Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial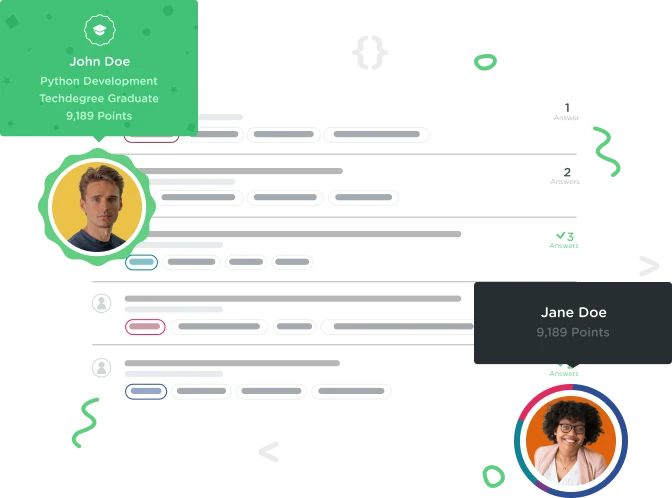

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsDOM Scripting By Example,The result should be in pixels (for example, the function could return: "50 px").
INSTRUCTIONS:
1.getWidth of the blue area 2.Return the width of the blue box 3.document.getElementById(id) method
JS CODE:
function getWidth(id) {
// Return the width of the element specified in the parameter "id"
}
// No need to change below this point.
// Note how we're using DOM elements here to display the result of the getWidth() function inside the "areaWidth" element
window.onload = () => {
document.getElementById('areaWidth').innerHTML = getWidth('mainDiv');
};
//don't change this line
if (typeof module !== 'undefined') {
module.exports = { getWidth };
}
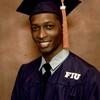
Dane Parchment
Treehouse Moderator 11,077 PointsWhat exactly do you need help with?
1 Answer
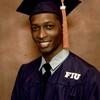
Dane Parchment
Treehouse Moderator 11,077 PointsAssuming you need help figuring out how to return the width of element
First we need to get the element that is using the specified id, we can do this via the document.getElementById
function. This returns an element with the associated id (the first one it comes across because their should only be a unique id per document)
function getWidth(id) {
let element = document.getElementById(id);
}
Next we need to get the width of that element, there are multiple ways to do this. We can get the offsetWidth if we want to return the actual calculated width of the element as a number. Or we can return the actual width entered in a style in the element's css by targeting it's style attribute, which will be returned as a string with the value and measurement.
In this case I think they want us to return the style as a value, so let's do that by targeting the style
attribute in the element.
function getWidth(id) {
let element = document.getElementById(id);
//We can return any css attribute here but it has to be camel case. For example
//background-color would be returned as style.backgroundColor
return element.style.width;
}
Now we can optimize the function to have a single return statement instead of saving a space in memory for that element variable. This isn't necessary but it's good programming practices to get into, it'll work with the above code as well.
function getWidth(id) {
return document.getElementById(id).style.width;
}

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 Pointsthank you for your idea it helped me a lot Mr.Dane Parchment
Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsKarl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsAny hints and or suggestions is very much appreciated, thank you in advance for your help.