Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial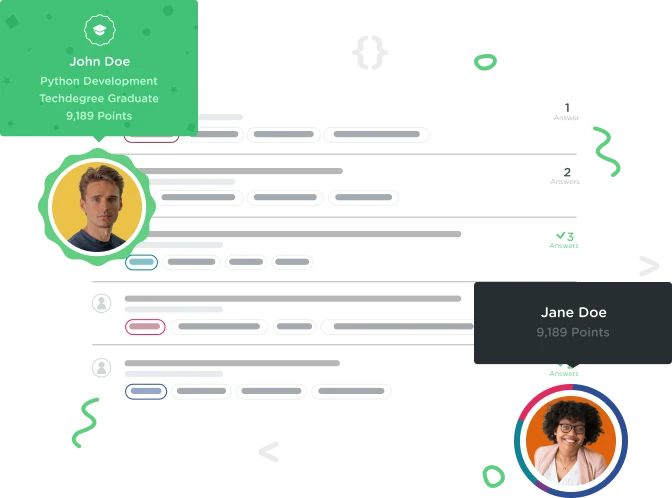
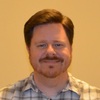
Geoffrey Emerson
18,726 PointsError in build.gradle: "No service of type StyledTextOutputFactory available in ProjectScopeServices."
This error pops up when following the "Introducing Spring Data REST" video.
Here's a possible solution derived from https://github.com/spring-gradle-plugins/dependency-management-plugin/issues/87
buildscript {
repositories {
mavenCentral()
maven { url "https://repo.spring.io/plugins-snapshot" }
maven { url "https://repo.spring.io/snapshot" }
maven { url "https://repo.spring.io/milestone" }
}
dependencies {
classpath "io.spring.gradle:dependency-management-plugin:0.6.0.BUILD-SNAPSHOT"
classpath('org.springframework.boot:spring-boot-gradle-plugin:1.3.3.RELEASE')
}
}
These additions make the error go away, but I don't know if they will cause problems later in this course.
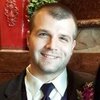
Michael Gulley
19,465 PointsThanks!
Additionally, if you replace the spring-boot-gradle-plugin with version 1.4.0 or above, the error does not appear. That being said, I don't know yet if there will be any implications resulting from upgrading the version later on in the course.
https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-gradle-plugin
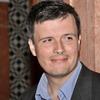
Brian Patterson
19,588 PointsThanks Geoffrey.
3 Answers
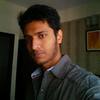
Ajinkya Borade
Courses Plus Student 16,635 Pointsuse https://start.spring.io/ next time to generate your project and then load it in Intellij IDEA. And find appropriate version at https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-gradle-plugin/1.5.10.RELEASE
This is my gradle config, works fine. since Im also using maven repo links.
group 'com.ajinkya.th'
version '1.0-SNAPSHOT'
buildscript {
ext {
springBootVersion = '1.5.10.BUILD-SNAPSHOT'
}
repositories {
mavenCentral()
maven { url "https://repo.spring.io/snapshot" }
maven { url "https://repo.spring.io/milestone" }
}
dependencies {
classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}")
}
}
apply plugin: 'java'
apply plugin: 'idea'
apply plugin: 'org.springframework.boot'
sourceCompatibility = 1.8
repositories {
mavenCentral()
maven { url "https://repo.spring.io/snapshot" }
maven { url "https://repo.spring.io/milestone" }
}
dependencies {
compile("org.springframework.boot:spring-boot-starter-web")
compile("org.springframework.boot:spring-boot-starter-thymeleaf")
testCompile("org.springframework.boot:spring-boot-starter-test")
}
https://spring.io/guides/gs/accessing-data-rest/ If you just want to use JAVA Spring rest
buildscript {
repositories {
mavenCentral()
}
dependencies {
classpath("org.springframework.boot:spring-boot-gradle-plugin:2.0.0.RELEASE")
}
}
apply plugin: 'java'
apply plugin: 'eclipse'
apply plugin: 'idea'
apply plugin: 'org.springframework.boot'
apply plugin: 'io.spring.dependency-management'
bootJar {
baseName = 'gs-accessing-data-rest'
version = '0.1.0'
}
repositories {
mavenCentral()
}
sourceCompatibility = 1.8
targetCompatibility = 1.8
dependencies {
compile("org.springframework.boot:spring-boot-starter-data-rest")
compile("org.springframework.boot:spring-boot-starter-data-jpa")
compile("com.h2database:h2")
testCompile("org.springframework.boot:spring-boot-starter-test")
}
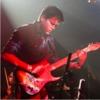
Matthew Germeyer
12,374 PointsThanks, I decided to use this as a gradleStarter for a basic Spring project.

Suyang Wang
2,286 PointsIt works for me. Thank you so much!

Chris Stratton
252 Pointsbuildscript { ext { springBootVersion = '2.0.3.RELEASE' } repositories { mavenCentral() } dependencies { classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}") } }
apply plugin: 'java' apply plugin: 'org.springframework.boot' apply plugin: 'io.spring.dependency-management'

Katsiaryna Krauchanka
Full Stack JavaScript Techdegree Graduate 22,154 PointsThat was very helpful, thanks Geoffrey Emerson! Works also for the course Unit Testing a Spring Application.
Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsChris Jones
Java Web Development Techdegree Graduate 23,933 PointsVery helpful...thank you!