Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial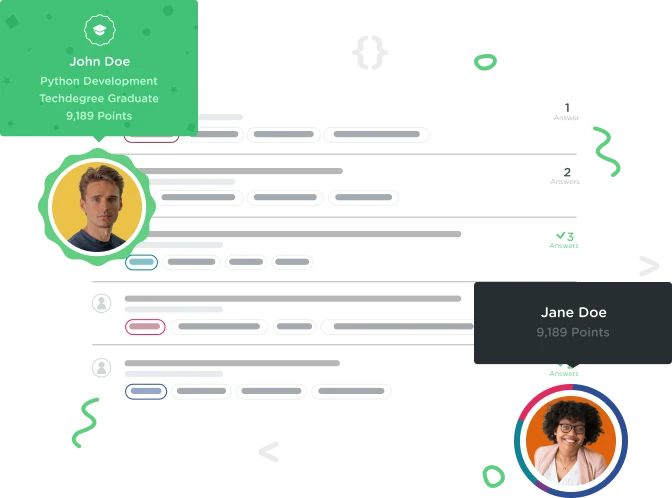

Anna Riehle
21,755 PointsError: “Can’t get the length of a Hand”
For Task 2 of the last challenge of the Object Oriented Python course, I keep getting the error “Can’t get the length of a Hand”, and I’m not sure why. I’ve tried troubleshooting for 2 hours in various ways, including building a len method, with no luck. What might be causing this, and how can I get around it? Code is attached.
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self):
super().__init__(sides=20)
from dice import D20
class Hand(list):
@property
def total(self):
return sum(self)
def roll(num):
l = []
for _ in range(num):
l.append(D20())
return l
4 Answers
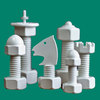
Steven Parker
230,970 PointsThe instructions say to "Create a classmethod", but you forgot to use the decorator.
The remaining instructions are incorrect. Instead of plain list, the challenge is expecting you to return a new instance of Hand with the two D20's in it. So you'll want to make this a
I've made a bug report to Support about this but perhaps it wouldn't hurt to send them an additional one.
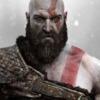
boi
14,242 PointsHeyyo Anna!
First thing is to make sure you make your "roll" method into a "Class Method";
@classmethod
def roll(cls,num):
pass
Notice that "cls" parameter? Class methods take "cls" parameter ( As far as I know).
and your "roll" method needs to return a new instance with the list you created.
WARNING SPOILER
from dice import D20
class Hand(list):
@property
def total(self):
return sum(self)
@classmethod
def roll(cls,num):
l = []
for _ in range(num):
l.append(D20())
return cls(l)
There are ALOT of people with the exact same problem as yours and many excellent explanations, just go to the community and search "Code Challenge RPG roller" and you will find tons of excellent answers.
I hope this helped

Anna Riehle
21,755 PointsDefinitely - thank you so much!

Manish Kumar Meena
Python Development Techdegree Student 2,594 Pointsfrom dice import D20
class Hand(list):
@property
def total(self):
return sum(self)
@classmethod
def roll(cls,num):
l = []
for _ in range(num):
l.append(D20())
return cls(l)