Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial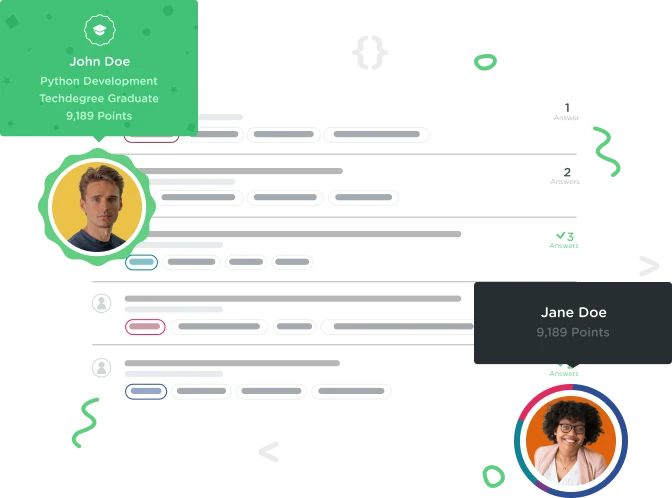
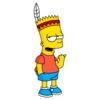
Will Hunting
13,726 PointsCould anyone kindly explain how to complete this task? I really don't understand what this question is asking of me...
Would be kindly appreciated.
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
ConferenceRegistrationAssistant test = new ConferenceRegistrationAssistant("lastName")
if(lastName.ch
/*lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
return lineNumber;
}
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
2 Answers

Jared Swain
3,153 PointsI had a really hard time with this challenge as well so don't feel discouraged. Also don't forget you can browse the community discussions with keywords to try and solve your problem. Saved me more then once.
So to answer your question, this challenge wants you to sort the patched in lastName into either line #1 or line #2 depending on the FIRST CHARACTER. Sounds easy but its challenging you to think in code which is still very new to us. So I'm gonna beat around the bush here a little so that hopefully you get it before I just give you the answer.
First things first we need to be able to access the first char of the String lastName to check IF its <= 'm' or >= 'n' to determine which line # that person should be in. To do this we need to check to see if there is a method in the String class that allows us to do that. https://docs.oracle.com/javase/7/docs/api/java/lang/String.html is a great link to check. Look in methods and find one that matches what we are trying to do.
Now that we have our method we need to set our parameters for what line lastName needs to be moved in. The example tab is showing you that chars like integers have a default weight or value. Example: A is < B and C is > then A. So to figure out where our lastName is going we should establish boundaries. Example: if lastName's first char is equal to or less then ''Z' and lastName's first char is equal or greater then 'N'.
Last but not least the action of returning either line 1 or line 2 based off the result of your if statement.
Now I walked through all this instead of just giving the answer because beyond this challenge Craig really ramps up his challenges and doesn't just give you the answer, he expects you to work for them. Hope this helps! Really recommend understanding this before moving on and trying to figure it out before just copying and pasting my code:) Also this is a very simple way to solve it you could go deeper if you wanted to but I feel its unnecessary.
int lineNumber = 1;
if(lastName.charAt(0) >= 'N' && lastName.charAt(0) <= 'Z'){
lineNumber++;
}
return lineNumber;
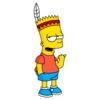
Will Hunting
13,726 PointsThanks Jared, appreciate your help on this one as I found myself trying to solve the above in my sleep.
Will refer to the docs on that site you have just recommended going fourth.
Samuel Essim
Front End Web Development Techdegree Student 15,963 PointsSamuel Essim
Front End Web Development Techdegree Student 15,963 PointsHi jared thanks it helped me too...but understanding these concepts is really a problem...i read all what you said but am not still getting it.