Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial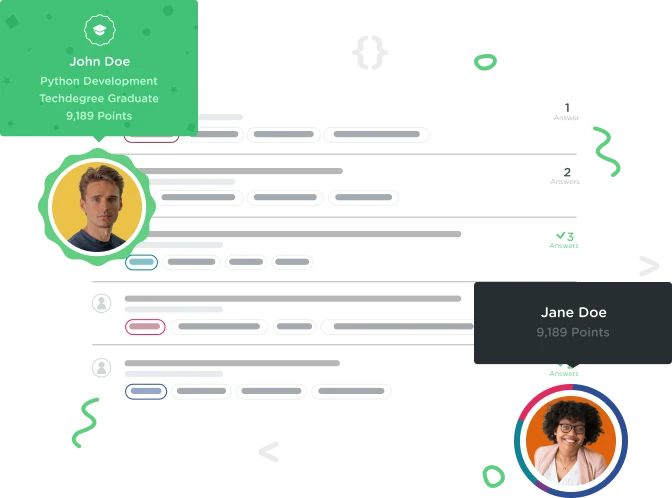

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 Pointsevent listener. hints, suggestions and comments are very much appreciated thank you
Question:
Now create an event listener on linkList that will be called when there is a click event.
Inside this event listener, write a conditional that checks if the tagName of the event target (i.e. the element clicked) is a BUTTON element.
If that condition is met, use querySelector to select the first element with a class of active and then set the className property to an empty string. This will remove the active class from the previous button. We can use querySelector here rather than looping over all of the buttons because there should only ever be one button with the active class.
Still inside of the conditional, lets next add the active class to the button that was clicked (i.e. the click target). Again, we can do this using the className property.
Last but not least, still in the conditional, we will call the showPage function passing the list parameter and the text content of the click target as arguments. We can access the text content of the click target by using the textContent property.
At this point, your addPagination function should be finished. Let's test it to make sure everything works as expected. Be sure that you are calling the addPagination function and passing data as an argument. If you refresh the page, you should see five pagination buttons on the screen. Clicking a button should display the nine students for that “page”.
My answer: buttonDiv.addEventListener('click', (event) => { let buttonNumber = parseInt(event.target.textContent); let max = buttonNumber * 10; let min = max - 10; for (let i = 0; i < StudentListItem.length; i++) { if (i >= min && i < max) { StudentListItem[i].style.display = ''; } else { StudentListItem[i].style.display = 'none'; } } });//evernt listener
}
console.log(buttonUl); console.log(TotalPages);
3 Answers
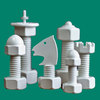
Steven Parker
241,970 PointsWhen posting code, use Markdown formatting to preserve the code's appearance and retain special symbols.
Depending on how the variables are established (not shown here), this code looks like it might appear to be functional. But it works in a completely different way from what is asked for:
- the instructions ask for a "conditional that checks if the tagName of the event target is a BUTTON element.", but this code makes no such check.
- then, the instructions ask that you use "
querySelector
to select the first element with a class of active", but this code is neither using that function nor selecting by class. - the instructions explain that querySelector is used "rather than looping over all of the buttons", but this code is looping over the buttons.
- the instructions ask for the active class to to be added using the className property, but that isn't being done here. This code is working on the display property instead.
- finally, the instructions say to call the showPage function, which is never done here.

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsSteven Parker hello sir apologies for the late reply but i already passed unit 2 :),
but if i may humbly ask for your help for unit 3 as below:
Im taking my time with this project and i am going to do it step by step bu when in downloaded the project files there was no js file and when i read through the instructions it said to make your own js file so i am making one right now:
The "Name" field: When the page loads, the first form field should be in focus, meaning it should have a flashing cursor and/or be highlighted in some way.
Create a variable to reference the “Name” <input type="text"> element and log the variable out to the console to ensure the correct element is being referenced. Use the variable and dot notation to set the focus property of the element to true. Now save and refresh the page, and the “Name” field should have the focus state. "Job Role" section The "Job Role" section has an <input type="text"> field where users can enter a custom job role. If the user selects "Other" in the "Job Role" drop down menu, they can enter info into the "Other job role" text field. But this field should be hidden by default and only displayed once users select "Other" in the drop down menu, and be hidden if the user selects any other option.
Hide the "text field" with the id of "other-job-role" so it is not displayed when the form first loads. Program the "Job Role" <select> element to listen for user changes. When a change is detected, display/hide the "text field" based on the user’s selection in the drop down menu.
My answer is:
HTML CODE:
<label for="name">Name: <span class="asterisk">*</span> <input type="text" id="name" name="user-name" class="error-border"> <span id="name-hint" class="name-hint hint">Name field cannot be blank</span> </label>
<label for="email">Email Address: <span class="asterisk">*</span>
<input type="email" id="email" name="user-email" class="error-border">
<span id="email-hint" class="email-hint hint">Email address must be formatted correctly</span>
</label>
<label for="title">Job Role</label>
<select id="title" name="user-title">
<option hidden>Select Job Role</option>
<option value="full-stack js developer">Full Stack JavaScript Developer</option>
<option value="front-end developer">Front End Developer</option>
<option value="back-end developer">Back End Developer</option>
<option value="designer">Designer</option>
<option value="student">Student</option>
<option value="other">Other</option>
</select>
<input type="text" name="other-job-role" id="other-job-role" class="other-job-role" placeholder="Other job role?">
</fieldset>
Convert to JavaScript:
let nameField = document.getElementsByTagName('INPUT')[0];
console.log(nameField);
nameField.focus();
let emailField = document.getElementById('email');
console.log(emailField);
emailField.focus();
let jobRoleSelection = document.getElementById("title"); let basicInfoSection = document.getElementsByTagName("basicinfo")[0]; let designSelection = document.getElementById("design"); let colorSelection = document.getElementById("color"); let hiddenColorOptions = []; console.log(jobRoleSelection); console.log(basicInfoSection); console.log(designSelection); console.log(colorSelection); jobRoleSelection.focus(); basicInfoSection.focus(); designSelection.focus(); colorSelection.focus();
Any hints, suggestions, and comments are very well appreciated. thank you in advance for all your help.
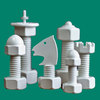
Steven Parker
241,970 PointsAlways start a new question instead of asking one in an answer (you seem to have already done this in a separate posting).

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsSteven Parker i have done it. thank you for your advise i have marked best answer. sir could you help me with unit 3? if i may humbly ask? https://teamtreehouse.com/community/job-role-section-html-to-javascript-how-do-i-convert-the-html-code-to-javascript . thank you for your time
Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsKarl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 Points@steven Parker hello sir apologies for the late reply:
This is my updated code:
onload = () => {
const doc = document, qsel = doc.querySelector.bind(doc), qall = doc.querySelectorAll.bind(doc), create = doc.createElement.bind(doc);
const students = qall(':not(.page) li');
const ipp = 9; // items per page
function showPage(page) { /* Create the
showPage
function This function will create and insert/append the elements needed to display a "page" of nine students */ const count = students.length; for (let i = 0; i < count; ++i) students[i].style.display = i < page*ipp && i >= (page-1)*ipp ? 'block' : 'none'; active(page); }function addPagination() { /* Create the
addPagination
function This function will create and insert/append the elements needed for the pagination buttons */ const main = qsel('.page'), div = create('div'), ul = create('ul'), total = Math.ceil(students.length/ipp); main.appendChild(div); div.appendChild(ul); div.className = 'pagination'; for (let i = 0; i < total; ++i) { const li = create('li'), a = create('a'); Object.assign(a,{ href: '#', textContent: i+1 }); ul.appendChild(li).appendChild(a); } div.addEventListener('click',evt => { if (evt.target.nodeName != "A") return; const page = +evt.target.textContent, max = page*ipp, min = max-ipp; for (let i = 0; i < students.length; ++i) students[i].style.display = min <= i && i < max ? '' : 'none'; active(page); }); }function active(page) { let e = qsel('.active'); e && e.classList.remove('active'); qall('a')[page-1].classList.add('active'); }
// Call functions addPagination(); showPage(1);
};
Steven Parker
241,970 PointsSteven Parker
241,970 PointsPlease review that information about Markdown formatting and use it when posting code to the forum.
This code still doesn't perform the steps outlined in the instructions. You might have better results if you start over and implement one part of the instructions at a time before moving on to the next.
It also looks like a lot of "tricky" code (such as "curried" functions) was added which might help make the code more compact but more difficult to read. If this is for one of the challenges, the tricky bits might make it difficult for the automated evaluation process to interpret the code even after it conforms to the instructions.