Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial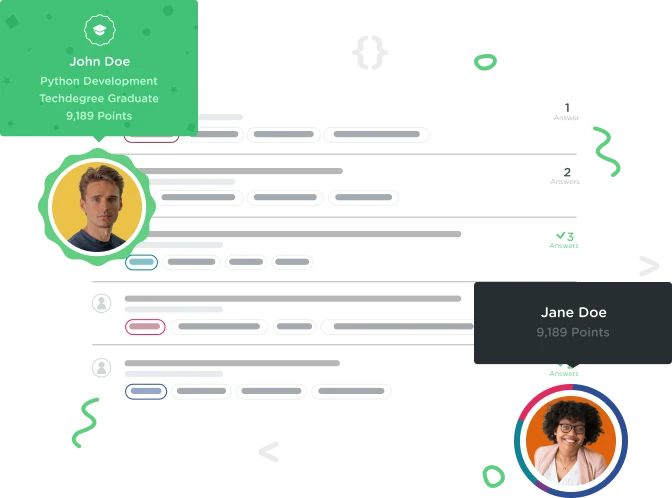

Baishali Chetia
1,149 PointsDoes it work if we use only "if" every time, instead of "else if" ?
Can we just do an "if" statement every time instead of "else if" and get the same results? for example:
// when the player collects an item on the screen
function itemHandler(player, item) {
item.kill();
if ( item.key === 'coin') {currentScore = currentScore + 10;
}
if ( item.key === 'poison') {currentScore = currentScore - 25;
}
if ( item.key === 'star') {currentScore = currentScore + 25;
if (currentScore === winningScore) {
createBadge();
}
}
Will this give the same result? I am not able to try it out myself as my Coin collector game preview for this video is not opening.
Please help! Thank you :)
3 Answers

Luis Rodriguez
7,275 PointsIf you use an if statement for every single condition then it would be in a sense a waste of time. For example if you had a 100 cases, and let's say you were trying to find item.key === coin if the first 'if' was if(item.key === coin) {...} then there you found your answer, BUT then if all the rest of the items were 'if' instead of 'else if' then it would have check every single 'if' statement remaining. if you would have used 'else if' then once you found your answer then it would skip the rest of the statements and run the next part of your code. Writing all 'if' statements would slow down your program. In your case since you have about 3 'if' for checking the item you won't really see a difference in performance, but if it was a large amount of 'if' statements then yes you would see a performance difference. But you should use
if (condition){}
else if(condition){}
else if(condition){}
else{}
because it is best practice and it is expected of you to write your code this way.

Baishali Chetia
1,149 PointsThis was very helpful. I understand better now. Thank you!
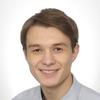
Sergey Podgornyy
20,660 PointsIf you are not using else if
statement, your program will continue execution, even after it found proper condition. Separate condition, additional check. If you use checking in if ... else if ... else
it will stop comparing after some condition will be truth.
But in your case I suggest you to use switch ... case
:
// when the player collects an item on the screen
function itemHandler(player, item) {
item.kill();
switch (item.key) {
case 'coin':
currentScore += 10;
break;
case 'poison':
currentScore -= 25;
break;
case 'star':
currentScore += 25;
break;
}
if (currentScore === winningScore) {
createBadge();
}
}

Baishali Chetia
1,149 PointsThis makes sense. thank you !
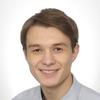
Sergey Podgornyy
20,660 PointsYou are welcome

Tobiasz Gala
Full Stack JavaScript Techdegree Student 23,529 PointsWhen you use if statement alone this condition may never happen what else gives you is that something must happen at least once.
if (false) {
// if this will never going to happen then else will always run
} else {
// this will happen
It is normal to use if statement alone when we need to check for certain condition. But when you need something happened if the condition is not correct you use else. You can also check more conditions using else if
if (condition1) {
//do this
} else if (condition2) {
// if condition 1 is wrong but codition2 is correct do this
} else {
// if both conditions are wrong always do this
}

Baishali Chetia
1,149 PointsThis was very helpful. Thank you!

Wayne Topp
948 PointsSo, just to be clear. In this case, there is no need for an else statement because there are only three possible things the player could pick up: coin, poison, or star. Since one of those conditions will always be met, there is not need for an else statement.
Jonathan Mitten
Courses Plus Student 11,197 PointsJonathan Mitten
Courses Plus Student 11,197 PointsFor this example, the results may well be the same, but there's an important reason why
else if
benefits you and your users.else if
conditions will not attempt to execute if an earlier condition is met, therefore freeing up that much more memory. With a series of simpleif
statements, each and every one of them will be evaluated - they'll all run, but they don't all need to run.Similarly, although it may be outside the scope of your question, you could look into a
switch
statement if you're attempting to evaluate the sameitem.key
, which would make your code even easier to read. Switch statements take a single expression as an argument, and a series of match cases. Read up switch statements here