Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial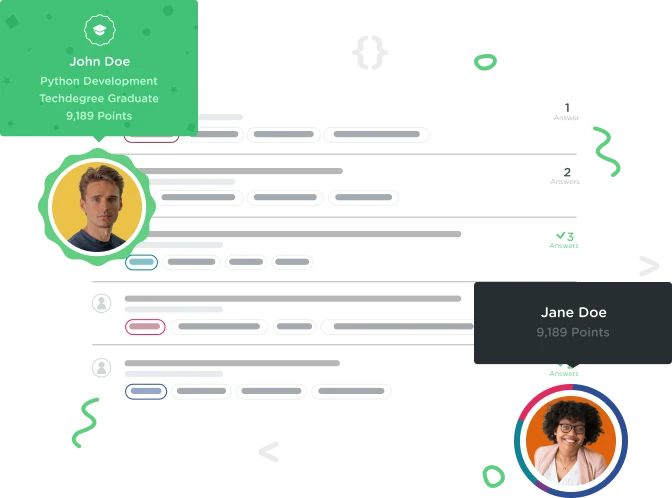

Johnny Baltazar
2,504 PointsFinding the sum of a "counter" variable loop that ran ten times then was pushed into the "numbers" array.
Hi everyone, I'm asking for help to find the sum of an array with elements that were pushed from a counter variable that had previously looped 10 times. I'm new to Javascript and was practicing for an assessment, and I've tried several different ways to do it and have only resulted with just a list of the elements within the numbers array. The following code is where I'm at. Thanks in advance.
var counter = 10;
var numbers = [];
for (i = 1; i <= 10; i ++) {
counter = [i + 73];
numbers.push(counter);
}
console.log(numbers);
function sum(arr) {
var s = 0;
for(var i = 0; i < arr.length; i++) {
s = s += arr[i];
}
return s;
}
console.log(sum([numbers]));
function getArraySum(a) {
var total = 0;
for (var i in a) {
total += a[i];
}
return total;
}
var numbers = getArraySum([numbers]);
console.log(numbers);
1 Answer

jb30
44,807 PointsIn your first for loop, you have
counter = [i + 73];
numbers.push(counter);
which sets an array to counter
and adds it to your numbers
array. If you wanted to add a number directly to the numbers
array, you could try counter = i + 73;
Instead of console.log(sum([numbers]));
and var numbers = getArraySum([numbers]);
, have you tried console.log(sum(numbers));
and var numbers = getArraySum(numbers);
? If you use [numbers]
, then it would create an array whose first element is the array numbers
.
In your getArraySum
function, you have
for (var i in a) {
total += a[i];
}
Try using total += i;
instead, since i
is already an element of a
.
In your sum
function, you have s = s += arr[i];
This doesn't cause any problems, but the s =
at the beginning is redundant. You probably meant either s = s + arr[i];
or s += arr[i];
Johnny Baltazar
2,504 PointsJohnny Baltazar
2,504 PointsThanks for the help JB30!