Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial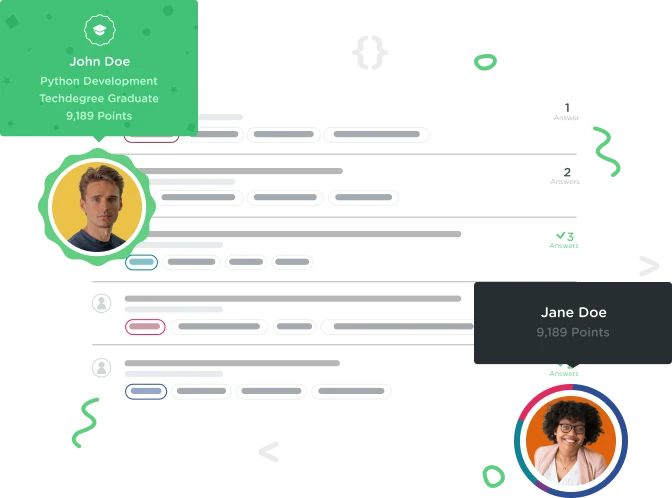

Neymat Kakar
iOS Development Techdegree Student 786 Pointscollections and control flow
Now that we have the while loop set up, it's time to compute the sum! Using the value of counter as an index value, retrieve each value from the array and add it to the value of sum.
For example: sum = sum + newValue. Or you could use the compound addition operator sum += newValue where newValue is the value retrieved from the array.
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
counter = numbers[counter]
sum = sum + counter
counter += 1
}
1 Answer

Jorge Solana
6,064 PointsHey Neymat!
You need to read and understand what your code is doing:
while counter < numbers.count {
This means you're going to use counter as index to measure how many times you repeat an action. In this particular case, it is going to repeat from 0 to 6 since there is 7 members in the numbers array.
counter = numbers[counter]
This is where everything explodes. You are telling counter to save the value of the counter position in the numbers array. First time in execution, that number is 2, so counter is going to switch from 0 to 2.
sum = sum + counter
counter += 1
Variable sum in this case is going to get the right value since you are filling counter with the value of the array, so this might be ok this time since sum is going to be "0 + 2" that is ok for this first iteration. But let's continue reading so you understand. Now, we add 1 to counter, so now we have "counter equals 3"
Then we go back to the while condition: 3 is less than 7, so it's ok and we get into the while statements. Repeat yourself the operation we just did. Now counter is going to receive the counter position in the array, since we start from 0, it's really the 4th position, so "counter = 16". Variable sum is now going to be "2+16", so you already jumped 2 values from the array. Are you getting the picture? Next thing, counter is adding 1 to that 16, so the next condition will ask if "17 < 7", and since it is false, your code will end, and sum will only got 2 values from the array instead of every single one of them.
Give it another try having this things in mind, and come back with the results. Keep up the hard work!
Neymat Kakar
iOS Development Techdegree Student 786 PointsNeymat Kakar
iOS Development Techdegree Student 786 Pointsbelow is the code: let numbers = [2,8,1,16,4,3,9] var sum = 0 var counter = 0
// Enter your code below