Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial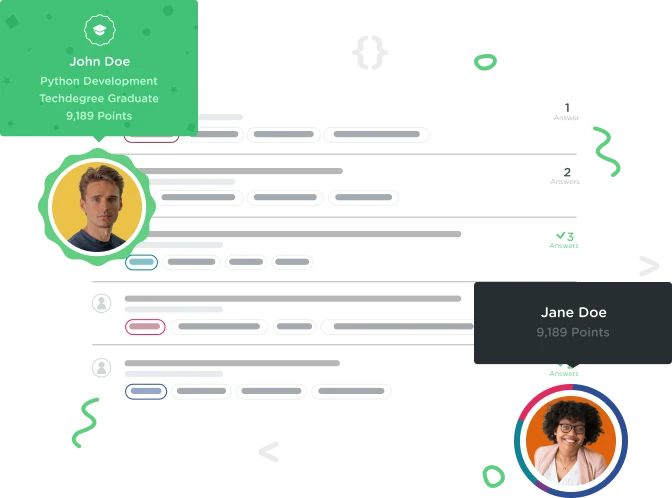

Stian Andreassen
523 PointsCustom Cell - button in cell updates multiple cells
I have a table view with custom cells. The cell consists of two buttons and a label. The buttons is a pluss button, and a minus button. The label is used for counting buttonpresses. When the pluss button is pressed, the label should increment by one, and decrease by one when the minus button is pressed. The problem is that the buttons updates multiple cells. How do I get just that one label in the corresponding cell to update?
Code: Custom Cell File:
import UIKit
class CustomTableViewCell: UITableViewCell {
var counter: Int = 0 {
didSet {
counterLabel.text = String(counter)
}
}
@IBAction func plussButton(_ sender: UIButton)
{
counter += 1
}
@IBAction func minusButton(_ sender: UIButton)
{
counter -= 1
}
@IBOutlet weak var nameLabel: UILabel!
@IBOutlet weak var counterLabel: UILabel!
override func awakeFromNib()
{
super.awakeFromNib()
// Initialization code
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
}
}
TableViewController:
import UIKit
class UnderMenyTableViewController: UITableViewController {
var nameArray = ["Cell 1", "Cell 2", "Cell 3", "Cell 4", "Cell 5" ]
override func viewDidLoad() {
super.viewDidLoad()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Table view data source
override func numberOfSections(in tableView: UITableView) -> Int {
// #warning Incomplete implementation, return the number of sections
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// #warning Incomplete implementation, return the number of rows
return nameArray.count
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "CustomCell", for: indexPath)
if let customCell = cell as? CustomTableViewCell
{
customCell.nameLabel.text = nameArray[indexPath.row]
customCell.counterLabel.text = String (customCell.counter)
}
return cell
}
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return 200
}
}
Appreciate any help.
P.S: Sorry for the bad formatting on code. This was as good as I could make it.
Taylor Smith
iOS Development Techdegree Graduate 14,153 PointsTaylor Smith
iOS Development Techdegree Graduate 14,153 PointsThere's a delegate method for tableviews called didSelectRowAt. type this in and it will auto fill like the other methods. This method allows you to manipulate the data for whatever cell is being clicked. Put whatever code you need in this method and it should work!