Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial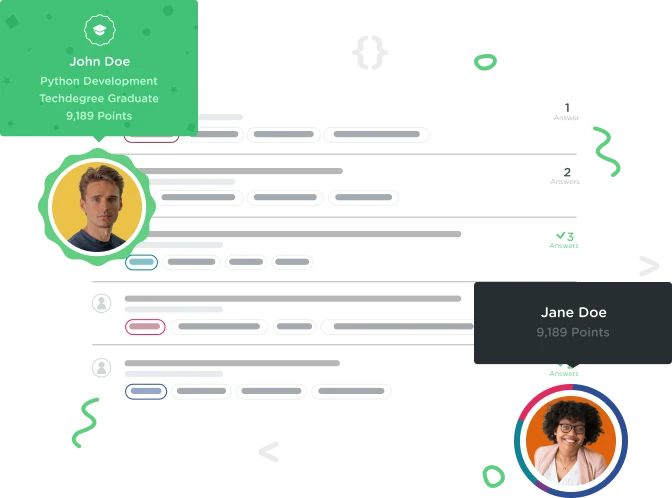
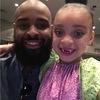
Raymond Carter, Jr.
iOS Development Techdegree Student 488 PointsFormatting Time
Formatting Time
You have been given a time value measured in seconds. Your task is to define a string literal that describes the time in hours, minutes and seconds. To achieve this, you will need to convert from the value in seconds to hours, minutes and seconds. An example of the final result we're looking for:
9700 seconds is 2 hours, 41 minutes and 40 seconds
*/
let time = 2390724
let hours = time/3600 // 664hours
let minutes = (time % 3600)/60 // 5 minutes
let seconds = time % 60 //24 seconds
"\(time) seconds is \(hours) hours,\(minutes) minutes and \(seconds) seconds"
I have a few questions that I need clarification on
- why do we put (time % 3600) in quotations? and why do we divide the that by 60? I thought we multiple that (I'm lost lol)
- why do we do time % 60?
- I don't understand how they wrote this string. please explain it to me
PLEASE HELP!!
3 Answers
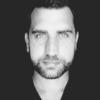
Jhoan Arango
14,575 PointsHello,
1) Why do we put (time % 3600) in quotations?
This is called precedence, what is precedence ?
Precedence is the condition of being considered more important.
let result = ( 1 x 1 ) + 5
// In this example we give precedence to ( 1 x 1 ) and then add 5 to its result.
// Meaning that the equation that is IN the parentheses needs to be solved first
// since it has precedence.
2) And why do we divide the that by 60?
So 1 hours has 3600 seconds, and we want to know the remaining of 239074 in minutes.
let time = 2390724
let hours = time / 3600 // 664hours
// If we divide ( time = 2390724 ) by 3600 seconds, it gives us 664 hours.
// the devision should return 664.09 but because we are using a whole number, or an Int
// it returns 664.
But we now need to know what is the remainder of 664.09
Once we get that remainder we want to divide that by 60 seconds which is basically converting decimals to minutes.
let minutes = (time % 3600) / 60 // 5 minutes
// So again, precedence takes over here by calculating ( time % 3600 ) which
// returns 324 then we divide it by 60 and will give us 5 minutes total
// Here we do the same using the remainder operators
let seconds = time % 60 // 24 seconds
Then the result is put into a string and using interpolation to get the values from the equations.
I hope this clears you a bit, you can read here about the remainder operator and much more about basic operators.
Good luck.
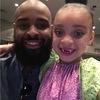
Raymond Carter, Jr.
iOS Development Techdegree Student 488 PointsCan you go into more detail about this part? "(time) seconds is (hours) hours,(minutes) minutes and (seconds) seconds"
I dont get it.... why is it saying (Hours) hours (minutes) minutes and (seconds) seconds Im lost on this. I dont know how they came up with this
and thank you for all your help
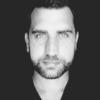
Jhoan Arango
14,575 PointsHello,
That is string interpolation.
Here is a great video that explains it to you. This is on the Swift Basics Video tutorials.
Hope you find that video helpful.
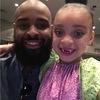
Raymond Carter, Jr.
iOS Development Techdegree Student 488 PointsI got now thank you for all your help. Can you help me understand this part now?
The code provided below defines the location of a tower and an enemy on a two dimensional coordinate map. towerX
and towerY
are the X and Y positions of the Tower while enemyX
and enemyY
are the X and Y values of the enemy.
*/
let towerX = 2.0 let towerY = 13.0
let enemyX = 4.0 let enemyY = 9.0
/*: Write code to determine how far they are from one another using the Distance Formula. The Distance Formula can be broken down into the following steps
- Subtract the distance between the X coordinates of the Tower and Enemy and square it
- Subtract the distance between the Y coordinates of the Tower and Enemy and square it
- Add the two square values
- Obtain the square root of the resulting value */
// Enter your code here let distancex = enemyX - towerX
//: For the final part, obtaining the square root, replace the 1 inside of the sqrt(1)
with the value you want to compute the square root for.
import Foundation
let root = sqrt(1)