Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial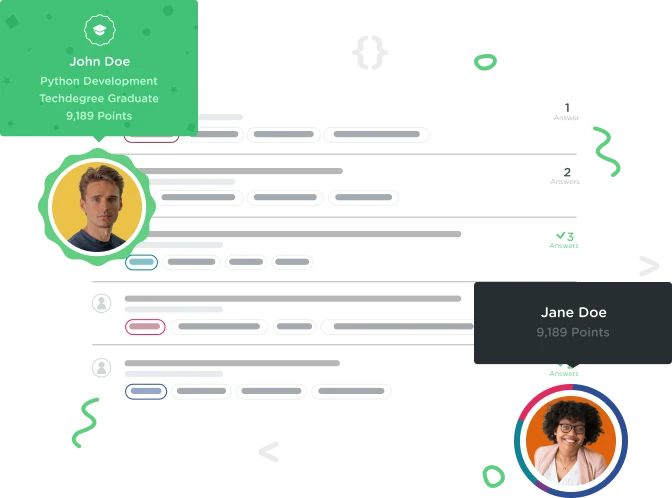

pawkan kenluecha
26,303 PointsFor this example, can I code like this ?
I've already declared a map interface at the top of this file. I don't sure why this didn't work. In my logic, this should get reference video from oldTitle and remove the old key after that they will assign a new one with a new title. The compiler keep saying " are you sure you update the title ?".
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Video video = videosByTitle(course).get(oldTitle);
videosByTitle.remove(oldTitle);
videosByTitle.put(newTitle,video);
}
2 Answers
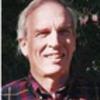
jcorum
71,830 PointsOK. I'll post the entire class, as I wasn't sure what you meant by "I've already declared a map interface at the top of this file." Note that this version doesn't need you to add a setVideo() method to the Course class (as I had suggested before), although it works, as I'm guessing the challenge would rather you did Task 1 without changing the Course class:
import com.example.model.Course;
import com.example.model.Video;
import java.util.Map;
import java.util.HashMap;
import java.util.List;
public class QuickFix {
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video newVideo = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
course.getVideos().add(1, newVideo);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Map<String, Video> videos = videosByTitle(course); //create a Map holding the videos for the course
Video video = videos.get(oldTitle); //get video that has the old title
video.setTitle(newTitle); //change the title
videos.put(newTitle, video);
}
public Map<String, Video> videosByTitle(Course course) {
Map<String, Video> videos = new HashMap<String, Video>();
List<Video> vids = course.getVideos();
for (Video v : vids) {
videos.put(v.getTitle(), v);
}
return videos;
}
}
If you want to test the code, here's a program that will do that:
import java.util.*;
public class QuickFixMain {
public static void main(String[] args) {
//create some videos
Video v1 = new Video("Star Wars");
Video v2 = new Video("Pride and Prejudice");
Video v3 = new Video("Harry Potter");
//put them in an ArrayList
List<Video> videoList = new ArrayList<>();
videoList.add(v1);
videoList.add(v2);
videoList.add(v3);
//create a Course
Course c1 = new Course("best movies", videoList);
System.out.println(c1);
QuickFix q = new QuickFix();
q.addForgottenVideo(c1);
System.out.println(c1);
q.fixVideoTitle(c1, "The Beginning Bits", "The Ending Bits");
System.out.println(c1);
}
}
This QuickFixMain program assumes you have added a toString() method to the Course class:
public String toString() {
String s = "";
s = "Course name: " + mName;
for (Video v : mVideos) {
s += "\n " + v.getTitle();
}
s += "\n";
return s;
}
Would be interested if you see a way to improve this code! As there are always different ways of doing things, and sometimes, in the heat of finishing a challenge, we may not find the best way!
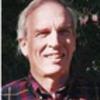
jcorum
71,830 PointsFirst, you need to add a method to Courses, so you can add videos to mVideos:
public void setVideo(int index, Video video) {
mVideos.add(index, video);
}
This method takes an index and a video and adds it to the mVideo list List<Video>. The next part is to do the TODOs in QuickFix:
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video v1 = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
course.setVideo(1, v1);
}
Here you create the video (I've given it the name v1, but you could name it something else if you wish), and then added it to the course that is passed in to the addForgottedVideo() method using the setVideo() method.
Happy coding, and good luck.

pawkan kenluecha
26,303 PointsThanks. Actually, I've passed this with another code.(Similar with your coding) I'm sorry I didn't explain it clearly. The problem here isn't the first and second example, but it's the third. My question is only focus on the third one. Please see the code below for more detail.
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Video video = videosByTitle(course).get(oldTitle);
videosByTitle.remove(oldTitle);
videosByTitle.put(newTitle,video);
I've known there is method setTitle(). I just wonder why I can't use code above instead.

Tristan Smith
3,171 PointsPawkan, I tried something similar.
Video video = videosByTitle(course).get(oldTitle);
videosByTitle(course).remove(oldTitle);
videosByTitle(course).put(newTitle,video);
I ended up using the setTitle though since it seemed like the task "wanted it". Really want to know why it wouldn't take.
pawkan kenluecha
26,303 Pointspawkan kenluecha
26,303 PointsI see. Thanks.