Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial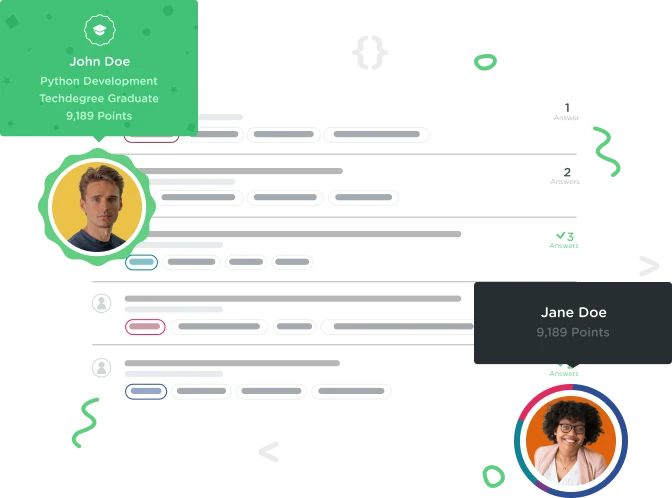

Arun Kampadathil
2,429 Pointserror: it says, "results" should contain first 10 multiples of 6, could someone help please?
error: it says, "results" should contain first 10 multiples of 6, could someone help please?
// Enter your code below
var results: [Int] = []
for multiplier in 1...10
{
let value: Int = multiplier * 6
results = [value]
}
3 Answers
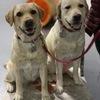
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Arun,
Your problem is with this line:
results = [value]
The =
operator performs assignment, so in every iteration of the loop you assign (replace) the value in results
with the current iteration's value of [value]
. What you want to do is combine the iteration's value with the value already in results
.
One way of doing this is assign the old value of results
plus the new value in [value]
to results
. The syntax for doing this looks like:
results = results + [value]
Swift (like many languages) recognises that this is such a common construction that they add some syntactic sugar to let you write this same expression and assignment in a more concise way:
results += [value]
Hope that clears everything up
Cheers
Alex
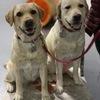
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Arun,
Don't pay too much attention to the type of the data. Think of a variable as a finger that can point at one thing, whether that is an individual item (like a number or boolean) or a box of things (like an array or a dictionary). Every time you use the assignment operator you are just pointing the finger at a different thing.
You say that results
is 'in an array', but that's not accurate. results
isn't 'in' anything, it is just pointing to the thing that happens to be an array.
In the specific case of your code, you first put 6 in a box and tell your results
finger to point that the box of 6, then you go through the loop again and put 12 in a new box. Then you move your finger so it now points at the box of 12 (since nothing is using the box of 6 anymore, it now disappears from memory). This process repeats for the duration of the loop.
Cheers
Alex

Mark Bailey, Jr.
7,403 PointsThanks Alex! This helped me a lot!
Arun Kampadathil
2,429 PointsArun Kampadathil
2,429 Pointsthank you alex, now the code is working, I understood what you saying, but my question is, if the variable "results" is a variable which stores only a single value, but in this case the variable "results" is an array, so why the previous value in an array is replaced by the current value in each iteration?