Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial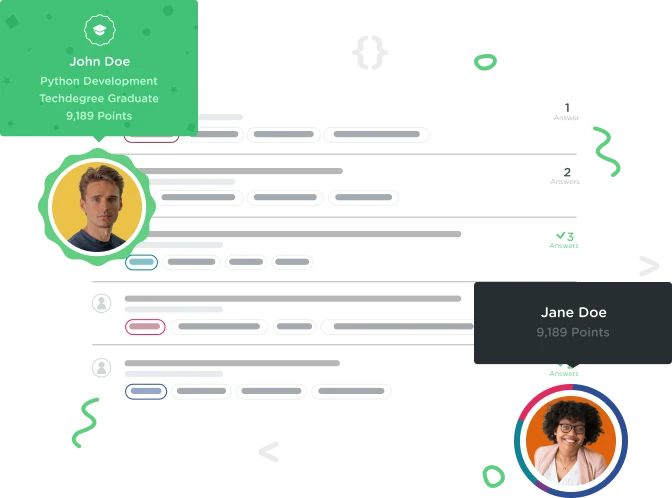

uday kiran
3,222 Pointscode is not working
please help
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod
def from_string(cls,x):
l=[]
for i in x:
if i=="dash":
l.append('_')
elif: i=="."
l.append('.')
return cls(l)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
5 Answers
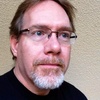
Chris Freeman
Treehouse Moderator 68,454 PointsYou have the right approach. Correct the following issues:
-
i
should be compared to "dot", not a literal period (".") - The colon for the
elif
statement needs to be a the end of the line - You need to spit
x
into words usingx.split()
. Otherwise, the for loop will evaluate each character of the string.
Post back if you need more help. Good luck!!

uday kiran
3,222 Pointsi got it can you help me with this problem Frustration
class Liar(list):
def __init__(self,*args,**kwargs):
super.__init__()
def __len__(self):
return len(self)-2
[MOD: added ```python formatting -cf]
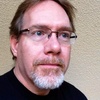
Chris Freeman
Treehouse Moderator 68,454 PointsSince you are overriding the __len__
method you need to use super().__len__()
to get the length of this instance.
Also, correct
- the "lie" should use addition, since you can't return a negative length.
- the
super()
in__init__
is missing parens
Good Luck!!

uday kiran
3,222 Pointsits still not working
class Liar(list):
def __init__(self,*args,**kwargs):
super().__init__()
def __len__(self):
return super().__init__()+2
[MOD: added ```python formatting -cf]
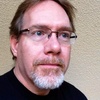
Chris Freeman
Treehouse Moderator 68,454 PointsYou need super().__len__
not __init__

uday kiran
3,222 PointsThis code is working but i have few questions
class Liar(list):
def __init__(self,*args,**kwargs):
super().__init__()
def __len__(self):
return super().__len__() + 2
1) How to use the above modified len method because when i use the below code, its not modifying the result
from Frustration import Liar
x=Liar()
x=['y','x']
print (len(x))
2) can the same job be done with out overriding init(), if not then why??
[MOD: added ```python formatting -cf]
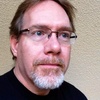
Chris Freeman
Treehouse Moderator 68,454 PointsThe code x=['y','x']
reassigns x
to be a regular list.
There are two ways to use Liar
:
# instantiate, then extend (or append):
from Frustration import Liar
x=Liar()
x.extend(["y", "x", "w"])
print(len(x))
# shows 5
# improve init to accept initial values
class Liar(list):
def __init__(self,*args,**kwargs):
super().__init__(*args,**kwargs) # pass args along
def __len__(self):
return super().__len__() + 2
x=Liar(["y", "x"])
#x=['y','x'] # skipped
print (len(x))
# shows 4

uday kiran
3,222 PointsThank you that helps a lot