Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial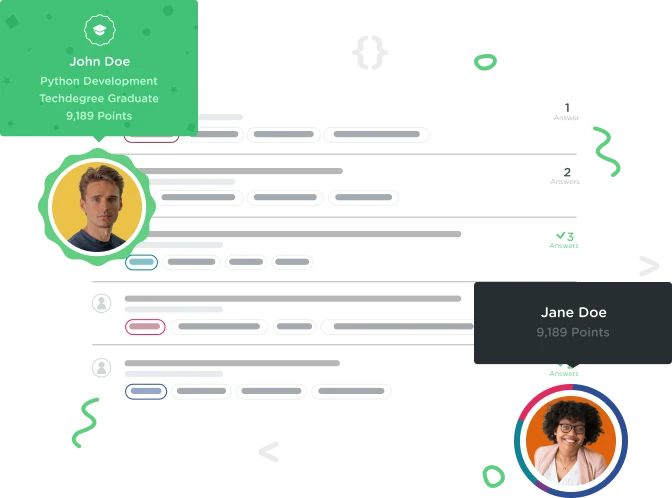

samt
6,039 PointsCould this be better?
Here is my code for the disemvowel code challenge. It works but it looks a little complex to me. Can anyone help me tidy it up or simplify it?
Thanks
Sam
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
word = list(word)
for each in vowels:
try:
while 1:
word.remove(each)
except ValueError:
continue
word = ''.join(word)
return word
4 Answers
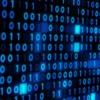
Alexander Davison
65,469 PointsAn even shorter version of james south's code:
return ''.join([x for x in word if x.lower() not in ['a', 'e', 'i', 'o', 'u']])
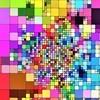
james south
Front End Web Development Techdegree Graduate 33,271 Pointshaha leave it in! i didn't try a word with more than one of a certain vowel and i should have. another approach that i used in making a one-liner for this one is to loop through the word and only take letters that aren't vowels and join those together. so instead of removing vowels, you would be adding (to a new string or list) consonants. most people loop through the word and remove vowels, but they modify the list they loop through which leads to the skipping i mentioned before. the solution here is to make a copy, loop through the copy and modify the original (or vice versa). lots of ways. just for fun here is my one-liner using a list comprehension:
return ''.join([x for x in word if x not in ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']])
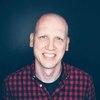
bryonlarrance
16,414 PointsNice!!
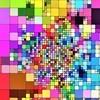
james south
Front End Web Development Techdegree Graduate 33,271 Pointsif you're a beginner i would say this is fine. you have avoided something that trips a lot of people up on this one, which is modifying a list while looping through it, which results in skipped elements. so your code works on a word with consecutive vowels like beauty. one thing you could remove that isn't doing anything is the while 1 statement.

samt
6,039 PointsHey James,
Thanks for the quick reply, without the while 1, only one instance of a given vowel was removed...that was really the bit that I thought was messy. Any ideas ?
Thanks
Sam

samt
6,039 PointsThanks Guys