Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial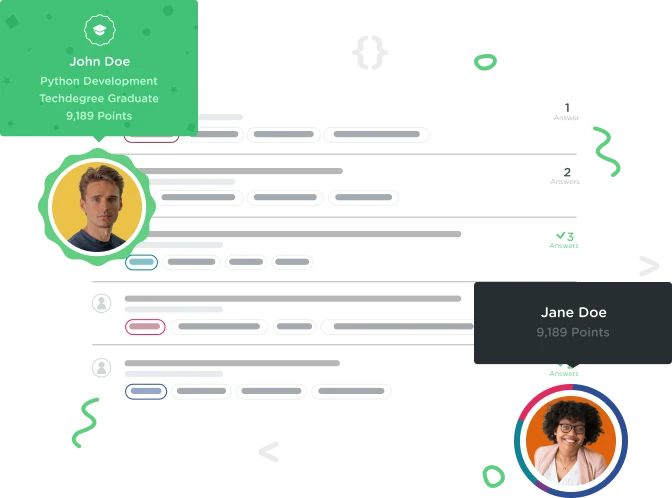

Aditya Puri
1,080 PointsDon't understand the reference thing.
I don't really understand the whole reference thing.
1) What is a reference
2)Why were the objects not equal
3)when we did Object object = object2
, then how was that true?
4) Why were the Strings equal if they were objects too?
5) When we did String string = new String("something")
, why weren't the STrings equal then?
This is a long question. In a nutshell i am asking what the whole video means .Please help and take time to answer this question.
5 Answers

Paul West
6,925 PointsYep, that was a really bad place to put that video. But let me try to help.
==
When comparing to "primitives" (only two you have seen are char and int I think but there are more) == compares the values. example int x = 2; int y = 2; x == y is true;
now String is not a primitive(the dead giveaway is the S is capitalized) it is a object
Objects: When you define a class you are defining a blueprint for an object. You can think of this as if I was an architect and I came up with the blueprints for a house. Now I could ask a builder to make two identical houses Smiths House and Jones House. In Java this would look like this:
House smithsHouse = new House(); House jonesHouse = new House();
now if I use the == like this:
smithsHouse == jonesHouse the answer is false (answer to your second question).
Even if the Smith's and Jones both have the same number of people in their family. These are still two different houses(objects) now if I said that the Hilens lived with the Jones in the same house this would look like this in code:
jonesHouse = hilensHouse (thus hilensHouse could be said to be referencing joneHouse. question 1 );
Then we are talking about the same house with different names so:
jonesHouse == hilensHouse is true(third question).
If you are still with me great! Now that we have objects out of the way notice how I told Java to make a house(object) from my blueprint(class). Standard format for this is
Object someName = new object();
Notice the object() is actually the constructor which is named the same thing as the class. Keeping with the analogy of a house, you can think of this as the options field. In this case I am saying give me a standard house with no options denoted by the empty (). I could have given the customer the option to change things like maybe door color. If the Jones and the Smiths both wanted the same color doors would they still live in separate houses?
House smithsHouse = new House(redDoor) and house jonesHouse = new House(redDoor)
and so smithsHouse == jonesHouse.
Now taking it back to Strings when
String stringOne = new String("abc");
String stringTwo = new String("abc");
are defined in the standard object protocol like this, they are two different object just like smithsHouse and jonesHouse where above. So when you compare them:
stringOne == stringTwo the answer is false. (question 5)
Now that we are done with the other questions we come back to question land mind of question 4. Notice if I want to define string normally. This is done by typing
String someName = "some string"; String someOtherString = "some string";
Notice the lack of a constructor. There is no new String(). In this case the "some string" is not in a new object and because Java is looking to save on space it stores identical strings in the same place in memory. So now someName == someOtherString is true because Java has made it true to save space in memory and both fields are referencing the same thing. (question 1 again)
Hope this helped

Aditya Puri
1,080 Pointsthx! but what does interning mean? is it intering?? i dont know if the spelling is right

Aaron Brewer
7,939 PointsThis is a really good conceptually explanation.

Aditya Puri
1,080 Pointsi didnt get what you said about and so smithsHouse == jonesHouse

Amir Tamim
8,597 PointsBetter explanation than the video, great help.

Lakshmi Narayana Dasari
1,185 PointsHi Paul West and Aditya Puri. Paul that was a great explanation but a small error i guess.
House smithsHouse = new House(redDoor) and house jonesHouse = new House(redDoor) and so smithsHouse == jonesHouse.
In the above line smithsHouse cannot be equal to jonesHouse.
For example String differentName = new String("hello"); String anotherName = new String("hello");
Object references must refer to the same object to use double equals.whenever we use a new keyword , java creates a new string object at a specified location in the memory(memory address).So as they cannot point to same memory as they both use new keywords they are pointing to two new different memory locations , hence they cannot be equal.
String m = "abcd"; // This is known as string literal. And this string literal have an intern method.whenever a string literal is created, the intern method is called. The intern method(just references an intern pool of string objects) just checks the string pool whether it has the same identical string.If it didn't find any identical string then it creates a new string.If it finds a string with same characters then it just points to that memory location, means it just uses the reference.
String m3 = "abcd"// intern method checks pool of string objects..it finds that String m is identical to m3 and just points to that string instead of creating a new string.
String m2 = new String('abcd"); // New keyword is used so it creates new string at a specified location at a different address.

Tomas Verblya
1,998 PointsThank you guys, was just looking for extra information after the video, this really cleared it up. Sometimes it's easier to see things on paper, rather than video. Also the house analogy was really helpful. Cheers everyone!

Paul West
6,925 PointsHere is an article about it. Basically describes how and why String a = "abc" and String b = "abc" makes a == b true. https://dzone.com/articles/string-interning-what-why-and

Paul West
6,925 PointsAditya Puri. the == is used to compare to things in Java. In the above example I built two identical house using the same blueprints for both. one of those houses is occupied by a family with the last name of Smith the other is occupied by a family named Jones. When you use the compare == in Jave and compare the two houses they are not equal because even though they are built using the same plans(class) they are different house(objects). I hope this helps.

Aditya Puri
1,080 Pointsyeah, but you wrote that 1st family's house = second family'shouse. The line is
House smithsHouse = new House(redDoor) and house jonesHouse = new House(redDoor) and so smithsHouse == jonesHouse.
Florian Tönjes
Full Stack JavaScript Techdegree Graduate 50,856 PointsFlorian Tönjes
Full Stack JavaScript Techdegree Graduate 50,856 PointsA reference is a pointer to where an object resides in memory.