Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial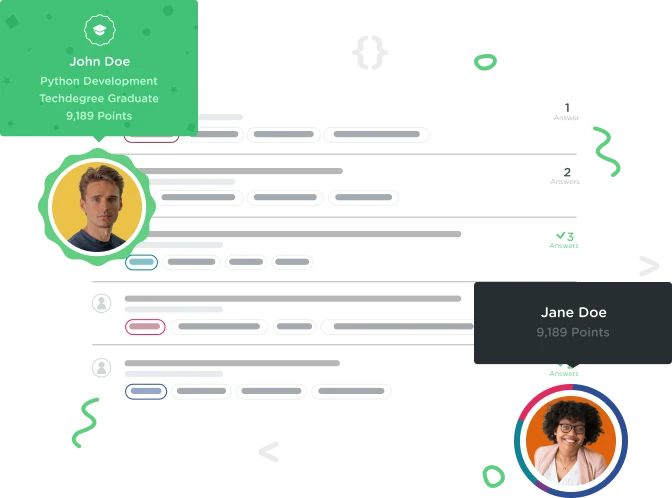

Joseph Mercado
5,846 PointsFor Loop Not working.
If I comment out the for loop, the page loads fine but the current list does not have any buttons. If I remove the comment for the for loop, the web page crashes. Here is my code:
// Changing Element Attributes
const toggleList = document.getElementById('toggleList');
const listDiv = document.querySelector('.list');
const descriptionInput = document.querySelector('input.description');
const descriptionP = document.querySelector('p.description');
const descriptionButton = document.querySelector('button.description');
const listUl = listDiv.querySelector('ul');
const addItemInput = document.querySelector('input.addItemInput');
const addItemButton = document.querySelector('button.addItemButton');
const lis = listUl.children;
// JavaScript creating Buttons
function attachListItemButtons(li) {
let up = document.createElement('button');
up.className = 'up';
up.textContent = 'Up';
li.appendChild(up);
let down = document.createElement('button');
down.className = 'down';
down.textContent = 'Down';
li.appendChild(down);
let remove = document.createElement('button');
remove.className = 'remove';
remove.textContent = 'Remove';
li.appendChild(remove);
}
for (let i = 0; i < lis.length; i += 0) {
attachListItemButtons(lis[i]);
}
//DOM Traverse parentNode and previousElementSibling and insertBefore
listUl.addEventListener('click', (event) => {
if (event.target.tagName == 'BUTTON') {
if (event.target.className == 'remove') {
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
}
if (event.target.className == 'up') {
let li = event.target.parentNode;
let prevLi = li.previousElementSibling;
let ul = li.parentNode;
if (prevLi) {
ul.insertBefore(li, prevLi);
}
}
//DOM Traverse parentNode and nextElementSibling
if (event.target.className == 'down') {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if (nextLi) {
ul.insertBefore(nextLi, li);
}
}
}
});
toggleList.addEventListener('click', () => {
if (listDiv.style.display == 'none') {
toggleList.textContent = 'Hide List';
listDiv.style.display = 'block';
} else {
toggleList.textContent = 'Show List';
listDiv.style.display = 'none';
}
});
descriptionButton.addEventListener('click', () => {
descriptionP.innerHTML = descriptionInput.value + ':';
descriptionInput.value = '';
})
// createElement example
addItemButton.addEventListener('click', () => {
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.textContent = addItemInput.value;
attachListItemButtons(li);
ul.appendChild(li);
addItemInput.value = '';
});
Where am I going wrong?
2 Answers
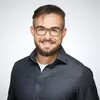
Raffael Dettling
32,999 PointsIn the for loop i +=0 "==" i=i+0 so i will stay the same you can use i++ to increase the value each time by 1 or simplie i = i+value ^^
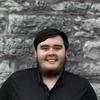
Michael Hulet
47,913 PointsThe problem is that you've created an infinite loop. When your page loads, it gets to that point and never goes past it, and the loop eats all of your computer's resources, so the browser kills the tab (or crashes, depending on the browser).
There are 3 parts to a for loop's declaration between the parentheses. The first one is run once, but any memory set in it is held on for the life of the loop. This is almost always used to set a counter variable, as you have in your loop. The second part is a conditional that is checked before the loop runs each iteration. If the condition is true
, the loop runs. If it's false
, the loop doesn't run and the code moves on past it. The last part is run after the loop finishes running each iteration. This portion is usually used to add 1 to (or "increment" in programmer terms) the counter variable that's usually set in the first portion.
You did a lot right in your declaration, but let's go through what happens.
The first time the loop runs, JavaScript creates a variable called i
and sets it to 0
. Then it checks how many items are in lis
, and checks to see if it's greater than i
. It then calls the function attachListItemButtons
and passes in lis[i]
(i
is 0
at this point). After that, it adds 0
to i
and continues onto the next iteration.
In the next iteration, it checks to see if there are still more items in lis
than the value of i
. i
is still 0 right now, and lis
isn't empty, so this is still true and the loop still runs. When it's done, it'll add 0
to i
again and start all over. The problem is that the way you've written it right now, i
will never be higher than 0
, because you're only ever adding 0
to it when the loop runs. What I believe you want to do is add 1
each time so i
will go up by 1
every time the loop runs, and it will eventually grow larger than lis.length
. A for
loop set up to increment like that looks something like this:
let exampleArray = ["This", "is", "an", "example"];
for(let i = 0; i < exampleArray.length; i += 1){ // Notice how it's i += 1 here instead of 0
console.log(exampleArray[i]);
}
That will print every word in exampleArray
to the console on a new line