Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial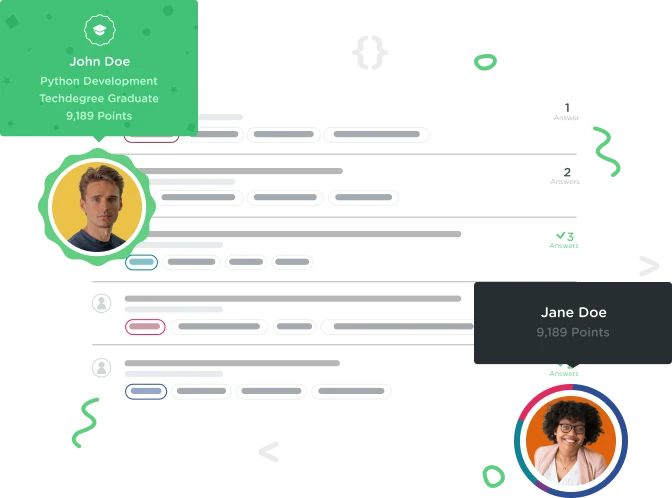

Joshua Dam
7,148 PointsDisemvowel - Issues with code
Here's my code. It seems to work properly in Workspaces but it does not pass for the challenge. Can't figure out what's wrong.
def disemvowel(word):
for letters in word:
try:
word.remove('a')
except ValueError:
pass
try:
word.remove('e')
except ValueError:
pass
try:
word.remove('i')
except ValueError:
pass
try:
word.remove('o')
except ValueError:
pass
try:
word.remove('u')
except ValueError:
pass
try:
word.remove('A')
except ValueError:
pass
try:
word.remove('E')
except ValueError:
pass
try:
word.remove('I')
except ValueError:
pass
try:
word.remove('O')
except ValueError:
pass
try:
word.remove('U')
except ValueError:
pass
my_word = ''.join(my_list)
return my_word
my_list = list('Alligator')
disemvowel(my_list)
3 Answers
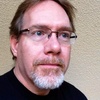
Chris Freeman
Treehouse Moderator 68,428 PointsThe word
argument is a string which does not have a .remove()
method. Create a list out of the word
, then remove letters from this new list. Be careful not to modify the same container as is used in the for
statement. This will cause the indexes to be off resulting in letters skipped (possible not removed). For example:
for letter in letter_list[:]: # notice the use of slice to create a copy so the removes don't affect the loop.
Within the function, my_list
is not defined. The argument to the join
should be the resultant list with the letters removed.
Also, when running the challenges, you typically do not need to include a call to the function or initialization values.
Post back if you're still having issues. Good luck!!
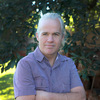
Christopher Shaw
Python Web Development Techdegree Graduate 58,248 PointsThere are two mistakes that I can see.
- The challenge will be giving you a string, your workings are for a list. So convert the string to a list before the for statement. For example.
word=list(word)
- Near the end, you have slipped in
my_list
, where it should beword
my_word = ''.join(word)
This should get it passing, but later you should come back and re look at the challenge as you will find it can be done in far fewer lines.

Joshua Dam
7,148 PointsGot it to work! Thank you for the answers. At first, I was converting the string into a list outside of the function. Moved it into the function and it passed. I can definitely make this simpler/smaller, just wanted to get it working period.
def disemvowel(word):
my_word = list(word)
for letters in my_word:
try:
my_word.remove('a')
except ValueError:
pass
try:
my_word.remove('e')
except ValueError:
pass
try:
my_word.remove('i')
except ValueError:
pass
try:
my_word.remove('o')
except ValueError:
pass
try:
my_word.remove('u')
except ValueError:
pass
try:
my_word.remove('A')
except ValueError:
pass
try:
my_word.remove('E')
except ValueError:
pass
try:
my_word.remove('I')
except ValueError:
pass
try:
my_word.remove('O')
except ValueError:
pass
try:
my_word.remove('U')
except ValueError:
pass
final_word = ''.join(my_word)
return final_word
disemvowel('Alligator')