Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial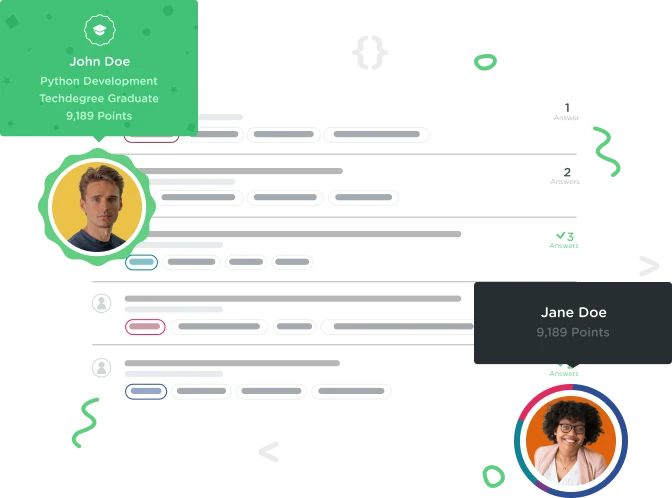

Steven Morimoto
Courses Plus Student 1,836 Pointsfor loop in Java Scrabble question
Hi, I was able to finally figure out the proper solution to this problem. But I'm still trying to figure out why char tile must be within the for loop and can't be declared above it?
ex. for(char tile : tiles.toCharArray())
I apologize if I'm asking an elementary question at this stage and appreciate the help.
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter)
{
int tileCount = 0;
for(char tile : tiles.toCharArray())
{
if(tile == letter)
{
tileCount++;
}
}
return tileCount;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
2 Answers
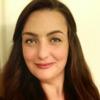
Jennifer Nordell
Treehouse TeacherHi there! It's simply how this type of for loop is done when looping through a collection or array. The char tile
is the initializer of the local variable that will only be accessible inside this for
loop. Essentially, it's a temporary variable declaration. You could do it using another method, but this is the method encouraged by Oracle.
I highly encourage you to take a look at this documentation. Scrolling all the way down you'll see the preferred method of looping through an array or collection.
Hope this helps!
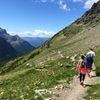
Stephen Wall
Courses Plus Student 27,294 PointsThink of the for loop as saying: "For each 'Char' that we will refer to as "tile", in the String "tiles" (watch the plural), do something. The for loop is a way of iterating through a collection by type. So maybe an easier way of saying it is, for each letter in the word "tiles"(this word is just an example) , do something. You cant create the Char outside of the loop because each time the loop repeats, it grabs the next letter in the String for you.
Say we had the String word.
String word = "word";
We could create a for loop that grabs each letter from that word one at a time and prints it on a new line.
// For each character, which we will refer to as "letter", in the String variable named "word",
// Print the letter
for (Char letter : word) {
System.out.print(letter);
}
So you see you don't need to create the char outside of the loop.
Hope this helps!