Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial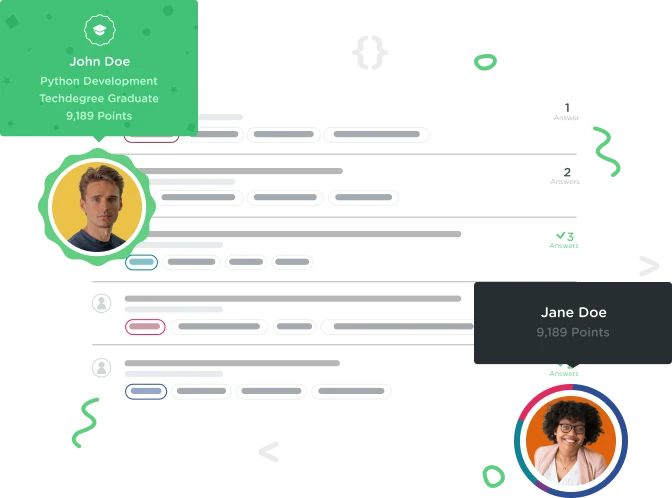

James Huang
6,368 PointsCode takes too long to run! How do i fix this?
Do i put all of the code under one do while loop?
/* So the age old knock knock joke goes like this:
Person A: Knock Knock.
Person B: Who's there?
Person A: Banana
Person B: Banana who?
...This repeats until Person A answers Orange
Person A: Orange
Person B: Orange who?
Person A: Orange you glad I didn't say Banana again?
*/
//Here is the prompting code
String who;
do {
console.printf("Knock Knock.\n");
who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
} while(who.equalsIgnoreCase("Banana"));
do {
who = console.readLine("Banana who?");
console.printf("%s who?\n", who);
console.printf("%s you glad I didn't say Banana again?", who);
} while(who.equalsIgnoreCase("Orange"));
2 Answers

Ken Alger
Treehouse TeacherJames;
I posted links to this information in your previous post on this topic, but let's have a go at it here:
Task 1
Read the comments and code below. We want to move that prompting code into a do while loop. Wrap the code into a do while and check in the condition to see if who equals "banana" so the loop continues. Remember to move your who declaration outside the do block.
Here is the code and comments we are given:
/* So the age old knock knock joke goes like this:
Person A: Knock Knock.
Person B: Who's there?
Person A: Banana
Person B: Banana who?
...This repeats until Person A answers Orange
Person A: Orange
Person B: Orange who?
Person A: Orange you glad I didn't say Banana again?
*/
//Here is the prompting code
console.printf("Knock Knock.\n");
String who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
For task 1 we need to create the do...while loop and meet the task requirements. Let's look at this with some basic problem solving methods (Prepare, Plan, Perform, and Perfect). We'll likely leave the Perfect stage until later. Prepare
We have watched the video lessons, and have the tools we need, we have the same output for which we are aiming in the code comments.
Plan
Here is what we are asked to do broken down into steps:
a. Move the prompting code into a do... while loop.
b. Check to see if the variable who is equal to banana.
c. They gave us a hint/tip of moving the who declaration outside the do block.
Perform
Our code would then need to look something like:
String who;
do {
console.printf("Knock Knock.\n");
who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
} while(who.equalsIgnoreCase("banana"));
Does that make any sense?
Task 2
You need to do a console.printf
line that matches the punchline code provided. This would be outside of the do... while block, so after all of the other code written. You need to use the string formatter in the answer as well.
So it would look like:
console.printf("%s you glad I didn't say Banana again?", who);
Ken

Ken Alger
Treehouse TeacherJames;
As long as you put the code outside the do...while
loop it will print out as long as you don't input banana
. That's the point of the loop. So, let's assume someone doesn't know about the code being a joke, and they put in the word "Muffin", the code would print out:
Muffin you glad I didn't say Banana again?
Functional, but not especially humorous.
For the sake of this challenge, assume that the only two possible inputs are "banana" and "orange". Keep putting in banana and you stay in the do...while
loop. Put in orange you're now out of the loop and the punchline is displayed.
Make any sense?
Ken
James Huang
6,368 PointsJames Huang
6,368 PointsIf you console.printf("%s you glad I didn't say Baana again?", who); Wouldn't the punchline be: Banana you glad I didn't say Banana again?