Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial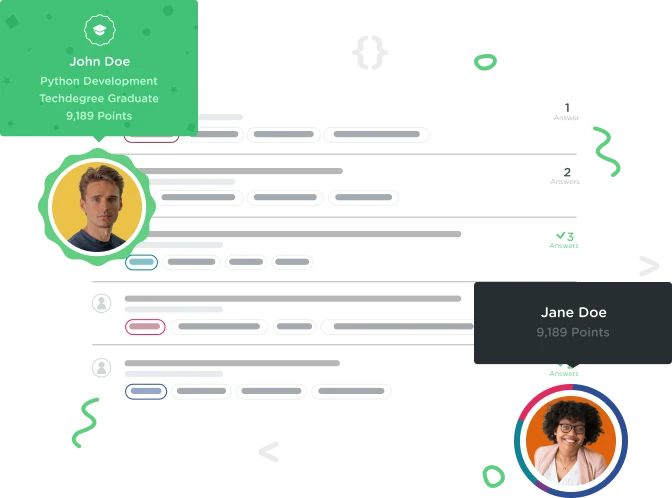

Lawrence Yeo
3,236 PointsDon't you have to unwrap the task.status enum value?
I was stuck on this problem because I was trying to unwrap the task.status enum value, but it looks like Amit has it so that you don't need to do so. Why is that?
1 Answer
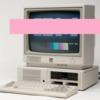
kols
27,007 PointsI wondered about this, too.
I believe what you're talking about is converting the status to an actual string, like "Pending." This would be a good next step if you wanted to display the status for a given task back in the form of a string (e.g., "Pending", "Doing", "Complete") instead of just showing '(Enum Value).'
I believe Amit didn't include it in the solution because it's not necessary to complete the challenge; i.e., the code still works, it just won't show you a description of the status in the form of a string... I'm not much further along than this in the coursework, though, so someone else may have a better answer for you.
enum Status {
case Doing, Pending, Complete
init() {
self = .Pending
}
func statusString() -> String {
switch self {
case .Pending:
return "Pending"
case .Doing:
return "Doing"
case .Complete:
return "Complete"
default:
return "No Status Found"
}
}
}
struct Task {
var description: String
var status = Status()
init(desc: String) {
self.description = desc
}
}
You can test that this is working with the following code:
var newTask = Task(desc: "It's a new task")
newTask.description
newTask.status.statusString()
newTask.status = .Complete
newTask.status.statusString()
Enrique MunguÃa
14,311 PointsEnrique MunguÃa
14,311 Pointscan you provide some code?