Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial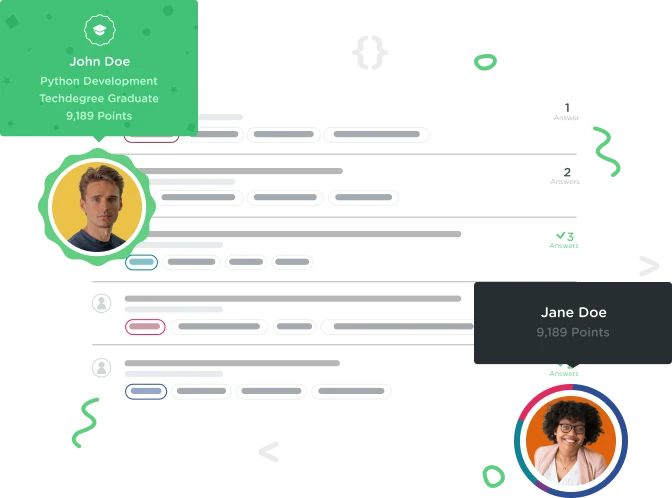
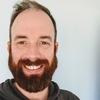
Jeremy Schaar
4,728 PointsFailed example, but Expected: / Got: identical
I'm getting the below. Any ideas what's up? It's failing, but "Expected:" and "Got:" look identical to me.
File "/home/treehouse/workspace/dungeon/dd_game.py", line 64, in dd_game.get_moves
Failed example:
get_moves((0,2))
Expected:
['RIGHT', 'UP', 'DOWN']
Got:
['RIGHT', 'UP', 'DOWN']
**********************************************************************
1 items had failures:
1 of 2 in dd_game.get_moves
***Test Failed*** 1 failures.
3 Answers
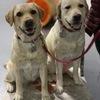
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Jeremy,
You have a really subtle bug in your code. If you look at the expected result line in your code, there is a trailing space character. Since "['RIGHT', 'UP', 'DOWN']
" has one different character to "['RIGHT', 'UP', 'DOWN']
", your test is failing.
I imagine this kind of thing happens a lot with doctests, which is probably why Kenneth copy-pasted the output from the shell into his code rather than typing it.
Cheers,
Alex
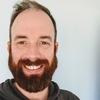
Jeremy Schaar
4,728 PointsSorry about that.
"""Dungeon Game
Explore a dungeon to find a hidden door and escape.
Watch out for the monster.
Created 2014
Updated: 2015
Author: Kenneth Love
"""
import os
import random
GAME_DIMENSIONS = (5, 5)
player = {'location': None, 'path': []}
def clear():
"""Clear the screen"""
os.system('cls' if os.name == 'nt' else 'clear')
def build_cells(width, height):
"""Create and return a width x height grid of two tuples
>>> cells = build_cells(2, 2)
>>> len(cells)
4
"""
cells = []
for y in range(height):
for x in range(width):
cells.append((x, y))
return cells
def get_locations(cells):
"""Randomly pick starting locations for the monster, the door,
and the player
>>> cells = build_cells(2, 2)
>>> m, d, p = get_locations(cells)
>>> m != d and d != p
True
>>> d in cells
True
"""
monster = random.choice(cells)
door = random.choice(cells)
player = random.choice(cells)
if monster == door or monster == player or door == player:
monster, door, player = get_locations(cells)
return monster, door, player
def get_moves(player):
"""Based on the tuple of the player's position, return the list
of acceptable moves
>>> GAME_DIMENSIONS = (2, 2)
>>> get_moves((0,2))
['RIGHT', 'UP', 'DOWN']
"""
x, y = player
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
if x == 0:
moves.remove('LEFT')
if x == GAME_DIMENSIONS[0] - 1:
moves.remove('RIGHT')
if y == 0:
moves.remove('UP')
if y == GAME_DIMENSIONS[1] - 1:
moves.remove('DOWN')
return moves
def move_player(player, move):
x, y = player['location']
player['path'].append((x, y))
if move == 'LEFT':
x -= 1
elif move == 'UP':
y -= 1
elif move == 'RIGHT':
x += 1
elif move == 'DOWN':
y += 1
return x, y
def draw_map():
print(' _'*GAME_DIMENSIONS[0])
row_end = GAME_DIMENSIONS[0]
tile = '|{}'
for index, cell in enumerate(cells):
if index % row_end < row_end - 1:
if cell == player['location']:
print(tile.format('X'), end='')
elif cell in player['path']:
print(tile.format('.'), end='')
else:
print(tile.format('_'), end='')
else:
if cell == player['location']:
print(tile.format('X|'))
elif cell in player['path']:
print(tile.format('.|'))
else:
print(tile.format('_|'))
def play():
cells = build_cells(*GAME_DIMENSIONS)
monster, door, player['location'] = get_locations(cells)
while True:
clear()
print("WELCOME TO THE DUNGEON!")
moves = get_moves(player['location'])
draw_map(cells)
print("\nYou're currently in room {}".format(player['location']))
print("\nYou can move {}".format(', '.join(moves)))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move in ['QUIT', 'Q']:
break
if move not in moves:
print("\n** Walls are hard! Stop running into them! **\n")
continue
player['location'] = move_player(player, move)
if player['location'] == door:
print("\n** You escaped! **\n")
break
elif player['location'] == monster:
print("\n** You got eaten! **\n")
break
if __name__ == '__main__':
play()
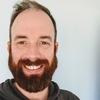
Jeremy Schaar
4,728 PointsTHANKS!
Alex Koumparos
Python Development Techdegree Student 36,887 PointsAlex Koumparos
Python Development Techdegree Student 36,887 PointsHi Jeremy,
Can you post your failing code?