
//The amount the camera should rotate
float rotateAmountX = mouseX * mouseSensititvity;
float rotateAmountY = mouseY * mouseSensititvity;
xAxisClamp -= rotateAmountY;
//Get the current rotation of the camera and player gameObject...
Vector3 targetRotationCamera = transform.rotation.eulerAngles;
Vector3 targetRotationBody = playerBody.rotation.eulerAngles;
//...add the appropriate amount the camera and body need to rotate...
targetRotationCamera.x -= rotateAmountY;
targetRotationBody.y += rotateAmountX;
//Make sure the camera doesn't flip over when looking too far down or up!
targetRotationCamera.z = 0;
if(xAxisClamp > 90)
{
xAxisClamp = 90;
targetRotationCamera.x = 90;
}
if(xAxisClamp < -90)
{
xAxisClamp = -90;
targetRotationCamera.x = 270;
}
//...and put it back into the respective transforms
transform.rotation = Quaternion.Euler(targetRotationCamera);
playerBody.rotation = Quaternion.Euler(targetRotationBody);
}
}
2 Answers
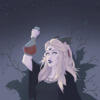
Matti Mänty
5,885 PointsAh, I don't know how I missed you using the built-in character controller. If you use Character Controller on your player object, you don't need a separate rigidbody nor a capsule collider. Character Controller should provide you with both functionalities. The skyrocketing was because your object's colliders kept on colliding with each other.
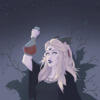
Matti Mänty
5,885 PointsAt a quick glance, I didn't notice anything out of the ordinary in the code. Sometimes rigidbodies behave strangely, but it shouldn't go up with super speed. If your capsule collider is within the ground's collider it should snap out of there and might give you a small jump though.
Try commenting out the jumping part of the code (it's the only part that gives the player velocity on the Y-axis right?) and see if the problem disappears. If so, then the Jump-key if-statement is registering as true every frame.
Also some variables were not visible in the scripts provided (inherited values?), so it's hard to say if they could cause problems.

Rory Letteney
1,342 PointsThe capsule's collider location (above, below, or within the ground collider) did not make a difference. I tried commenting out the jump function code, and as I said it worked just fine before I added any jump function code. However, once I commented it out, the strange skyrocketing still occurred. I then removed the rigid body component from the capsule and the skyrocketing stopped, but I am still left without a jump feature. I tried many different values for the serialized fields, so I am fairly certain it isn't the values being used if those were the variables you were referencing. I am still very new to Unity and C#.