Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial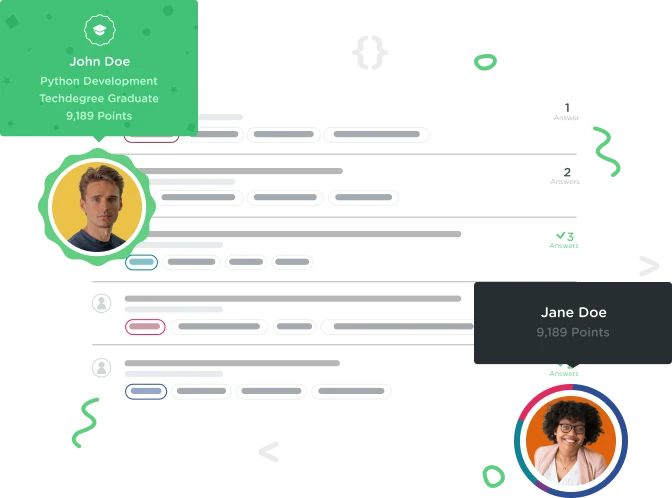

anna saraswati
Courses Plus Student 30 Pointsconfused with 'return' in Swift
hi all,
i am confused with 'return' in swift. I understand if it is used to return the value in a function, if it is used something like this i understand
func double (value: int) -> Int {
return value * 2
}
but I often see just 'return' is used , i mean something like in guard statement in optional binding like this
guard let value = value else (
print ("nothing")
return
}
so what the purpose of just 'return' in the guard statement like this ? actually i often see not only in guard statement of optional binding. i always find this problem when writing code, when i want to use optional string from a dictionary
let info = ["name":"sarah","hometown":"sydney"]
class UserInfo {
func getTheName () -> String {
guard let name = info["name"] else {return}
return name
}
}
xcode error : non-void function should return a value (even though i have written 'return name')
even though i have written 'return name', but xcode still consider that i have not returned a value. is it because of 'return' in guard statement ?
so could you please tell me the purpose of 'return' in swift ? it is confusing for me
Thanks in Advance :)
2 Answers
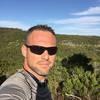
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsIn the situation of the name not being present you would want to throw an error and then handle the error. The function is expected to return a String but if name is not present it will not.
You could always do this to satisfy it:
func getTheName () throws -> String {
guard let name = info["name"] else {return "No name"}
return name
}
Yes you are right you are returning a value but you can also return nothing and just break out of the function.
Place this into a playground:
var sum = 0
func method(){
let num = 0
if num == 1 {
print("Equal")
} else {
print("Not Equal")
return //stops further execution of method
}
//The 2 lines of code below will not be executed since num is not equal to 1
sum = sum + num
print(sum)
}
method()
Now change the value of num to 1 and watch it finish executing.
Or as you stated you can return a value as well:
func someNumber() -> Int {
return 1 + 2
}
let value = someNumber()
print(value)
Also here is how you can format your code for the forum.
```Swift
///Code goes here
``` /// and then 3 more back ticks below the code

anna saraswati
Courses Plus Student 30 PointsThanks Brandon...
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsBrandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsUpdated answer