Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial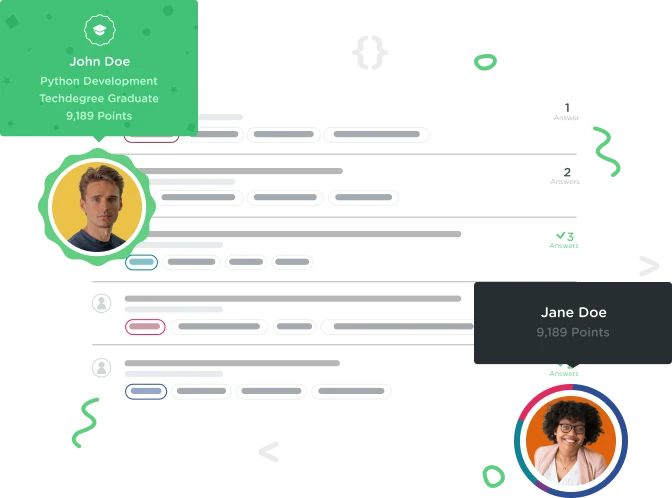
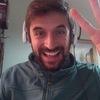
Francesco Cabiddu
2,297 Pointsdisemvowel challenge
Hi everyone! Do you know why my code doesn't pass the challenge? Thank you very much!
def disemvowel(word):
vowels = ['a', 'e' , 'i' , 'o' , 'u']
word = word.lower()
word = list(word)
for letter in word:
for vowel in vowels:
try:
word.remove(vowel)
except ValueError:
continue
word = "".join(word)
return word
5 Answers

Ryan S
27,276 PointsHi Francesco,
There are many different ways to solve this one, but your logic is on the right track so I'll try to offer suggestions that work with what you've already written.
The first thing is that by immediately using .lower()
on word
, you have overwritten any uppercase consonants that might be expected in the output. So no matter if you successfully remove all the vowels or not, it still may not pass the challenge. One way of avoiding this is to simply add the uppercase vowels to your vowels
list, and leave the case of the original word as it is.
The next thing is that it is usually not recommended to remove items from a list as you are iterating through it. This will cause some unwanted behaviour in the indexing of the list. For example, if you have a list with 5 items, and as you are iterating you remove the item at the current index (say, index 2), the for
loop will know to go to index 3 next, but the rest of the list will have shifted because of the removed item. So you will end up skipping some items.
A way around this is to make a copy of the list and work with that, but still iterate through the original string. Then assign it back to word
when you are done.
def disemvowel(word):
vowels = ['a', 'e' , 'i' , 'o' , 'u', 'A', 'E', 'I', 'O', 'U'] # add uppercase vowels
word_list = list(word) # make list copy of string
for letter in word: # iterate through original string
for vowel in vowels:
try:
word_list.remove(vowel) # remove vowel from the copy
except ValueError:
continue
word = "".join(word_list) # assign joined word_list back to word
return word
Hopefully this clears things up..

Dean Vollebregt
24,583 PointsThe regex library can be used too, its super powerful
import re
def disemvowel(word):
word = re.sub(r'[AEIOU-]', '', word, flags=re.IGNORECASE)
print(word)
I dont know my code isnt all in the box
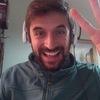
Francesco Cabiddu
2,297 PointsHI Ryan, Thank you very much for your clear answer! =) I realized that my code was also making the consonants lowercase, but I didn't figure it out how to fix it.
Francesco
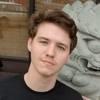
Austin Jones
8,305 PointsWhen I run this code in Pythonista for IOS, it works fine yet the challenge does not accept it.
def disemvowel(word):
my_word = list(word)
for letter in my_word:
for i in ["a", "A", "o", "O", "u", "U", "e", "E", "i", "I"]:
if letter == i:
my_word.remove(letter)
word = "".join(my_word)
return word

Jaco Vermuelen
Python Web Development Techdegree Student 167 PointsHere is a simpler version without a nested "for loop"
def disemvowel(word):
word_array = list(word)
for letter in word:
if letter.upper() == 'A' or letter.upper() == 'E' or letter.upper() == 'I' or letter.upper() == 'O' or letter.upper() == 'U':
word_array.remove(letter)
word_string = ''.join(word_array)
return word_string