Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial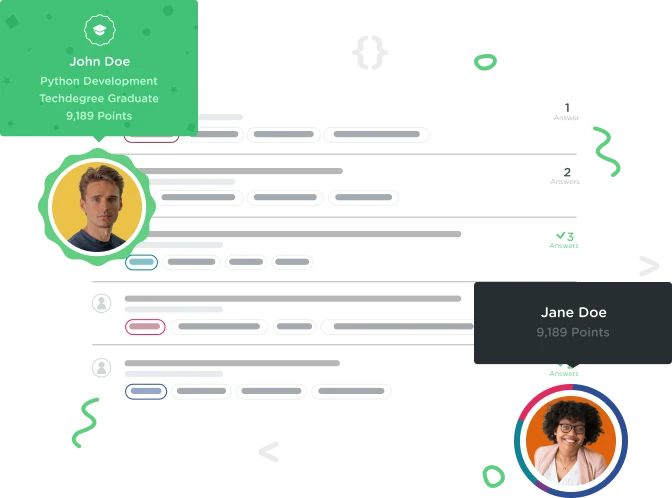

Colin Warn
6,358 PointsConstraints in Code, What Am I Missing?
Trying to add constraints into my code for the Intro to Autolayout challenge. Using the .height, .width, .centerX and .centerY attributes, yet it's saying I'm putting in the wrong attributes. Any idea what's wrong?
Code: let height = NSLayoutConstraint(item: sampleView, attribute: .height, relatedBy: .equal, toItem: self, attribute: .height, multiplier: 1.0, constant: 50.0)
let width = NSLayoutConstraint(item: sampleView, attribute: .width, relatedBy: .equal, toItem: self, attribute: .width, multiplier: 1.0, constant: 50)
let verticalConstraint = NSLayoutConstraint(item: sampleView, attribute: .centerX, relatedBy: .equal, toItem: view, attribute: .centerX, multiplier: 1.0, constant: 1.0)
let horizontalContraint = NSLayoutConstraint(item: sampleView, attribute: .centerY, relatedBy: .equal, toItem: view, attribute: .centerX, multiplier: 1.0, constant: 1.0)
// Add constraints below
view.addConstraints([height,
width,
verticalConstraint,
horizontalContraint])
1 Answer

Christian Mangeng
15,970 PointsHi Colin,
that's how I did it:
let height = NSLayoutConstraint(item: sampleView, attribute: .height, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 50.0)
let width = NSLayoutConstraint(item: sampleView, attribute: .width, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 50.0)
let centerHor = NSLayoutConstraint(item: sampleView, attribute: .centerX, relatedBy: .equal, toItem: view, attribute: .centerX, multiplier: 1.0, constant: 0.0)
let centerVer = NSLayoutConstraint(item: sampleView, attribute: .centerY, relatedBy: .equal, toItem: view, attribute: .centerY, multiplier: 1.0, constant: 0.0)
Note that width and height do not refer to any other item, so put nil and .notAnAttribute for toItem and the second attribute argument, respectively. Also, make sure to set the constant in width to 50.0, not the int value 50. It expects a float value input.