Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial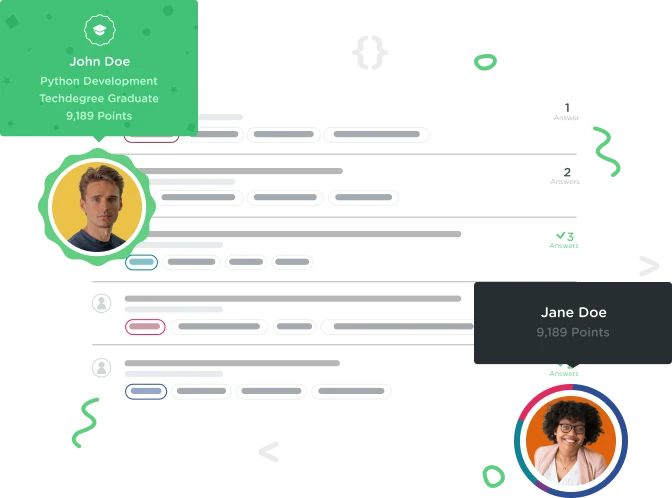
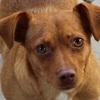
Michalis Efstathiou
Full Stack JavaScript Techdegree Student 8,605 PointsClosures - Still unsure why the counter resets
when you call the outer function which contains var counter = 0, why doesnt counter reset
1 Answer

Phillip Kazanjian
6,273 PointsOk, let’s break this down.
This is the makeBirdCounter function from the video:
function makeBirdCounter() {
var count = 0;
return function () {
count += 1;
return count + ‘ birds’;
}
}
makeBirdCounter is an example of an outer function. Let’s look at the line inside makeBirdCounter that says return. That function without a name is an inner function.
Remember: inner functions can access variables defined in outer functions.
When we call makeBirdCounter(), it’s going to return the inner function.
So, writing makeBirdCounter() will return this:
function() {
count += 1;
return count + ‘ birds’;
}
So, let’s call makeBirdCounter() and assign it to a variable called birdCounter:
var birdCounter = makeBirdCounter();
The birdCounter variable now points to the inner function that was on makeBirdCounter’s return line.
So, it’s like we directly assigned the inner function to the variable:
Var birdCounter = function() {
count += 1;
return count + ‘ birds’;
}
When we write birdCounter(), we are only calling the inner function.
Only this logic is ran each time we call birdCounter():
count += 1;
return count + ‘ birds’;
So, when we call birdCounter(), that line of code var count = 0
from the outer function is not read.
That’s why the count doesn’t reset.
Instead, our inner function increments the outer function's count variable.

Brian Kidd
UX Design Techdegree Graduate 36,188 PointsBeautifully explained. Thanks!

Akshay Alok
7,857 PointsGreat explanation!
So, when we call birdCounter(), that line of code var count = 0 from the outer function is not read. That’s why the count doesn’t reset. Instead, our inner function increments the outer function's count variable.
This really solved my doubt, thanks
odzer
20,483 Pointsodzer
20,483 PointsIt resets to 0 when the outer function is called, but in the video most of the time it is only the inner function being called. In the console, he calls the outer function and puts the result (which is the inner function) in a variable, and then calls that variable several times. So he is only calling the inner function.