Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial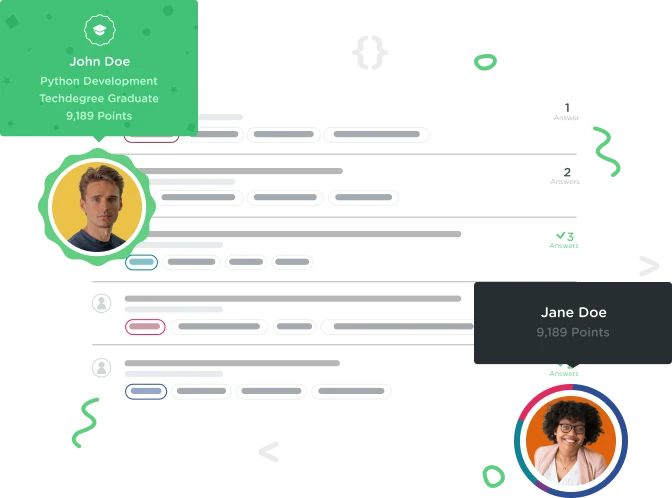

Itsa Snake
3,852 PointsConcatenating - correct use of converting int to str?
Hello,
I am doing this task and have figured out how to pass it.
The goal is to strip out all the text from a list, then add all the figures together, then combine the two.
While trying to code it, I kept getting this error on line 10: TypeError: can only concatenate str (not "int") to str
My fix was therefore to convert the return_num (int or float) into a str before calling combining it.
I'd be interested in how a pro would code the below. I feel like i've done an amateur workaround.
def combiner(the_list):
return_text = ""
return_num = 0
for item in the_list:
if isinstance(item, (int, float)):
return_num = return_num + item
elif isinstance(item, str):
return_text = return_text + item
return return_text+str(return_num)
my_list = ["silly", "bun", 1, 2, 3]
print(combiner(my_list))
2 Answers

ygh5254e69hy5h545uj56592yh5j94595682hy95
7,934 Points- I would not say that I'm a "PRO", but this is how I would solve this challenge
def combiner(listOfWordsAndNumber):
return_num = [itemInList for itemInList in listOfWordsAndNumber if isinstance(itemInList, (int, float))]
return_text = [itemInList for itemInList in listOfWordsAndNumber if isinstance(itemInList, (str))]
return ''.join(return_text) + str(sum(return_num))
my_list = ["silly", "bun", 1, 2, 3]
print(combiner(my_list))

ygh5254e69hy5h545uj56592yh5j94595682hy95
7,934 Points# It works fine when I use it locally. I guess TreeHouse wants you to use isinstance(each, (int, float)), like this
def combiner(arg):
word = ""
num = 0
for each in arg:
if isinstance (each, str):
word += each
elif isinstance (each, (int, float)):
num += each
output = word + str(num)
return output
Sergey Arestov
7,601 PointsSergey Arestov
7,601 PointsAnd this code is the answer. But can you please tell me what's the difference with mine (that wasn't correct ("Didn't get the expected output")):