Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial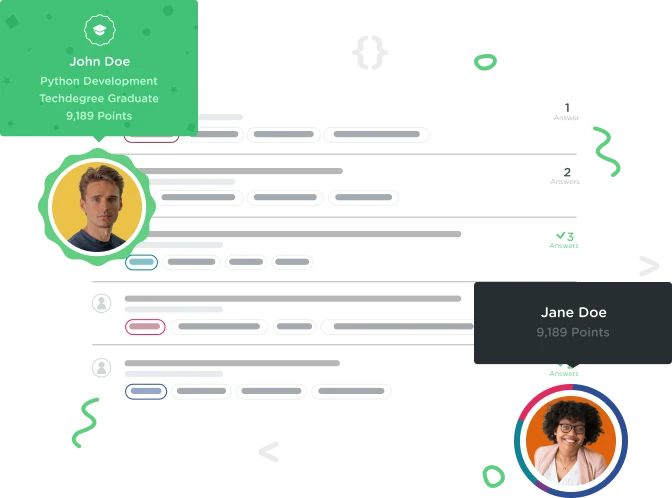

ALEKSANDAR TODOROVSKI
Courses Plus Student 8,508 PointsCreate a function named max that accepts two numbers as arguments (name the parameters whatever you would like). The fun
function max(10, 20) {
return larger;
}
1 Answer

Joshua Ordehi
4,648 Pointsfunction max(10, 20) { return larger; }
Hey Aleksandar,
Happy to see you're learning JavaScript.
It looks like you're passing two numbers as function parameters, however, function parameters are like variable names that take a value when you call the function later in the code. For example:
function max(num1, num2) {
}
It also looks like you're returning a variable named larger
, but the challenge wants you to find which of the two numbers passed to your max
function is the larger and then return it. For that, you need to use a conditional statement to compare one number to another to find which is larger, then return the one that's larger. For example:
function max(num1, num2) {
if (num1 > num2) {
return num1;
} else {
return num2
}
}
What this says basically is:
- if num1 is greater than num2
- then return num1
- else return num2
To unpack that, what I'm doing is comparing if num1 is bigger than num2, if it is, we return num1, if it's not, then num2 is larger so we return that.
Hope that helps, and good luck in your journey!