Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial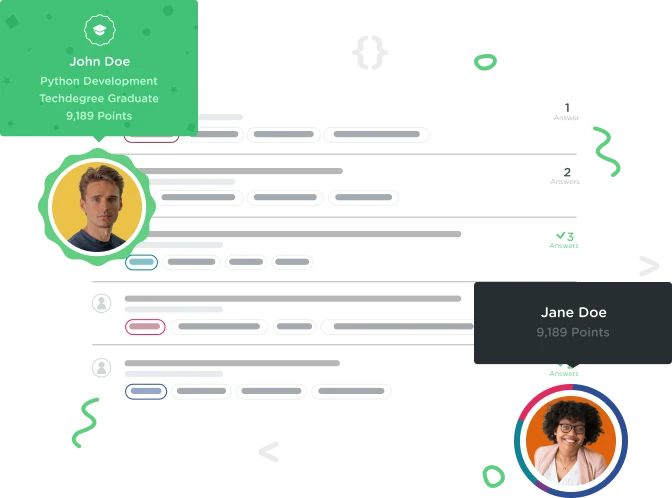

Hussein Amr
2,461 Pointscode challenge
Am I close to the answer?
def combiner(lista):
lista = ["7amada", 81 , "rollers", 5.6]
for i in lista:
if isinstance(i , (str, int , float)):
print(i.join(str, int, float))
1 Answer
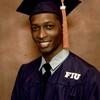
Dane Parchment
Treehouse Moderator 11,077 PointsNo, unfortunately you are not close to solving this problem, so to alleviate that, why don't we solve this together?
The instructions ask us to create a function called combiner that will take a list as a parameter:
def combiner(the_list):
pass
Then we are told how to solve the problem: Namely we need to iterate through each item within the list and depending on it's type we have to either append it to a string or add it to a number. So let's begin setting that up:
def combiner(the_list):
sum_of = 0;
str_of = ""
So above what we do is create two important variables: sum_of
and str_of
. These two variables will store the sum of all of the numbers within the list and the combined strings of the list respectively. Now we need to iterate through the list and access each item, we can easily do that with a for in
loop.
def combiner(l):
sum_of = 0;
str_of = ""
for item in l:
Now that we are iterating through each list item referencing each item as item
we can now check if said item is of a number or string type!
def combiner(l):
sum_of = 0;
str_of = ""
for item in l:
if(isinstance(item, str)):
str_of += item
First we check and see if it is of type str
(or string) and if so we add it to our empty string from earlier, then moving to the next item! Now we can check if the item is of a number type: int, long, float, complex
and if so add it to our sum_of variable.
def combiner(l):
sum_of = 0;
str_of = ""
for item in l:
if(isinstance(item, str)):
str_of += item
if(isinstance(item,(int, float, complex))):
sum_of += item
That's it! Now we can just return the combined string and sum together and return it!
def combiner(l):
sum_of = 0;
str_of = ""
for item in l:
if(isinstance(item, str)):
str_of += item
if(isinstance(item,(int, float, complex))):
sum_of += item
return "{}{}".format(str_of, sum_of)
There is of course a fatal flaw of this program but it will work regardless! (Hint: the fatal flaw has to do with the fact that this program will always assume that their are numbers in the list, what do you think it will do wrong if there are no numbers in the list?)
Hussein Amr
2,461 PointsHussein Amr
2,461 PointsThanks lad! well I don't know what it would do but we can use an exception to fix it I guess