Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial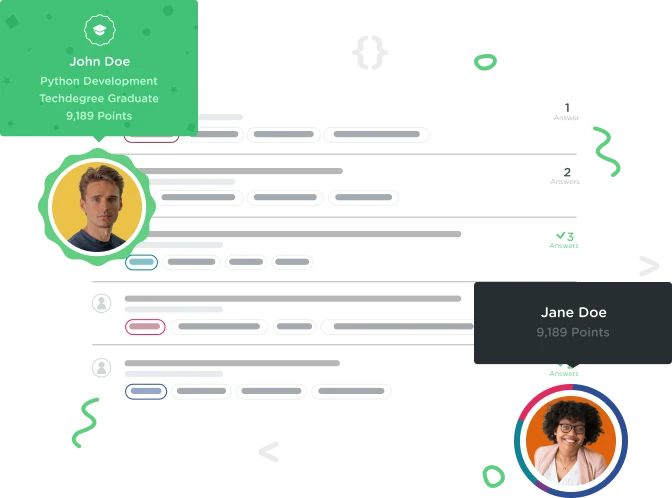

shu Chan
2,951 PointsFix the getLineNumberFor method
Hello! Now I know we already have a correct answer to this question on the forum but I wanted to go at this exercise in a different angle. Could you tell me if what I'm doing is viable?
Here is my thinking process:
char name = lastName.charAt(0); - I am naming the first letter of the last name as "name"
if (name < 'N') {lineNumber = 1;} else {lineNumber = 2;}
- I am comparing "name" with N if it is before N than it will be assigned number 1 else 2
return lineNumber;
- I am returning the lineNumber to get the line number
Does this not work because the user hasn't inputted their name in yet so the char name line doesn't work?
public class ConferenceRegistrationAssistant {
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
char name = lastName.charAt(0);
if (name < 'N') {lineNumber = 1;} else {lineNumber = 2;}
return lineNumber;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
}
}
import java.util.Scanner;
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
2 Answers

andren
28,558 PointsDoes this not work because the user hasn't inputted their name in yet so the char name line doesn't work?
The lastName
is a parameter. That means that it is assigned a value when the method is called. Which means you can safely assume that is has been set with some value when you are writing your code, even though you won't know exactly what that value is yourself.
All of the code you went though is valid, and your logic is also fine. The reason your solution does not pass the challenge is this line you have placed at the top of the class:
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
You are creating an instance of the class ConferenceRegistrationAssistant
within the ConferenceRegistrationAssistant
class. Which is not necessary and will in fact create an infinite loop of Java creating ConferenceRegistrationAssistant
instances.
If you remove that line then your code will work.

shu Chan
2,951 PointsOh Fantastic! I'm so excited it works using my own creativity! In your opinion which is better? The code I cooked up myself or this one here?
String sequence = "ABCDEFGHIJKLM";
if(sequence.contains((lastName.charAt(0)+"").toUpperCase()))
{
lineNumber = 1; }else { lineNumber = 2; }